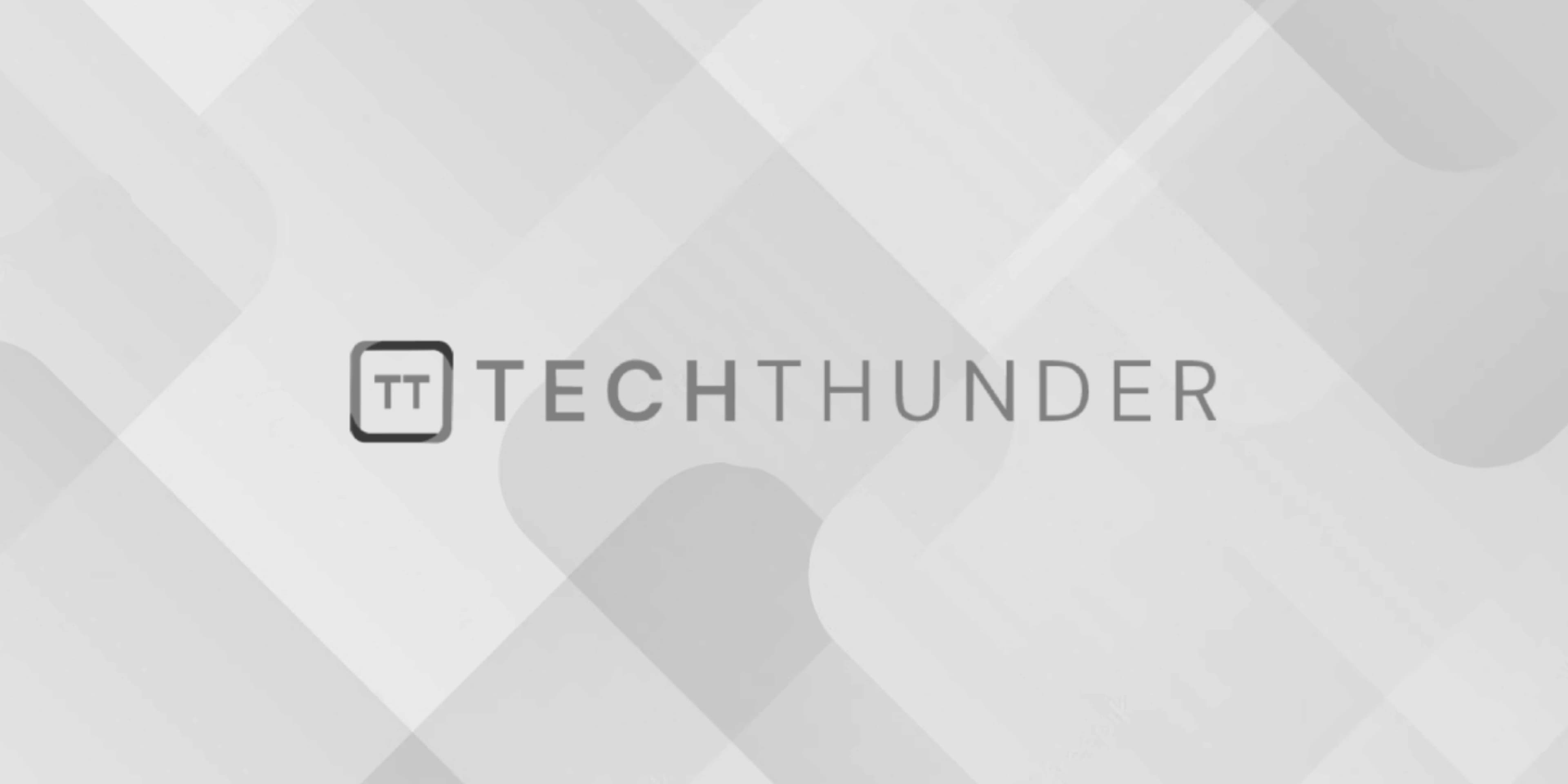
JavaScript Endswith() Function
JavaScript does not have a built-in endsWith()
function like startsWith()
for checking the end of a string. However, you can easily implement this functionality using the slice()
method or regular expressions.
- Using
slice()
method:
function endsWith(str, suffix) {
return str.slice(-suffix.length) === suffix;
}
console.log(endsWith('Hello, World!', 'World!')); // true
console.log(endsWith('Hello, World!', 'World')); // false
In this example, the endsWith()
function takes two parameters: str
(the input string) and suffix
(the string to check if it is at the end of str
). It uses the slice()
method to extract the substring from the end of str
with the same length as suffix
and compares it to suffix
. If they match, it returns true
; otherwise, it returns false
.
- Using regular expressions:
function endsWith(str, suffix) {
var regex = new RegExp(suffix + '$');
return regex.test(str);
}
console.log(endsWith('Hello, World!', 'World!')); // true
console.log(endsWith('Hello, World!', 'World')); // false
In this example, the endsWith()
function creates a regular expression pattern using suffix
concatenated with the $
symbol, which represents the end of the string. It then uses the test()
method to check if str
matches the regular expression pattern.
Both approaches achieve the same result of checking if a string ends with a specific substring. Choose the method that suits your needs and coding style.