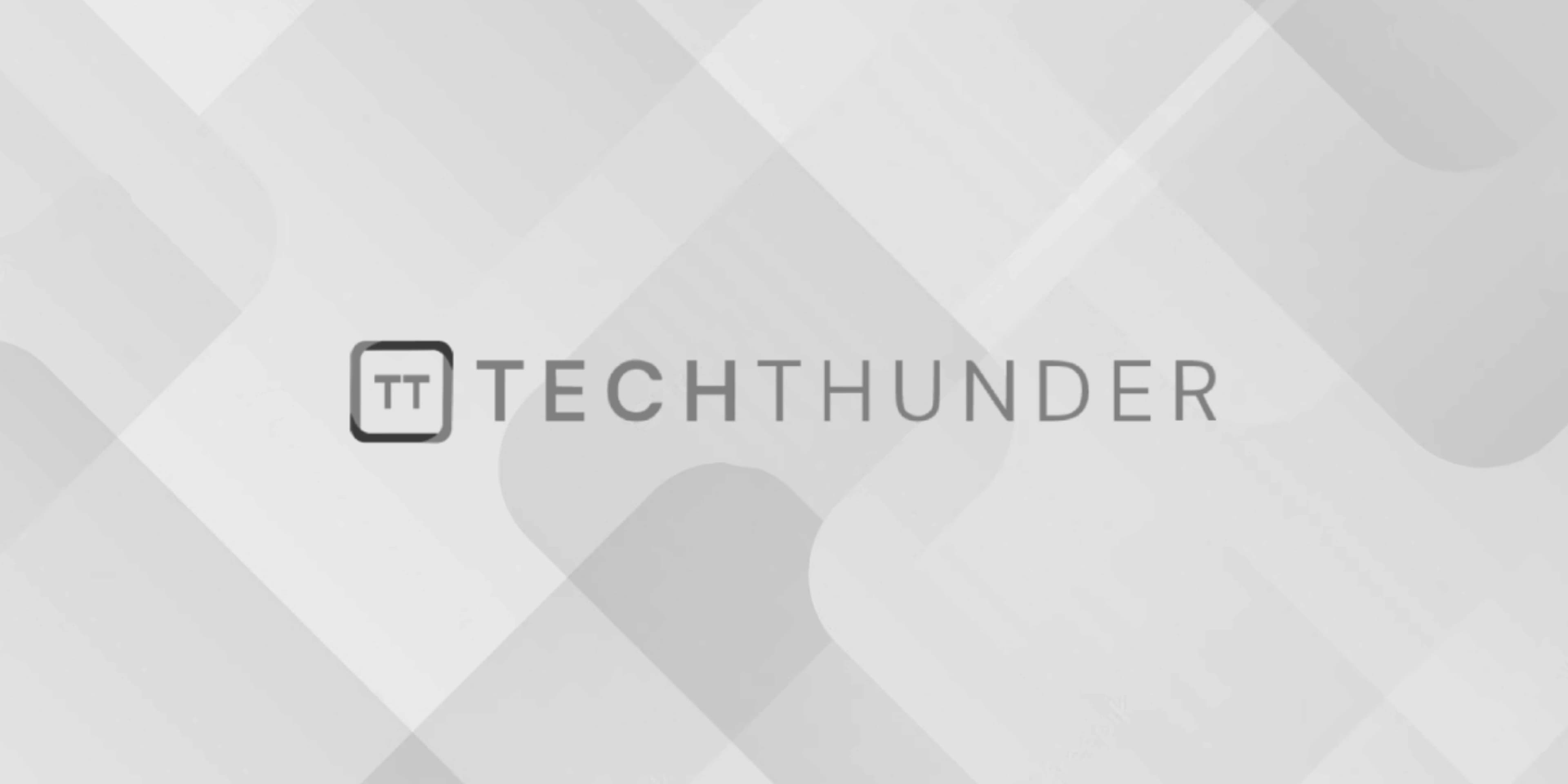
JavaScript Cookies
JavaScript cookies are small pieces of data that can be stored on a user’s computer by a website. They are commonly used to store information about the user’s preferences, session state, or other data that needs to be remembered between different visits to the website.
Here are the basic steps to work with cookies in JavaScript:
1. Creating a Cookie: To create a cookie, you can use the document.cookie
property. Assign a string value to it, following the format “key=value”. For example:
document.cookie = "username=John Doe";
2. Reading a Cookie: To read the value of a cookie, you can access the document.cookie
property. However, this property returns all the cookies as a single string. You’ll need to parse and extract the value you’re interested in. Here’s an example of reading the “username” cookie:
function getCookie(name) {
const cookies = document.cookie.split(';');
for (let i = 0; i < cookies.length; i++) {
const cookie = cookies[i].trim();
if (cookie.startsWith(name + '=')) {
return cookie.substring(name.length + 1);
}
}
return null;
}
const username = getCookie('username');
console.log(username);
3. Updating a Cookie: To update the value of a cookie, you can simply set a new value using the same name. The browser will automatically overwrite the existing cookie with the new value. For example:
document.cookie = "username=Jane Smith";
4. Deleting a Cookie: To delete a cookie, you can set its expiration date to a past date. This will cause the browser to remove the cookie. For example:
document.cookie = "username=; expires=Thu, 01 Jan 1970 00:00:00 UTC";
It’s important to note that cookies have limitations, such as a maximum size and expiration date. Also, cookies are sent to the server with every request, which may impact performance. For more advanced scenarios or to work with cookies more conveniently, you may consider using third-party libraries or frameworks that provide additional functionality and abstraction around cookie handling.