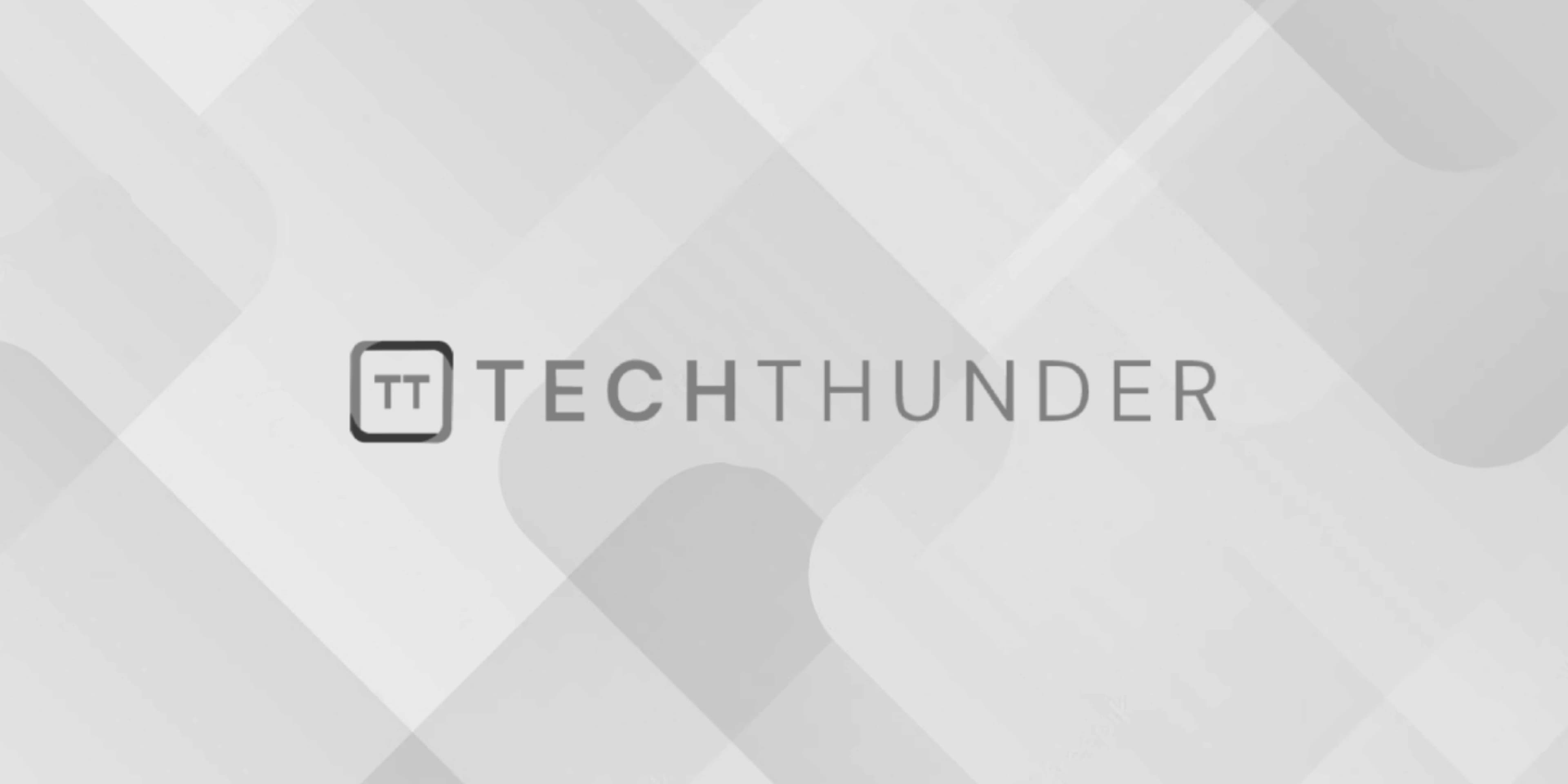
134 views
JavaScript Fetch API
The Fetch API is a modern JavaScript API for making HTTP requests, such as fetching resources from a server. It provides a more powerful and flexible alternative to the older XMLHttpRequest (XHR) object.
Here’s a basic example of how to use the Fetch API to make a GET request:
JavaScript
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not OK');
}
return response.json();
})
.then(data => {
// Process the response data
console.log(data);
})
.catch(error => {
// Handle any errors that occurred during the request
console.error(error);
});
In the example above:
- The
fetch()
function is used to make a GET request to the specified URL (https://api.example.com/data
in this case). It returns a promise that resolves to the response from the server. - In the first
.then()
block, we check if the response was successful using theok
property. If the response is not OK (e.g., a 404 error), we throw an error to be caught in the subsequent.catch()
block. - If the response is OK, we call
response.json()
to parse the response body as JSON and return another promise. - In the second
.then()
block, we have access to the parsed JSON data, which we can process or use as needed. - The
.catch()
block is used to handle any errors that occurred during the request or parsing of the response.
The Fetch API supports various options and configurations for making different types of requests, including specifying request headers, HTTP methods, request body, and more. It also allows you to handle different types of response data, such as JSON, text, or binary data.
For more information and advanced usage of the Fetch API, you can refer to the official documentation or other online resources.