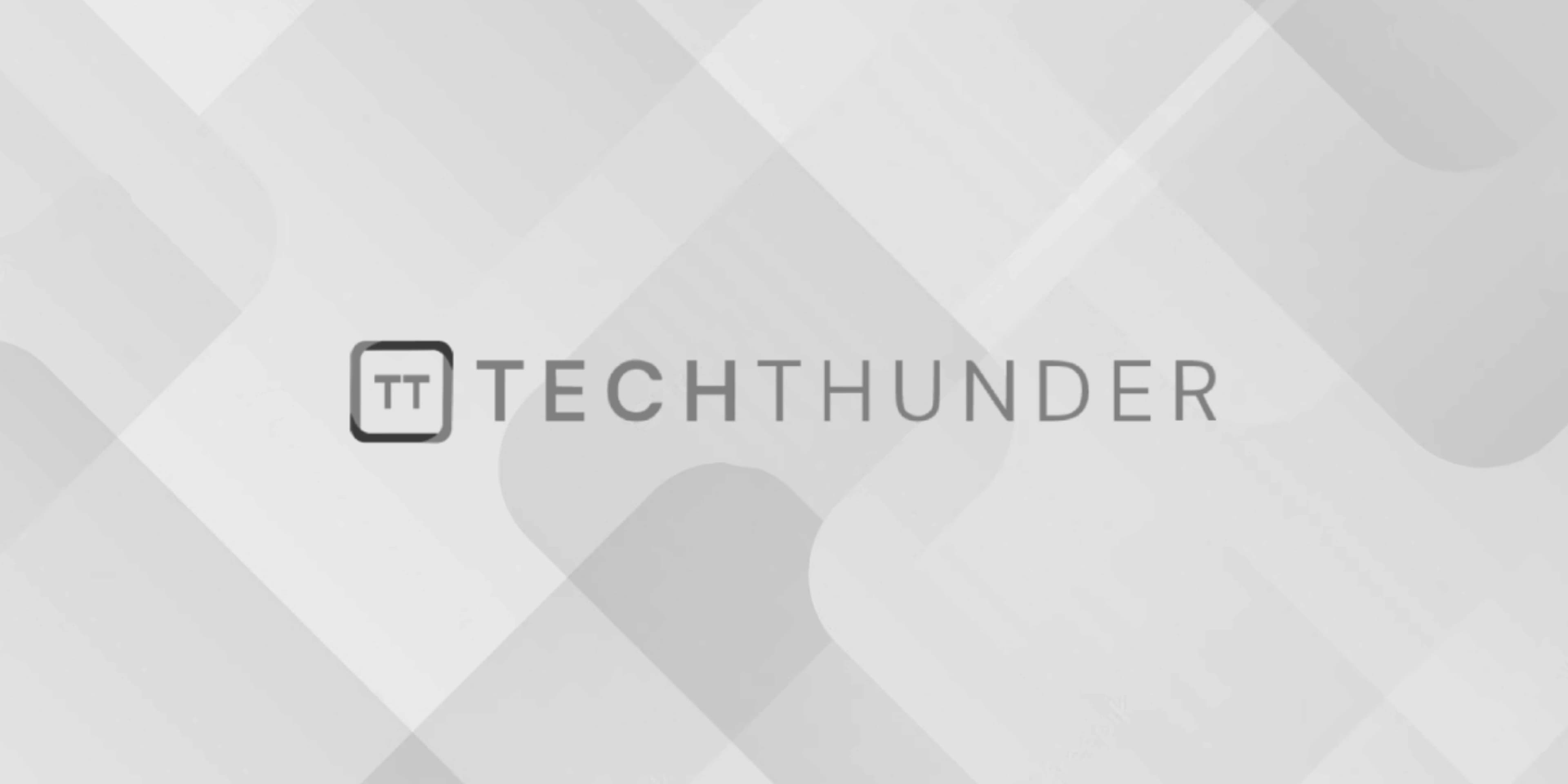
What is a promise in JavaScript
A Promise in JavaScript is an object that represents the eventual completion or failure of an asynchronous operation and its resulting value. It provides a way to handle asynchronous operations more elegantly and avoid callback hell, making code easier to read and maintain.
A Promise has three states:
- Pending: The initial state when the Promise is created, and the asynchronous operation is in progress.
- Fulfilled: The state when the asynchronous operation is successfully completed, and the Promise has a resolved value.
- Rejected: The state when the asynchronous operation encounters an error or fails, and the Promise has a reason for rejection.
The basic syntax to create a Promise is as follows:
const myPromise = new Promise((resolve, reject) => {
// Asynchronous operation
if (/* operation successful */) {
resolve(result); // Resolve the Promise with a value
} else {
reject(error); // Reject the Promise with an error
}
});
In the Promise constructor, you define an asynchronous operation within the callback function, which takes two parameters: resolve
and reject
. These parameters are functions that are used to either fulfill the Promise with a resolved value (resolve(result)
) or reject it with an error (reject(error)
).
You can then handle the fulfillment or rejection of the Promise using the .then()
and .catch()
methods:
myPromise
.then(result => {
// Handle fulfillment
console.log(result);
})
.catch(error => {
// Handle rejection
console.error(error);
});
The .then()
method is used to handle the fulfillment of the Promise, and the resolved value is passed to the callback function as an argument. The .catch()
method is used to handle the rejection of the Promise, and the reason for rejection is passed to the callback function as an argument.
Promises also provide additional methods such as .finally()
for executing code regardless of the Promise’s state and .all()
or .race()
for handling multiple Promises simultaneously.
Promises greatly simplify asynchronous programming by providing a structured way to handle asynchronous operations and their results, making it easier to write and reason about asynchronous code.