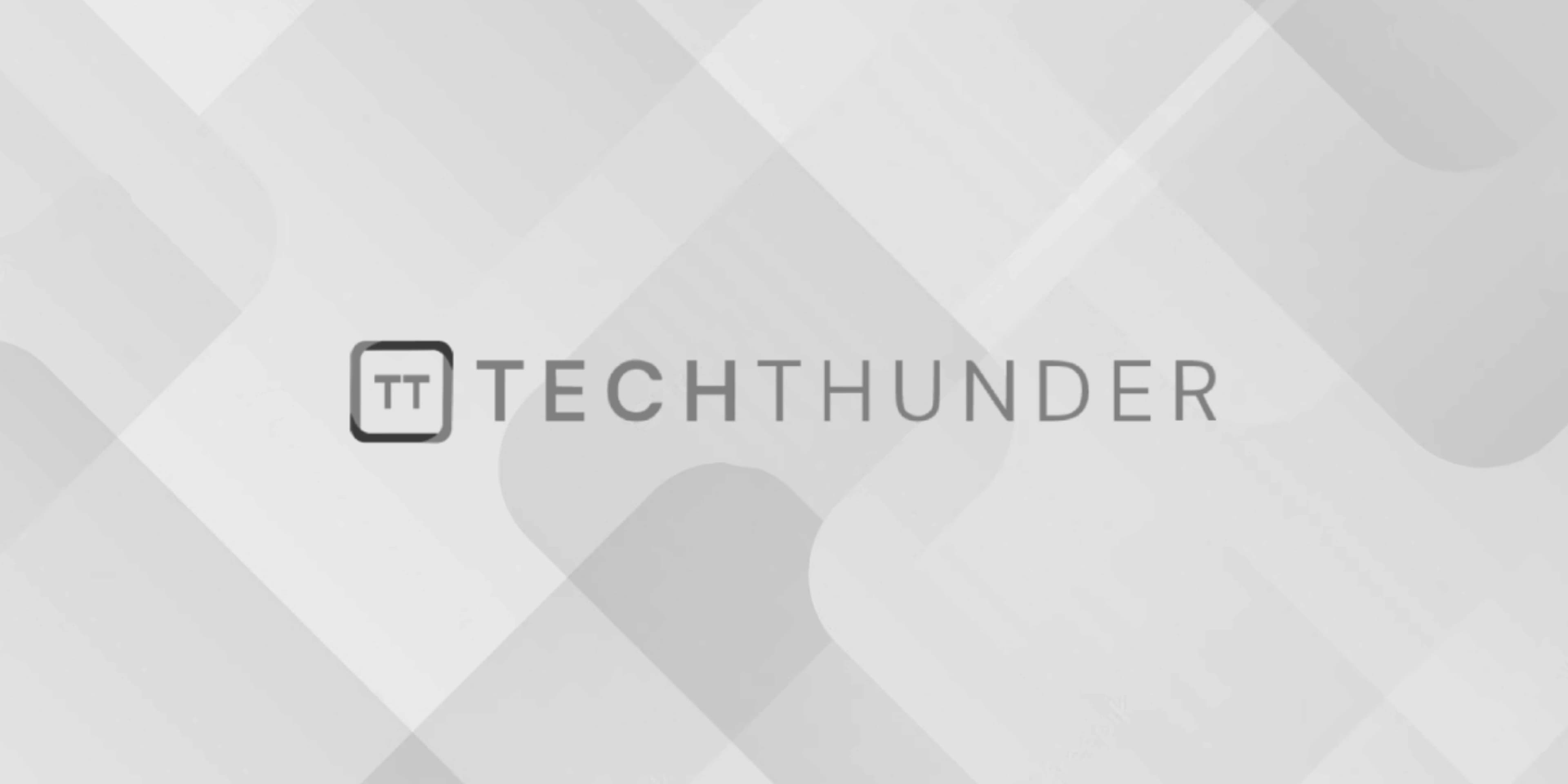
JavaScript Async/Await
Async/await is a feature introduced in ECMAScript 2017 (ES8) that provides a more concise and expressive syntax for working with asynchronous code in JavaScript. It allows you to write asynchronous code in a more synchronous style, making it easier to read and understand.
To use async/await, you need to define an asynchronous function with the async
keyword. Within the async function, you can use the await
keyword to pause the execution and wait for a promise to resolve or reject before proceeding further.
Here’s an example that demonstrates the usage of async/await:
function delay(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function processData() {
console.log('Start');
try {
await delay(2000);
console.log('Async operation 1 complete');
await delay(1500);
console.log('Async operation 2 complete');
} catch (error) {
console.error('An error occurred:', error);
}
console.log('End');
}
processData();
In this example, we have an async
function processData
that performs two asynchronous operations with delays using the delay
function, which returns a promise that resolves after a specified number of milliseconds.
Inside the processData
function, the await
keyword is used to pause the execution and wait for the promises returned by delay
to resolve before moving on to the next statement. This allows the function to execute in a synchronous-like manner, without blocking the main thread.
The await
keyword can only be used inside an async
function. It suspends the execution of the function until the promise is resolved or rejected. If the promise is resolved, the value of the resolved promise is returned. If the promise is rejected, an error is thrown, which can be caught using a try/catch block.
When you run the code, you will see the following output:
Start
Async operation 1 complete
Async operation 2 complete
End
The async/await syntax simplifies the handling of asynchronous operations by eliminating the need for explicit promise chaining or callbacks. It improves code readability and makes asynchronous code flow more intuitively.