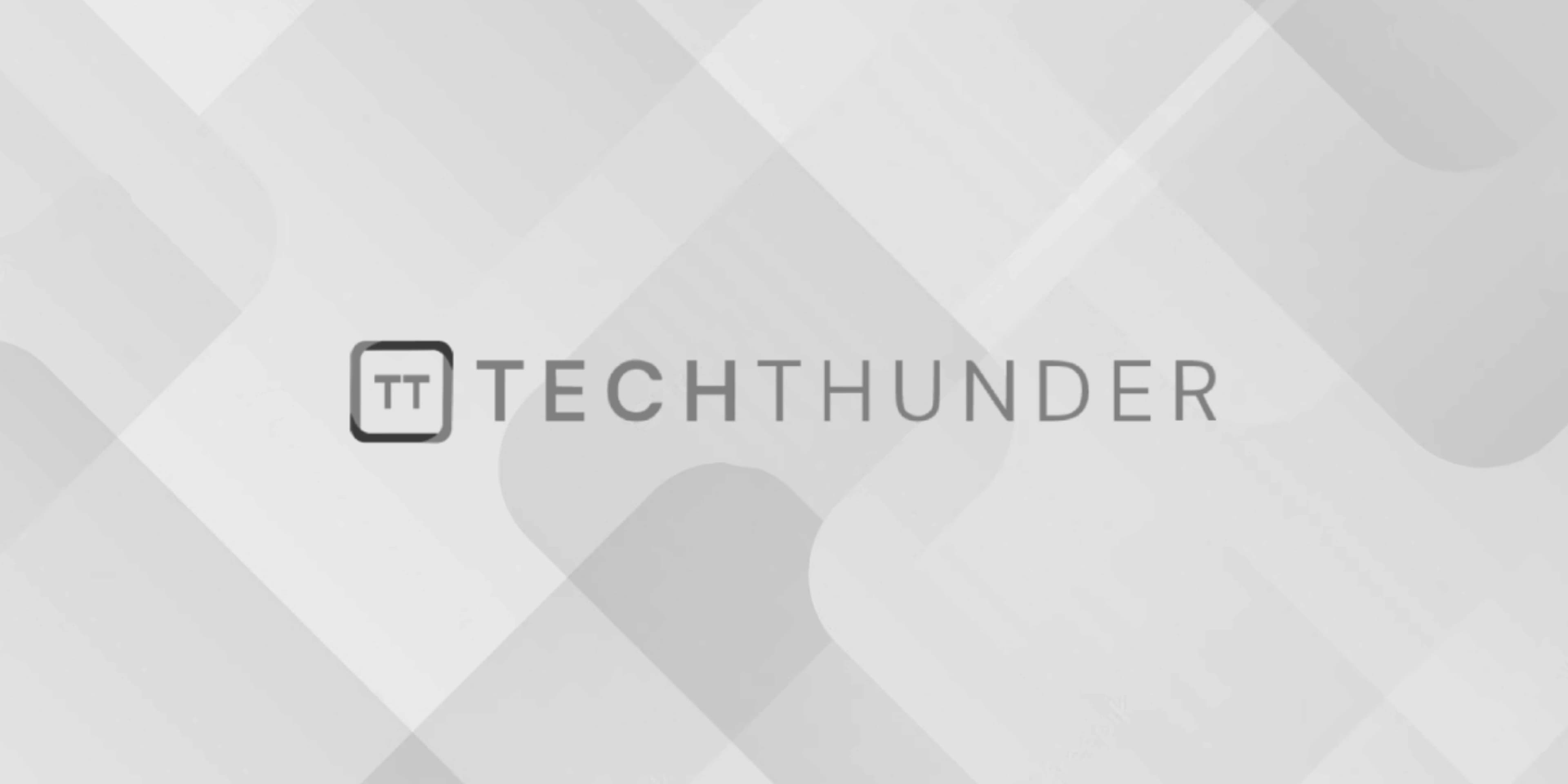
JavaScript let keyword
The let
keyword in JavaScript is used to declare block-scoped variables. It was introduced in ECMAScript 6 (ES6) as an alternative to the var
keyword, which has function scope. Here’s an overview of how the let
keyword works:
Block Scope:
When you declare a variable with let
inside a block (a block is defined by curly braces {}
), the variable is scoped to that block and any nested blocks within it. This means the variable is only accessible within the block where it is defined and not outside of it.
function exampleFunction() {
if (true) {
let blockScopedVar = "Hello";
console.log(blockScopedVar); // "Hello"
}
console.log(blockScopedVar); // Error: blockScopedVar is not defined
}
In the example above, the variable blockScopedVar
is declared with let
inside the if statement block. It is accessible within that block, but attempting to access it outside of the block results in an error.
Block Scoping in Loops:
One common use of let
is to mitigate issues related to variable hoisting in loops. When using var
in a loop, the variable is hoisted to the enclosing function scope, which can cause unintended behavior. However, with let
, a new variable is created for each iteration of the loop, maintaining separate values.
for (let i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
In the above example, using let i
in the loop creates a separate variable i
for each iteration. Each setTimeout
function captures the value of i
at that specific iteration, resulting in the expected output of numbers from 0 to 4 with a delay of one second.
Variable Shadowing:
When using let
, you can declare a variable with the same name as an outer variable in an inner block, effectively shadowing the outer variable. This means the inner variable takes precedence within its block, while the outer variable remains unchanged.
let x = 5;
if (true) {
let x = 10;
console.log(x); // 10
}
console.log(x); // 5
In the example above, the inner x
variable shadows the outer x
variable within the if statement block. The value of the outer x
is not affected, and the two variables exist independently within their respective scopes.
Overall, using the let
keyword provides block scoping for variables, which can help avoid issues related to variable hoisting and provide more predictable code behavior.