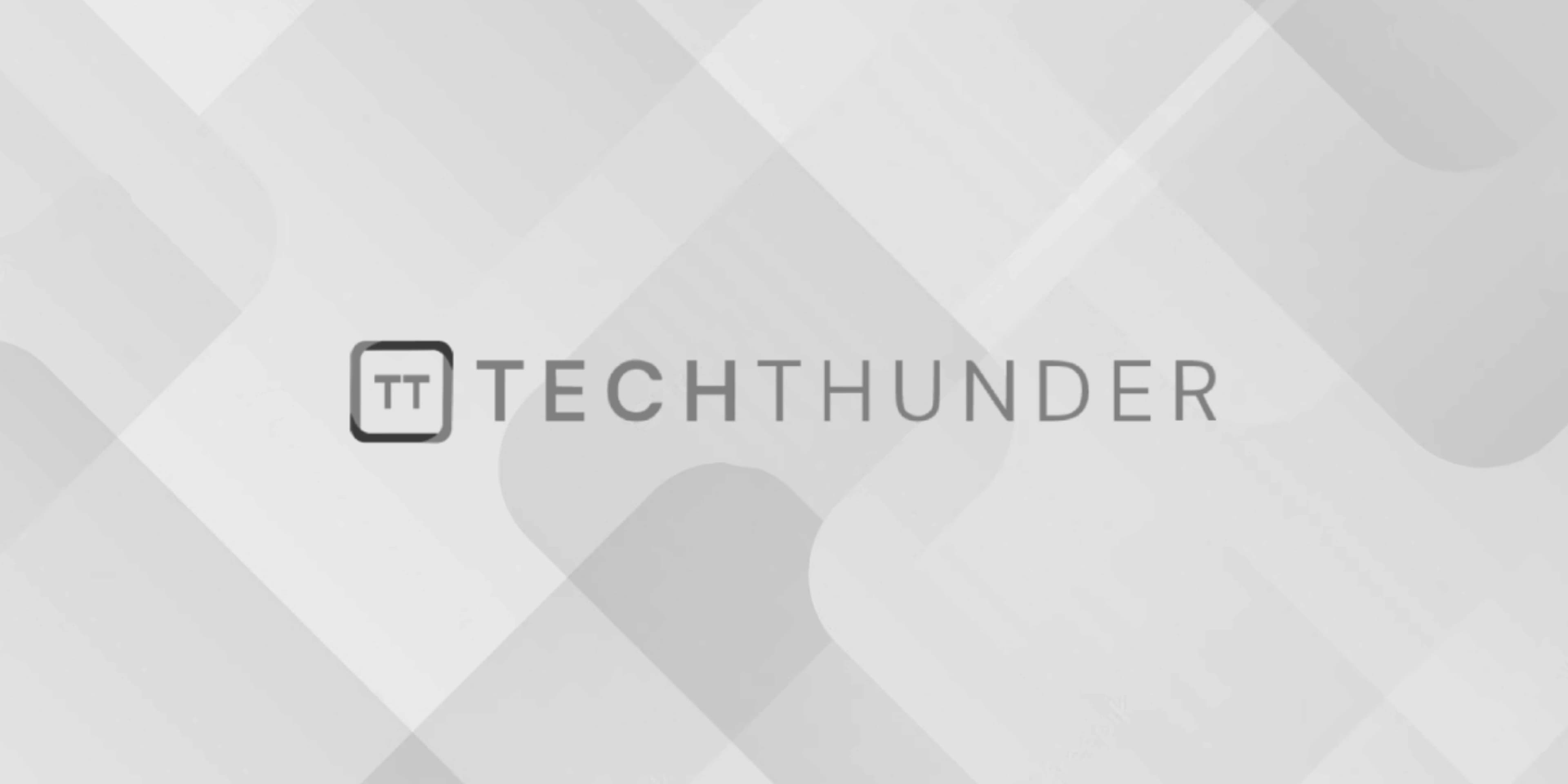
JavaScript Prototype
In JavaScript, the prototype is an object that is associated with every function and object. It is a mechanism that allows objects to inherit properties and methods from other objects.
Every JavaScript object has a prototype property, which references the prototype object associated with it. The prototype object contains properties and methods that are shared among all instances created from the same constructor function or derived from the same object.
The prototype object is used primarily for inheritance in JavaScript. When a property or method is accessed on an object, JavaScript first looks for it directly on the object. If it’s not found, it then checks the object’s prototype, and if still not found, it continues to traverse up the prototype chain until it reaches the top-level Object.prototype
.
To set the prototype of an object or a constructor function, you can use the prototype
property. Here’s an example:
function Person(name) {
this.name = name;
}
Person.prototype.sayHello = function() {
console.log(`Hello, my name is ${this.name}`);
};
const john = new Person("John");
john.sayHello(); // Output: "Hello, my name is John"
In the above example, the Person
constructor function has a prototype object, which is shared among all instances created using new Person()
. The sayHello
method is defined on the prototype, so every Person
object can access it.
Using prototypes, you can add properties and methods to all objects of a particular type without duplicating them for each instance. This saves memory and promotes code reusability.
Prototype-based inheritance is a key feature of JavaScript and allows for powerful and flexible object-oriented programming. It provides a way to define a blueprint for objects and allows objects to inherit properties and methods from their prototypes.