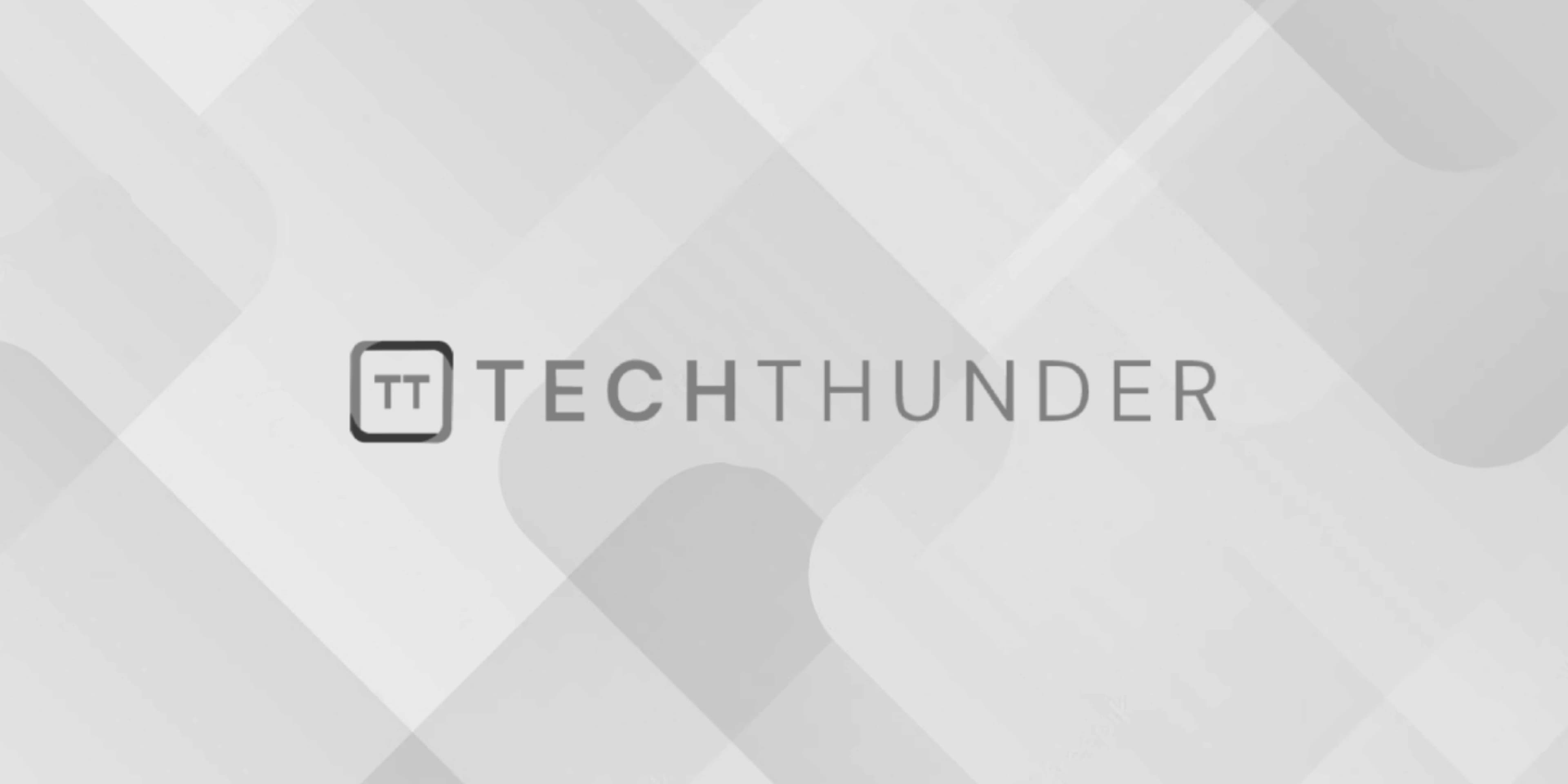
Lodash _.get() Method
The _.get()
method in Lodash is used to retrieve the value of a property from an object based on a provided property path. It allows you to safely access nested properties without encountering undefined
errors or throwing exceptions if any intermediate property is undefined.
Here’s the basic syntax for using _.get()
:
_.get(object, path, [defaultValue])
object
: The object from which to retrieve the value.path
: The property path as a string or an array representing the nested structure of properties.defaultValue
(optional): The value to return if the resolved value isundefined
.
Example usage:
const user = {
id: 1,
name: 'John',
address: {
street: '123 Main St',
city: 'New York',
country: 'USA'
}
};
const cityName = _.get(user, 'address.city');
console.log(cityName); // 'New York'
const zipcode = _.get(user, 'address.zipcode', 'N/A');
console.log(zipcode); // 'N/A'
In the example above, we have an object representing a user with nested properties. We use _.get()
to retrieve the value of the city
property nested within the address
property. The method returns 'New York'
, the value of the city
property.
In the second example, we attempt to retrieve the value of the non-existent zipcode
property using _.get()
. Since the property doesn’t exist, we provide a default value of 'N/A'
. The method returns the default value instead of undefined
.
The path
parameter can be provided as a dot-separated string or an array of property names to represent the nested structure. For example:
const value = _.get(object, 'nested.property.path');
// or
const value = _.get(object, ['nested', 'property', 'path']);
The _.get()
method can be especially useful when dealing with complex nested data structures or when you’re uncertain about the existence of certain properties. It allows you to safely access nested properties without writing complex and error-prone checks for each intermediate property.