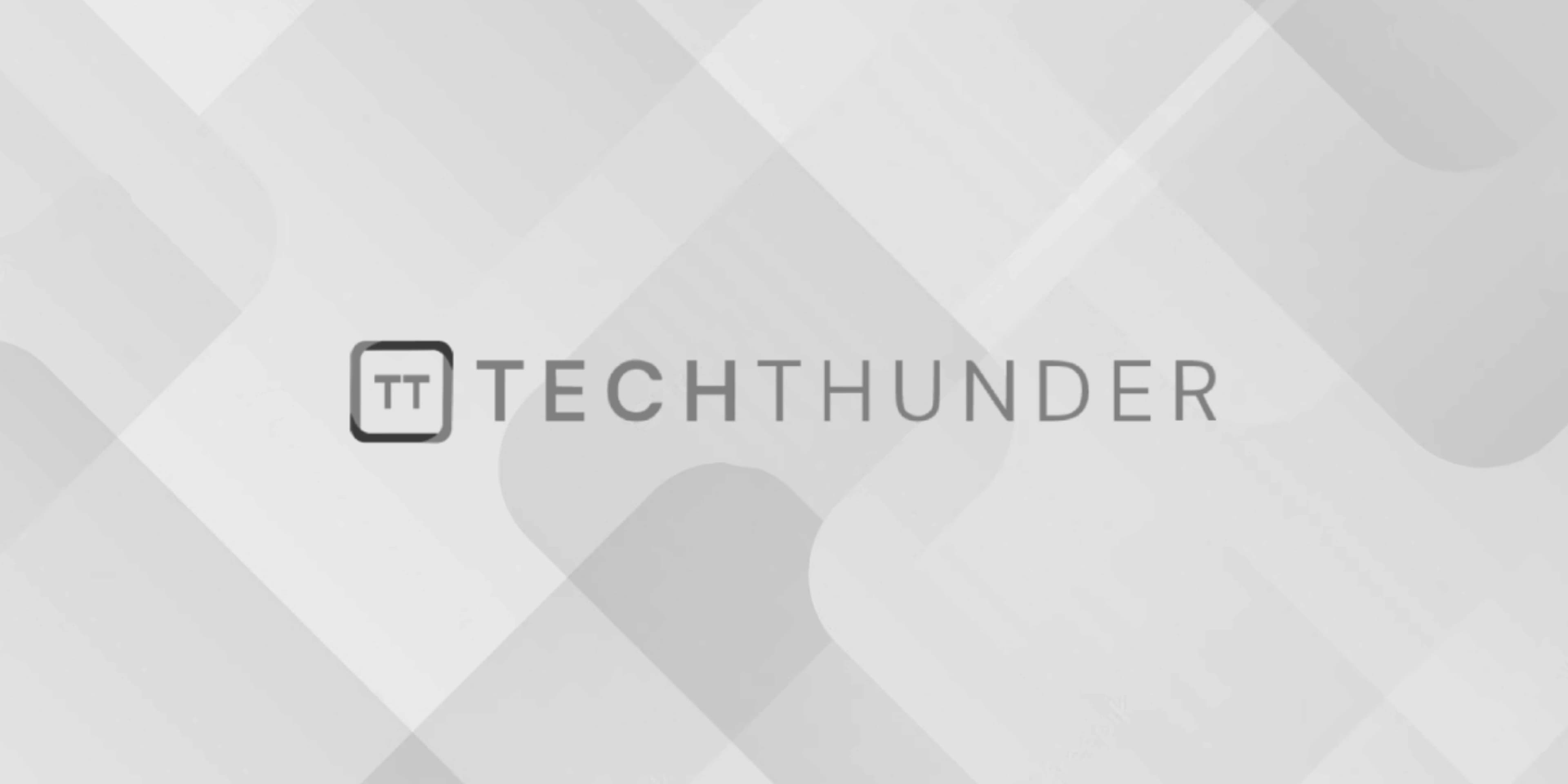
Currying in JavaScript
Currying is a technique in functional programming that involves transforming a function with multiple arguments into a series of functions, each taking a single argument. This allows you to create specialized versions of the original function and provides more flexibility in function composition and reuse.
In JavaScript, you can implement currying using closures and function chaining. Here’s an example to illustrate the concept:
function add(x) {
return function(y) {
return x + y;
};
}
// Curried version of the add function
var addCurried = add(5);
console.log(addCurried(3)); // Outputs: 8
In this example, the add
function takes an argument x
and returns an anonymous inner function that takes another argument y
and returns the sum of x
and y
.
By invoking add(5)
, we create a curried version of the add
function where x
is fixed to 5
. The result is a new function called addCurried
, which expects only the remaining argument y
to be provided.
When we call addCurried(3)
, it adds 3
to the previously fixed value of x
(which is 5
), resulting in 8
as the output.
Currying allows you to partially apply arguments to a function, creating new functions specialized for specific use cases. This can be particularly useful when you want to reuse a function with certain arguments while keeping the flexibility to provide the remaining arguments later.
There are also libraries like Lodash and Ramda that provide utility functions to curry functions in JavaScript. These libraries offer additional features and flexibility for working with curried functions in functional programming scenarios.