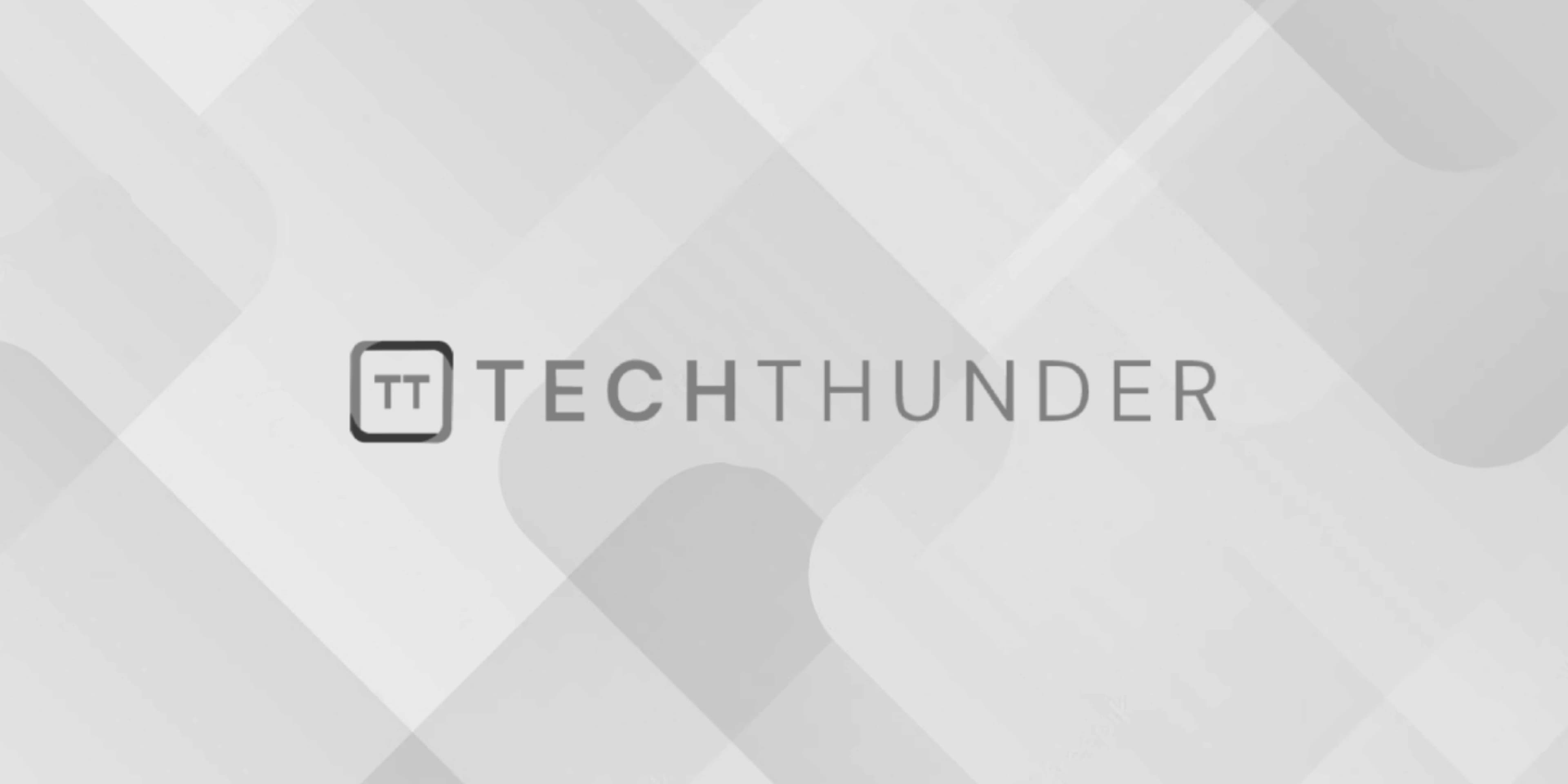
Random image generator in JavaScript
To generate a random image in JavaScript, you can utilize an array of image URLs and select a random URL from that array. Here’s an example of how you can implement a random image generator:
// Array of image URLs
var imageUrls = [
'image1.jpg',
'image2.jpg',
'image3.jpg',
// Add more image URLs as needed
];
// Get a random index from the array
var randomIndex = Math.floor(Math.random() * imageUrls.length);
// Get the random image URL
var randomImageUrl = imageUrls[randomIndex];
// Create an <img> element
var img = document.createElement('img');
// Set the source attribute to the random image URL
img.src = randomImageUrl;
// Append the image to a container element on your page
var container = document.getElementById('imageContainer');
container.appendChild(img);
In this code, you start by defining an array of image URLs (imageUrls
). Each URL represents a different image that can be displayed randomly.
Next, you generate a random index (randomIndex
) using the Math.random()
function. The index is calculated by multiplying a random decimal between 0 and 1 with the length of the imageUrls
array. The Math.floor()
function is then used to round down the result to the nearest whole number.
After that, you retrieve the randomly selected image URL from the imageUrls
array by accessing the element at the random index (imageUrls[randomIndex]
).
Then, you create an <img>
element using the document.createElement()
method.
Set the src
attribute of the <img>
element to the random image URL.
Finally, you append the <img>
element to a container element on your page. Replace 'imageContainer'
with the actual ID of the container element where you want to display the random image.
With this code, each time it runs, a random image URL from the imageUrls
array will be selected, and the corresponding image will be displayed in the specified container on your page.