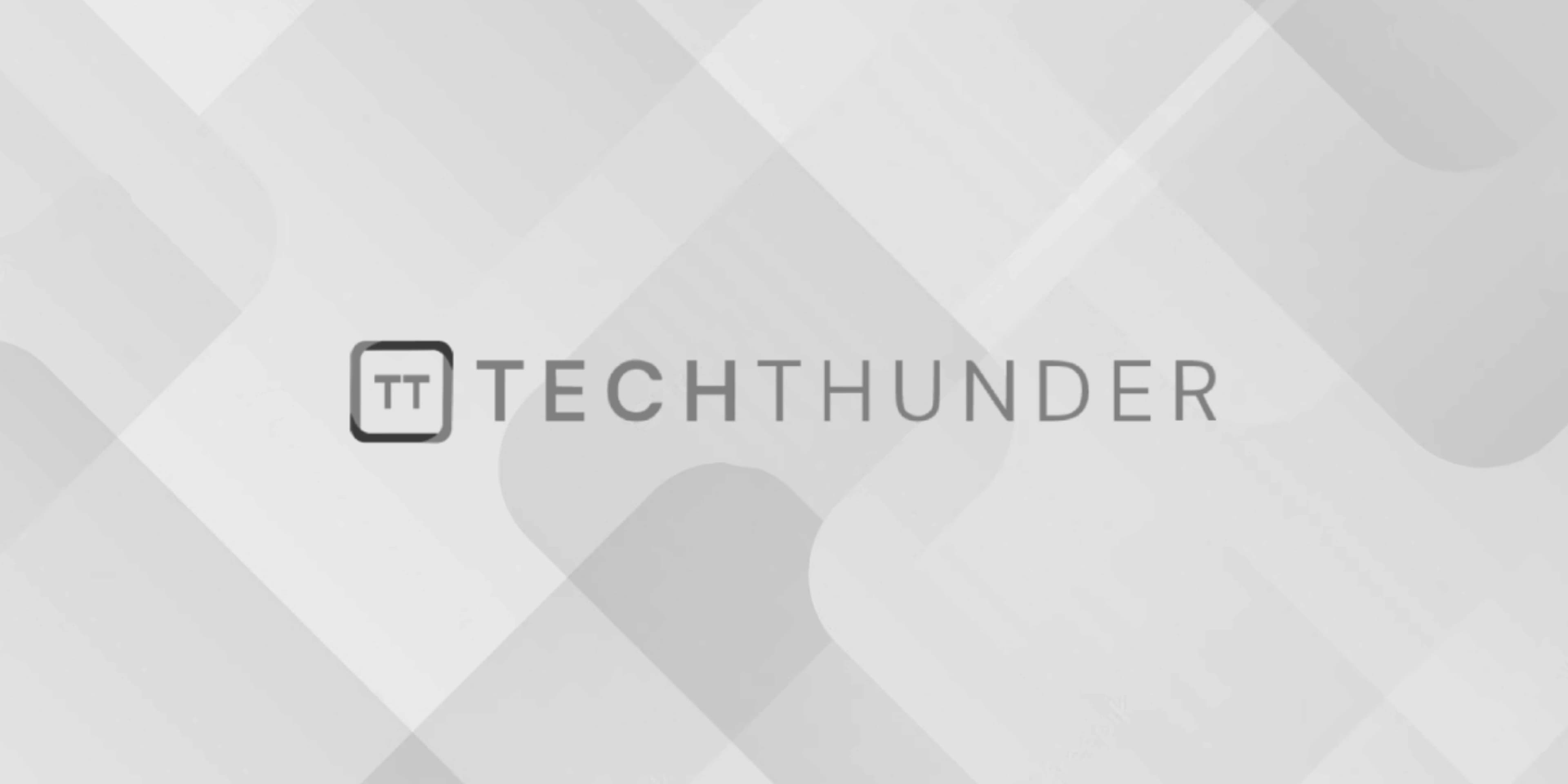
JavaScript Compare dates
The JavaScript can compare dates using the Date objects and various comparison operators. Here’s an example of how you can compare dates:
const date1 = new Date('2022-01-01');
const date2 = new Date('2022-02-01');
// Comparing two dates
if (date1 < date2) {
console.log('date1 is before date2');
} else if (date1 > date2) {
console.log('date1 is after date2');
} else {
console.log('date1 and date2 are the same');
}
// Comparing the current date with a specific date
const currentDate = new Date();
const specificDate = new Date('2022-03-01');
if (currentDate < specificDate) {
console.log('currentDate is before specificDate');
} else if (currentDate > specificDate) {
console.log('currentDate is after specificDate');
} else {
console.log('currentDate and specificDate are the same');
}
In the examples above, we create Date objects representing different dates and then use the less than (<
), greater than (>
), and equal to (===
) operators to compare them. The comparison operators work because the Date objects in JavaScript are internally represented as numeric values, allowing for straightforward comparison.
Keep in mind that when comparing dates, the time component is also taken into account. If you want to compare dates without considering the time, you can set the time component of the Date objects to the same value or use methods like setHours()
, setMinutes()
, and setSeconds()
to set the time to a consistent value.
Additionally, if you’re working with dates in different formats or from different sources, you may need to parse them using appropriate functions like Date.parse()
or by manually extracting the year, month, and day components and constructing a Date object.
Remember to always be mindful of time zones and handle them appropriately when working with dates in JavaScript.