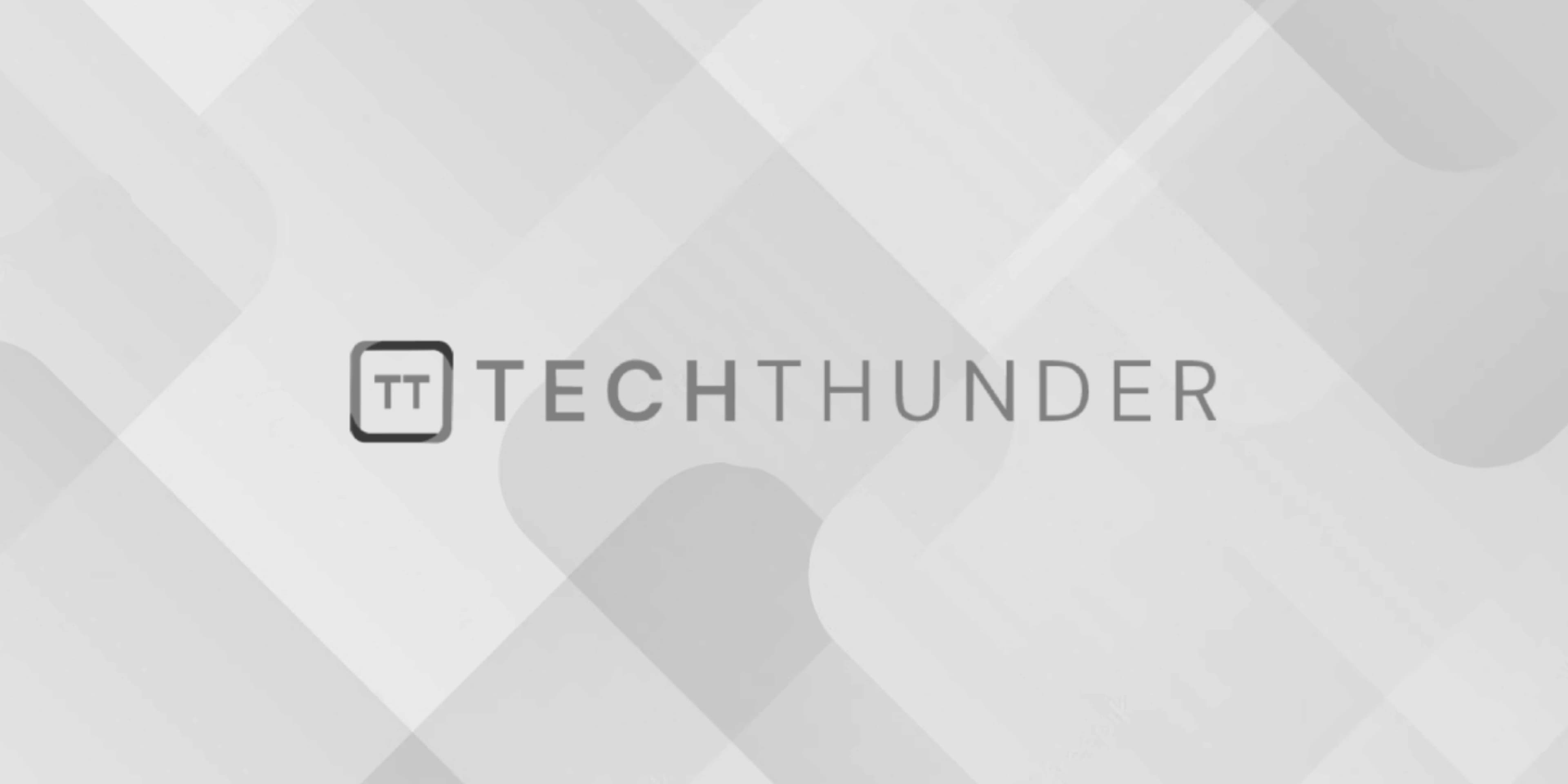
JavaScript setInterval() method
The setInterval()
method in JavaScript is used to repeatedly execute a function or a piece of code at a specified interval. It sets up a recurring timer that calls a function or evaluates an expression repeatedly, based on the specified time interval.
The syntax of the setInterval()
method is as follows:
setInterval(function, interval);
Here’s how it works:
- The
function
parameter is the function or code to be executed repeatedly. It can be a function reference or an anonymous function. - The
interval
parameter is the time interval between each execution of the function, specified in milliseconds. For example, if you set the interval to 1000, the function will be called every 1000 milliseconds (or 1 second).
Here’s an example that demonstrates the usage of the setInterval()
method:
function sayHello() {
console.log("Hello!");
}
// Call the sayHello function every 2 seconds
setInterval(sayHello, 2000);
In this example, the sayHello
function will be executed every 2 seconds. It will print “Hello!” to the console repeatedly until the setInterval()
timer is cleared or the page is refreshed.
To stop the recurring execution of the function, you can use the clearInterval()
method and pass in the timer ID returned by setInterval()
. For example:
var timerId = setInterval(sayHello, 2000);
// Stop the execution after 10 seconds
setTimeout(function() {
clearInterval(timerId);
}, 10000);
In this case, the clearInterval()
method is called after 10 seconds to stop the execution of the sayHello
function.