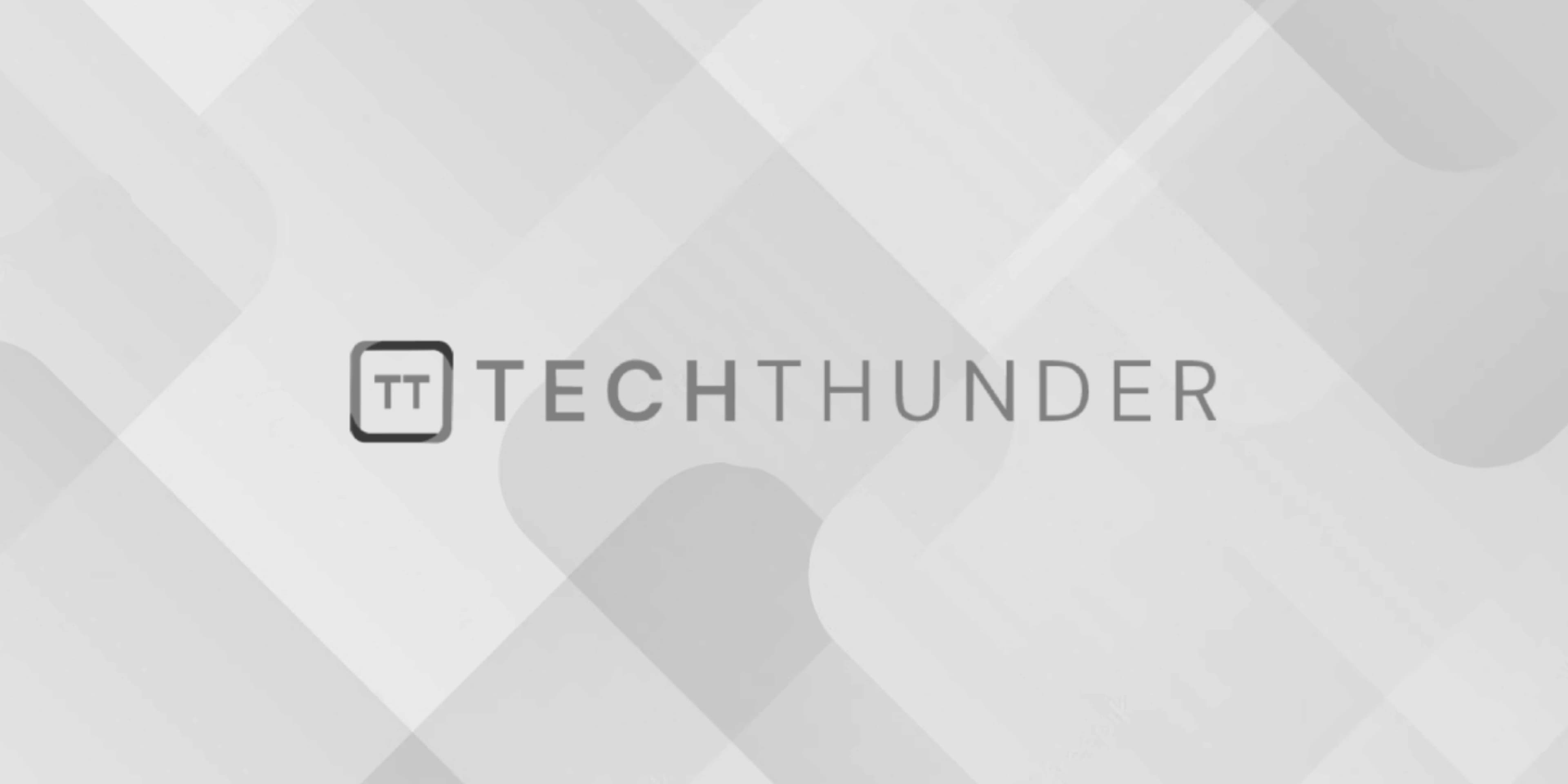
JavaScript Default Parameters
JavaScript default parameters allow you to specify default values for function parameters in case the caller does not provide an argument or provides an undefined value for that parameter. Default parameters provide a convenient way to handle optional arguments or fallback values in functions.
Here’s an example of using default parameters in a function:
function greet(name = 'Anonymous') {
console.log('Hello, ' + name + '!');
}
greet(); // Output: Hello, Anonymous!
greet('John'); // Output: Hello, John!
In this example, the greet
function has a parameter name
with a default value of 'Anonymous'
. If the name
argument is not provided when calling the function, or if it is explicitly set to undefined
, the default value 'Anonymous'
will be used.
When we call greet()
without passing an argument, the default value 'Anonymous'
is used, and the function outputs “Hello, Anonymous!”. When we call greet('John')
with the argument 'John'
, the provided value is used, and the function outputs “Hello, John!”.
Default parameter values can be any valid JavaScript expression, not just simple values. This means you can use function calls or expressions to compute the default value dynamically. For example:
function multiply(a, b = getDefaultMultiplier()) {
return a * b;
}
function getDefaultMultiplier() {
return 2;
}
console.log(multiply(5)); // Output: 10
console.log(multiply(5, 3)); // Output: 15
In this example, the multiply
function has a parameter b
with a default value obtained from the getDefaultMultiplier()
function. If no value is provided for b
, the getDefaultMultiplier()
function is called, and the returned value is used as the default value for b
.
Default parameters are a powerful feature that allows you to define functions with flexible argument handling and provide fallback values when needed. They help make your code more concise and eliminate the need for manual checks for undefined arguments.