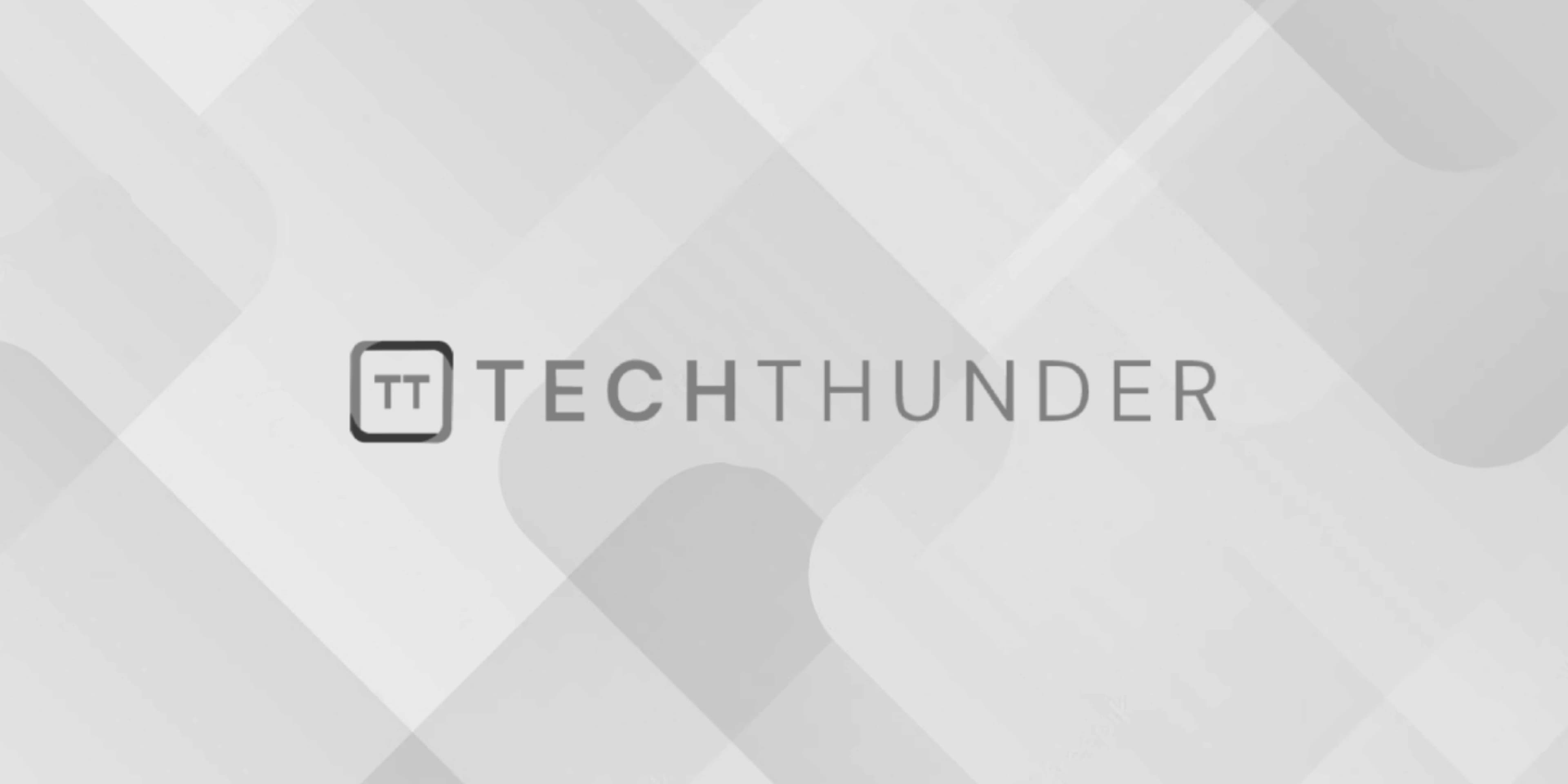
251 views
Check if the array is empty or null, or undefined in JavaScript
To check if an array is empty, null, or undefined in JavaScript, you can use the following approaches:
- Check for empty array: Use the
length
property of the array to determine if it has any elements. An empty array will have alength
of 0.
JavaScript
var array = []; // Empty array
if (array.length === 0) {
console.log('Array is empty');
}
- Check for null or undefined: Use the strict equality operator (
===
) to check if the array is null or undefined.
JavaScript
var array = null; // Null value
if (array === null || array === undefined) {
console.log('Array is null or undefined');
}
Alternatively, you can combine both checks to handle all cases at once:
JavaScript
var array = null; // Null value or undefined
if (array === null || array === undefined || array.length === 0) {
console.log('Array is empty, null, or undefined');
}
Note that the order of the conditions matters. Checking for null or undefined first prevents potential errors when accessing the length
property on a null or undefined value.
It’s important to distinguish between an empty array ([]
) and null/undefined (null
or undefined
) because they represent different states. An empty array signifies an array with no elements, while null or undefined indicates the absence of an array itself.
By using the above checks, you can determine if an array is empty, null, or undefined in JavaScript and handle the appropriate logic accordingly.