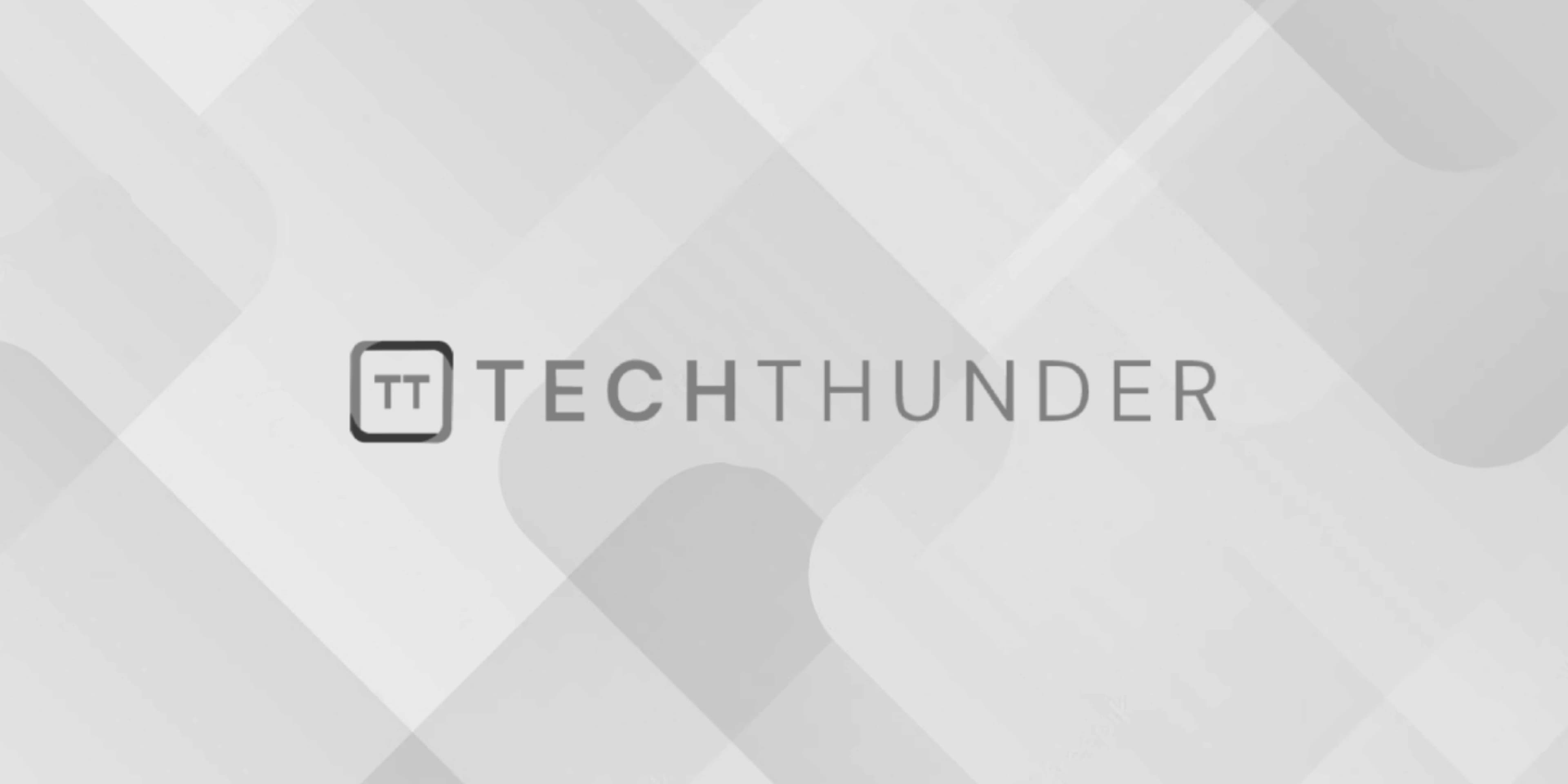
Lodash _.find() Method
The _.find()
method in Lodash is used to iterate over a collection (array or object) and return the first element that matches a given condition. It provides a convenient way to find elements based on a specific criteria without writing manual loops.
Here’s the basic syntax for using _.find()
:
_.find(collection, [predicate=_.identity], [fromIndex=0])
collection
: The collection to iterate over.predicate
(optional): The function or object used to match elements. If a function is provided, it should return true for the desired element. If an object is provided, it will be used for key-value matching.fromIndex
(optional): The index to start the search from.
const users = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Jane', age: 30 },
{ id: 3, name: 'Alice', age: 28 }
];
const user = _.find(users, { age: 30 });
console.log(user); // { id: 2, name: 'Jane', age: 30 }
In the example above, we have an array of user objects. We use _.find()
to search for the first user object with an age of 30. The method returns the first matching object, which is { id: 2, name: 'Jane', age: 30 }
. If no match is found, it returns undefined
.
You can also use a predicate function instead of an object for more complex matching. The predicate function takes each element as an argument and should return true
for the desired element.
const users = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Jane', age: 30 },
{ id: 3, name: 'Alice', age: 28 }
];
const user = _.find(users, (user) => user.age > 25);
console.log(user); // { id: 2, name: 'Jane', age: 30 }
In this example, we use a predicate function that checks if the user’s age is greater than 25. The _.find()
method returns the first user object that matches the condition.
Overall, the _.find()
method is a powerful tool provided by Lodash for finding elements in a collection based on a specific condition, simplifying the process of searching and filtering data.