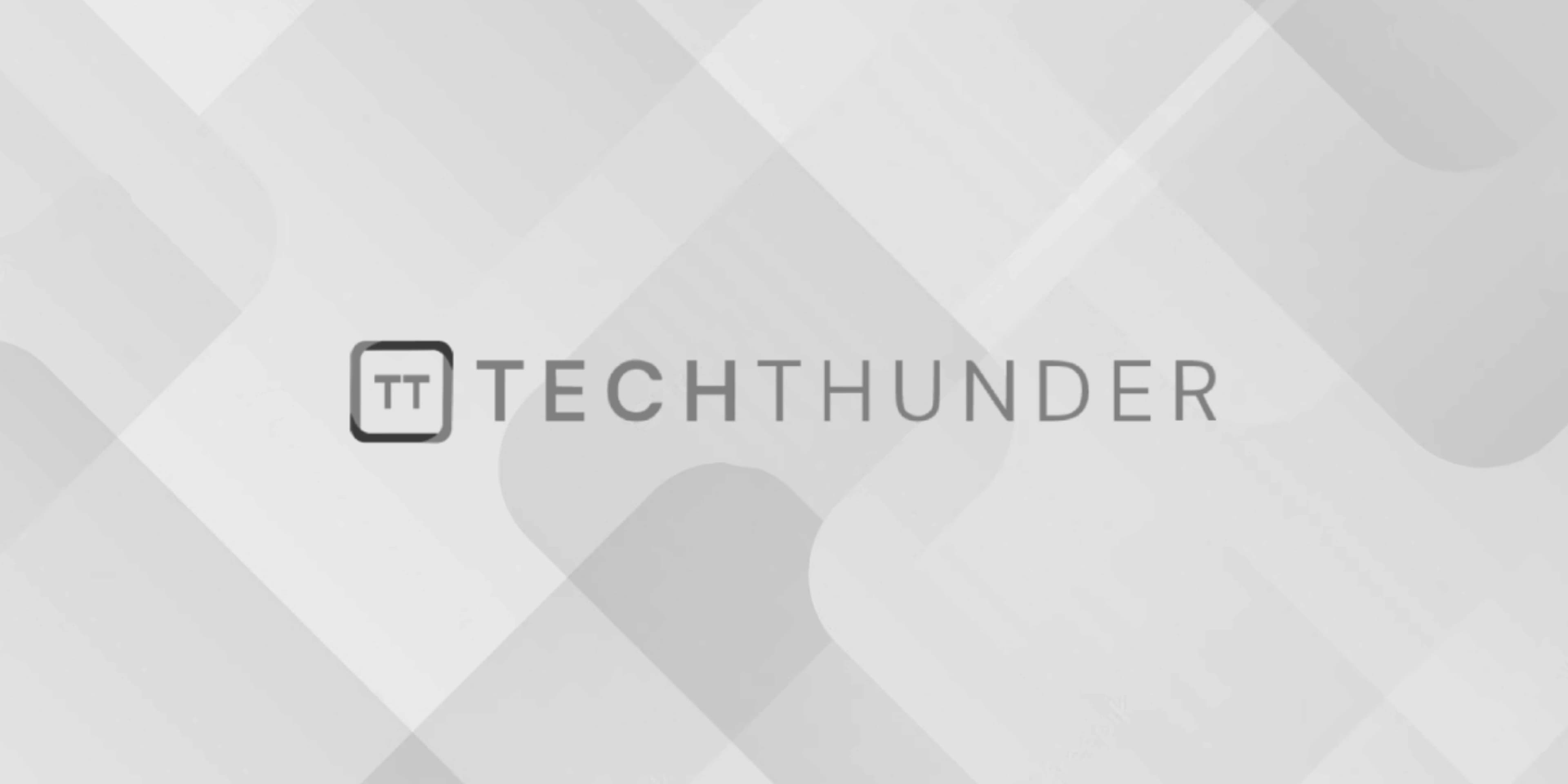
Ripple effect JavaScript
A ripple effect is a visual effect that simulates ripples spreading out from a point of interaction, typically seen when clicking on a button or element. You can create a ripple effect using JavaScript and CSS. Here’s an example:
HTML:
<button class="ripple-effect">Click me</button>
CSS:
.ripple-effect {
position: relative;
overflow: hidden;
}
.ripple-effect:after {
content: "";
position: absolute;
top: 50%;
left: 50%;
width: 0;
height: 0;
border-radius: 50%;
background-color: rgba(255, 255, 255, 0.7);
transform: translate(-50%, -50%);
opacity: 0;
pointer-events: none;
}
.ripple-effect.animate:after {
animation: ripple 0.6s linear;
}
@keyframes ripple {
0% {
width: 0;
height: 0;
opacity: 0.7;
}
100% {
width: 200%;
height: 200%;
opacity: 0;
}
}
JavaScript:
const buttons = document.querySelectorAll('.ripple-effect');
buttons.forEach(button => {
button.addEventListener('click', function(e) {
const ripple = this.querySelector(':after');
ripple.classList.remove('animate');
ripple.style.top = e.clientY - this.offsetTop + 'px';
ripple.style.left = e.clientX - this.offsetLeft + 'px';
ripple.classList.add('animate');
});
});
In this example, we have a button with the class “ripple-effect”. The CSS styles define the appearance and animation of the ripple effect using the :after
pseudo-element.
The JavaScript code adds a click event listener to each button with the class “ripple-effect”. When a button is clicked, it creates a ripple effect by adding and removing the “animate” class to the :after
element. It also positions the ripple at the click coordinates using the e.clientX
and e.clientY
properties.
When you click the button, you should see a ripple effect spreading out from the point of interaction. You can customize the appearance and animation of the ripple effect by modifying the CSS styles.
Note: The above example assumes you have multiple buttons with the “ripple-effect” class. If you have only one button, you can simplify the JavaScript code by directly targeting that button instead of using querySelectorAll
and forEach
.