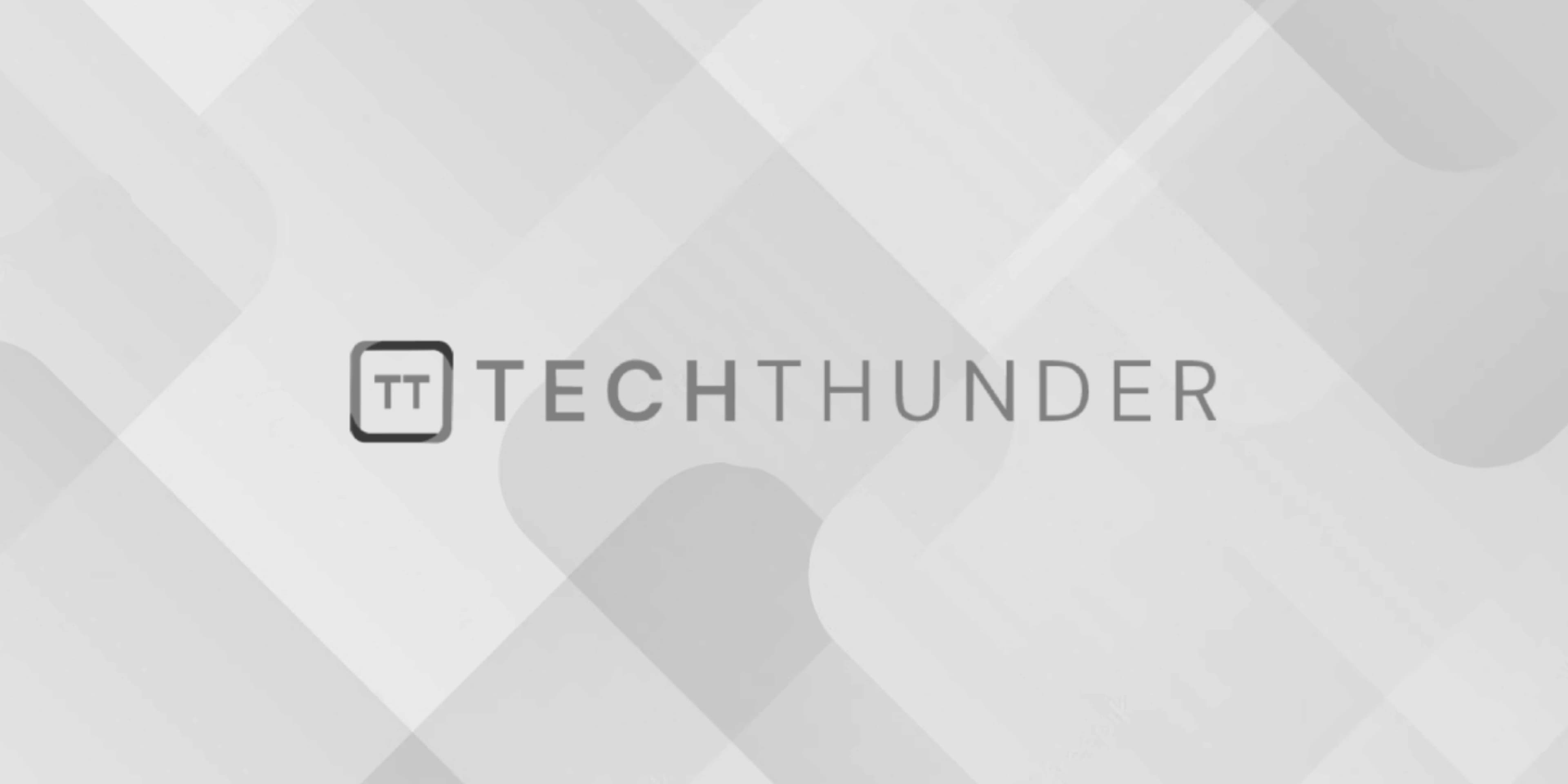
439 views
JavaScript Math
The JavaScript Math
object provides a set of built-in functions for performing mathematical operations. The Math
object is not a constructor, so you don’t need to use the new
keyword to create an instance of it. You can use the methods and properties of the Math
object directly, like this:
JavaScript
console.log(Math.PI); // Output: 3.141592653589793
console.log(Math.abs(-10)); // Output: 10
console.log(Math.ceil(3.14)); // Output: 4
console.log(Math.floor(3.14)); // Output: 3
console.log(Math.max(1, 2, 3)); // Output: 3
console.log(Math.min(1, 2, 3)); // Output: 1
console.log(Math.pow(2, 3)); // Output: 8
console.log(Math.random()); // Output: a random number between 0 and 1
console.log(Math.round(3.5)); // Output: 4
console.log(Math.sqrt(16)); // Output: 4
Here is a brief explanation of some of the most commonly used methods of the Math
object:
Math.PI
: Returns the value of pi (3.141592653589793).Math.abs(x)
: Returns the absolute value ofx
.Math.ceil(x)
: Returns the smallest integer greater than or equal tox
.Math.floor(x)
: Returns the largest integer less than or equal tox
.Math.max(x1, x2, ..., xn)
: Returns the largest of the specified values.Math.min(x1, x2, ..., xn)
: Returns the smallest of the specified values.Math.pow(x, y)
: Returnsx
raised to the power ofy
.Math.random()
: Returns a random number between 0 and 1 (exclusive).Math.round(x)
: Returns the value ofx
rounded to the nearest integer.Math.sqrt(x)
: Returns the square root ofx
.
There are many other methods and properties available on the Math
object. You can find more information on the Math
object in the JavaScript documentation.