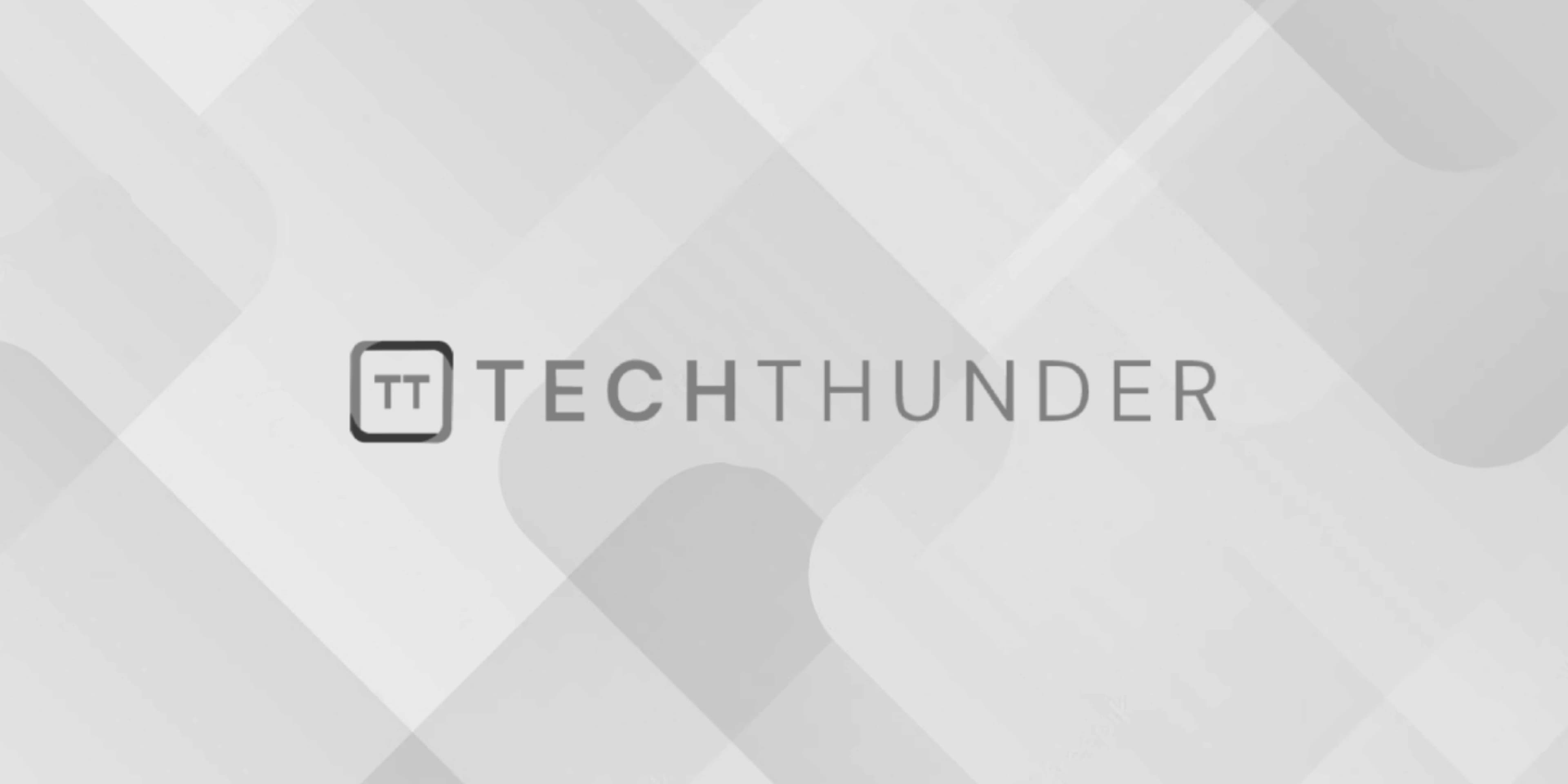
How to make beep sound in JavaScript
The JavaScript can make a beep sound by utilizing the HTML5 <audio>
element and playing a short audio file or by generating a sound using the Web Audio API. Here are examples of both approaches:
- Using an audio file:
function playBeep() {
var audio = new Audio("beep.mp3");
audio.play();
}
// Call the function to play the beep sound
playBeep();
In this example, you would need to have an audio file named “beep.mp3” in the same directory as your HTML file. The Audio
object is created with the audio file’s path, and then the play()
method is called to play the sound.
- Using the Web Audio API:
function playBeep() {
var audioContext = new (window.AudioContext || window.webkitAudioContext)();
var oscillator = audioContext.createOscillator();
oscillator.type = "square";
oscillator.frequency.value = 1000;
oscillator.connect(audioContext.destination);
oscillator.start();
oscillator.stop(audioContext.currentTime + 0.1);
}
// Call the function to play the beep sound
playBeep();
In this example, the Web Audio API is used to generate a short beep sound. An AudioContext
is created, and an Oscillator
is created within the context. The type
property of the oscillator is set to “square” to generate a square wave, and the frequency
property is set to 1000 Hz. The oscillator is then connected to the audio destination (e.g., speakers) using connect()
. Finally, the oscillator is started and stopped after a duration of 0.1 seconds.
You can call the playBeep()
function to play the beep sound.
Both approaches will produce a beep sound, but using an audio file gives you more control over the sound’s characteristics and duration, while the Web Audio API allows you to generate sounds programmatically.