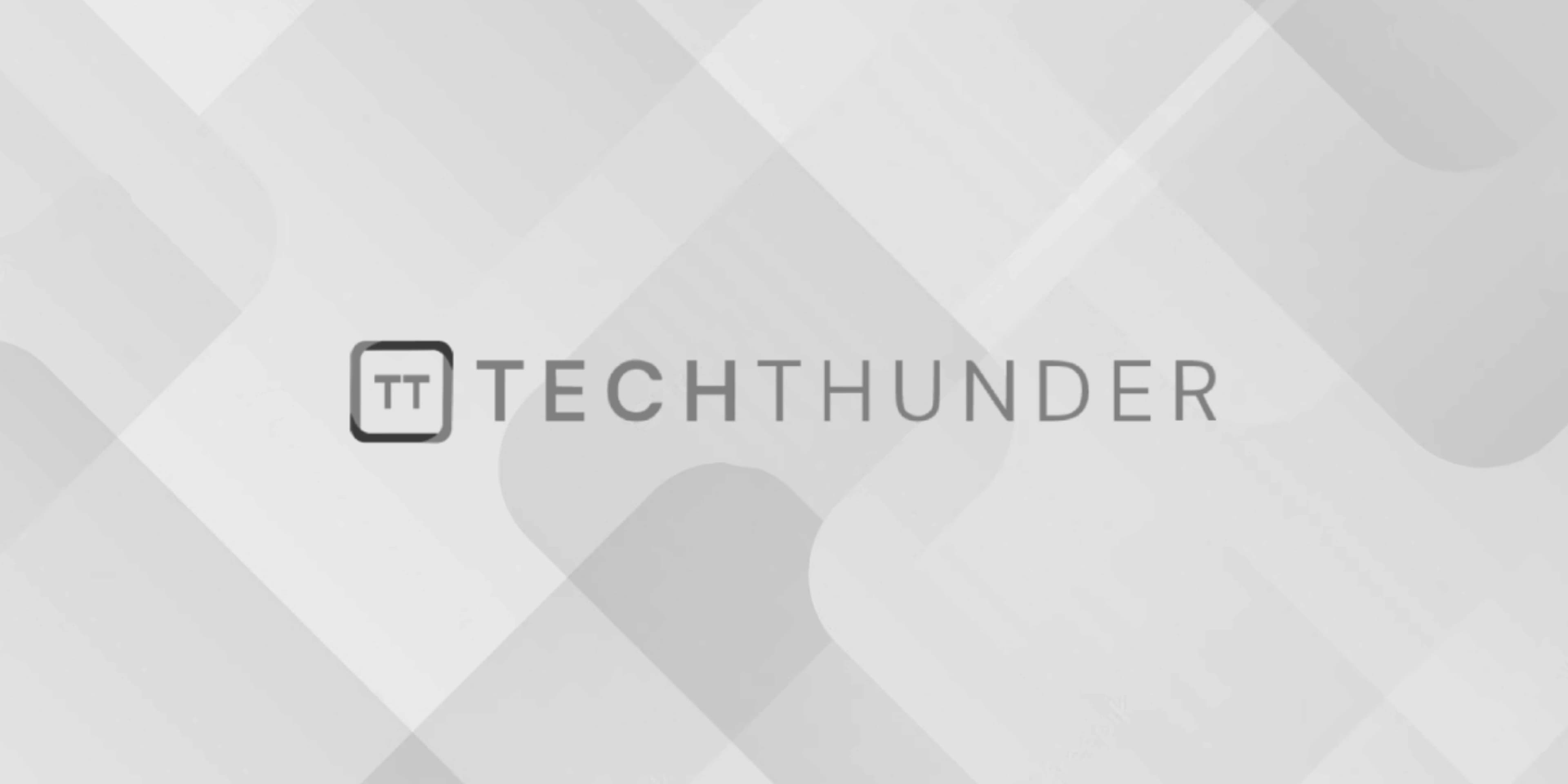
Confirm password validation
To perform password validation that includes a confirmation step in JavaScript, you can compare the values of two input fields, one for the password and the other for the confirm password. Here’s an example:
HTML:
<label for="password">Password:</label>
<input type="password" id="password" />
<label for="confirm-password">Confirm Password:</label>
<input type="password" id="confirm-password" />
<button onclick="validatePassword()">Submit</button>
JavaScript:
function validatePassword() {
const passwordInput = document.getElementById('password');
const confirmPasswordInput = document.getElementById('confirm-password');
const password = passwordInput.value;
const confirmPassword = confirmPasswordInput.value;
if (password !== confirmPassword) {
alert("Passwords do not match!");
return;
}
// Passwords match, perform further actions
// ...
}
In this example, the validatePassword()
function is triggered when the user clicks the “Submit” button. It retrieves the values entered in the password and confirm password input fields and compares them using an if
statement.
If the passwords do not match, an alert message is displayed to the user. Otherwise, you can perform further actions or validations as needed.
Note that this is a basic example, and you may want to add additional checks such as password complexity requirements or handling of empty input fields. Additionally, it’s recommended to provide visual feedback to the user indicating whether the passwords match in real-time, such as displaying a checkmark or error message next to the confirm password field as the user types.
Remember to add appropriate form validation and security measures when handling passwords in a production environment.