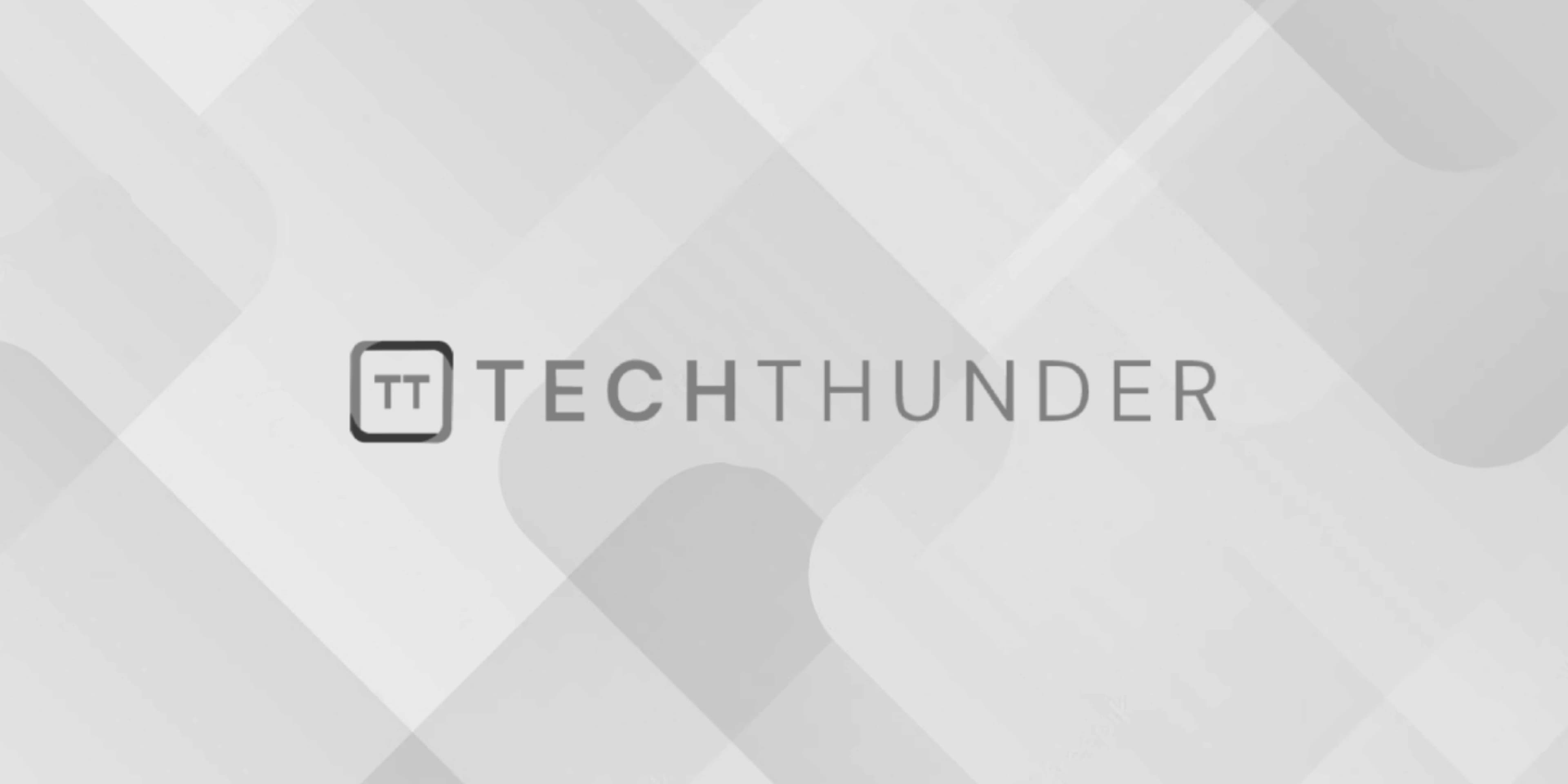
JavaScript Calculator
Here an example of a simple calculator implemented in JavaScript. It supports basic arithmetic operations like addition, subtraction, multiplication, and division.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Calculator</title>
<style>
.calculator {
width: 200px;
padding: 10px;
background-color: #f4f4f4;
border: 1px solid #ccc;
border-radius: 5px;
}
</style>
</head>
<body>
<div class="calculator">
<input type="text" id="result" disabled>
<br>
<button onclick="appendToResult('7')">7</button>
<button onclick="appendToResult('8')">8</button>
<button onclick="appendToResult('9')">9</button>
<button onclick="appendToResult('+')">+</button>
<br>
<button onclick="appendToResult('4')">4</button>
<button onclick="appendToResult('5')">5</button>
<button onclick="appendToResult('6')">6</button>
<button onclick="appendToResult('-')">-</button>
<br>
<button onclick="appendToResult('1')">1</button>
<button onclick="appendToResult('2')">2</button>
<button onclick="appendToResult('3')">3</button>
<button onclick="appendToResult('*')">*</button>
<br>
<button onclick="appendToResult('0')">0</button>
<button onclick="appendToResult('.')">.</button>
<button onclick="calculate()">=</button>
<button onclick="appendToResult('/')">/</button>
<br>
<button onclick="clearResult()">Clear</button>
</div>
<script>
function appendToResult(value) {
var resultInput = document.getElementById("result");
resultInput.value += value;
}
function calculate() {
var resultInput = document.getElementById("result");
var result = eval(resultInput.value); // Using eval for simplicity (not recommended for production use)
resultInput.value = result;
}
function clearResult() {
var resultInput = document.getElementById("result");
resultInput.value = "";
}
</script>
</body>
</html>
Save the above code in an HTML file and open it in a web browser. You’ll see a simple calculator interface with buttons for numbers (0-9), decimal point (.), and arithmetic operators (+, -, *, /). The result is displayed in the input field at the top.
The calculator uses JavaScript functions like appendToResult
, calculate
, and clearResult
to handle button clicks and perform the respective operations. The eval
function is used to evaluate the arithmetic expression entered in the input field, but note that using eval
is not recommended for production use due to security and performance concerns.
Feel free to customize and enhance the calculator according to your requirements!