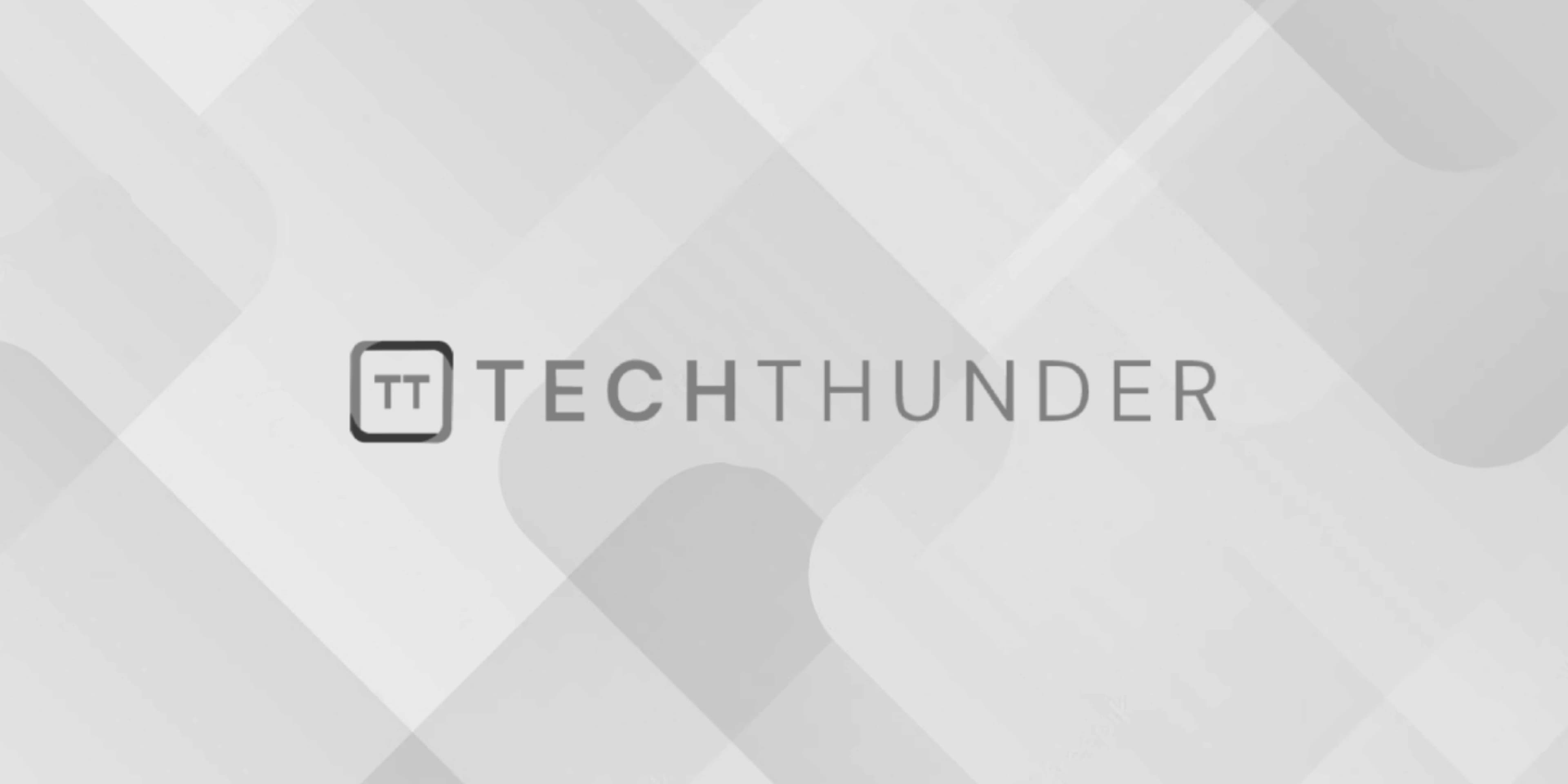
Create a JavaScript Countdown Timer
Sure! Here’s an example of how you can create a countdown timer in JavaScript:
HTML:
<div id="countdown"></div>
JavaScript:
function countdown() {
// Set the date and time to countdown to
var countdownDate = new Date('2023-12-31T23:59:59').getTime();
// Update the countdown every second
var countdownInterval = setInterval(function() {
// Get the current date and time
var now = new Date().getTime();
// Calculate the time remaining
var timeRemaining = countdownDate - now;
// Check if the countdown has ended
if (timeRemaining <= 0) {
clearInterval(countdownInterval);
document.getElementById('countdown').innerHTML = 'Countdown Ended!';
return;
}
// Calculate days, hours, minutes, and seconds
var days = Math.floor(timeRemaining / (1000 * 60 * 60 * 24));
var hours = Math.floor((timeRemaining % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
var minutes = Math.floor((timeRemaining % (1000 * 60 * 60)) / (1000 * 60));
var seconds = Math.floor((timeRemaining % (1000 * 60)) / 1000);
// Display the countdown in the element with id="countdown"
document.getElementById('countdown').innerHTML =
'Countdown: ' +
days + ' days ' +
hours + ' hours ' +
minutes + ' minutes ' +
seconds + ' seconds ';
}, 1000);
}
// Start the countdown
countdown();
In this example, we define a countdown()
function that sets the target date and time to countdown to and then updates the countdown every second. Inside the interval function, we calculate the remaining time in milliseconds and then convert it to days, hours, minutes, and seconds. Finally, we display the countdown in the HTML element with the ID “countdown”.
You can customize the target date and time by modifying the countdownDate
variable with your desired date and time.
Please note that this example provides a basic implementation of a countdown timer. You may want to enhance it further with additional formatting or styling based on your specific requirements.