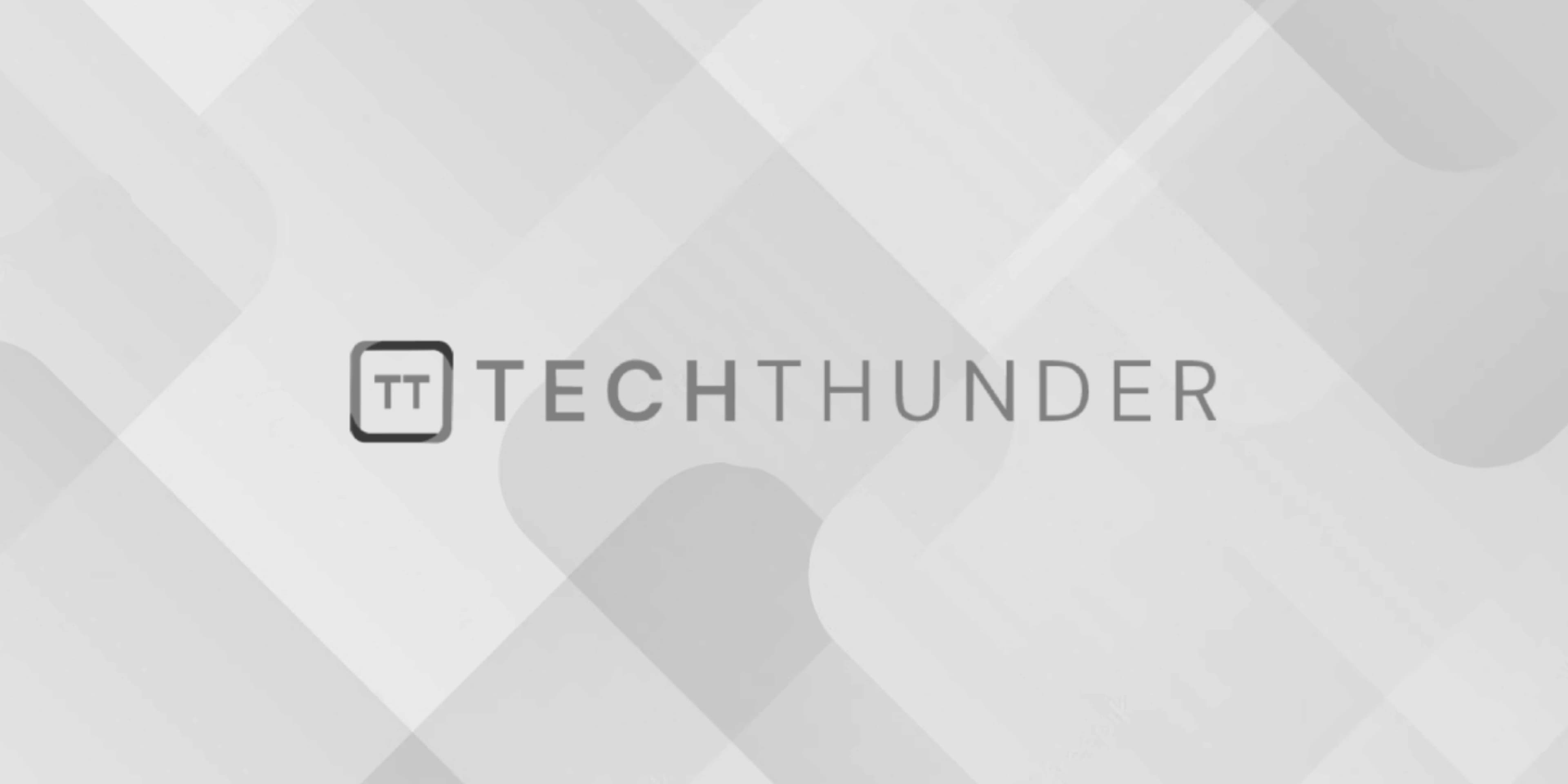
JavaScript Garbage
The JavaScript “garbage” term refers to unused or unreferenced objects in memory that are no longer needed by the program. JavaScript has a built-in garbage collector that automatically frees up memory by identifying and removing these unused objects.
The garbage collector in JavaScript works by using a technique called “automatic memory management.” It keeps track of objects and their references within the program. When an object is no longer reachable by any part of the program, it is considered garbage and can be safely removed from memory.
Here are a few scenarios where the garbage collector in JavaScript comes into play:
- Object Deletion: When you delete an object reference, either by setting it to
null
or using thedelete
operator, the garbage collector can detect that the object is no longer reachable and free up the memory associated with it.
var obj = { name: "John" };
obj = null; // The object { name: "John" } becomes garbage
- Scope Cleanup: When a function completes execution, the variables and objects within that function’s scope are no longer accessible. The garbage collector can identify and release the memory used by these objects.
function myFunction() {
var data = [1, 2, 3]; // data array is garbage after function execution
// ...
}
- Circular References: If two or more objects reference each other in a circular manner, but are not reachable from the rest of the program, the garbage collector can detect this situation and reclaim the memory occupied by those objects.
var obj1 = {};
var obj2 = {};
obj1.ref = obj2;
obj2.ref = obj1;
obj1 = null; // Both obj1 and obj2 become garbage due to circular reference
obj2 = null;
It’s important to note that the garbage collector operates automatically and transparently in JavaScript, freeing up memory without any explicit action required from the developer. This behavior helps simplify memory management in JavaScript applications, as developers don’t have to manually deallocate memory or explicitly invoke a garbage collection process.