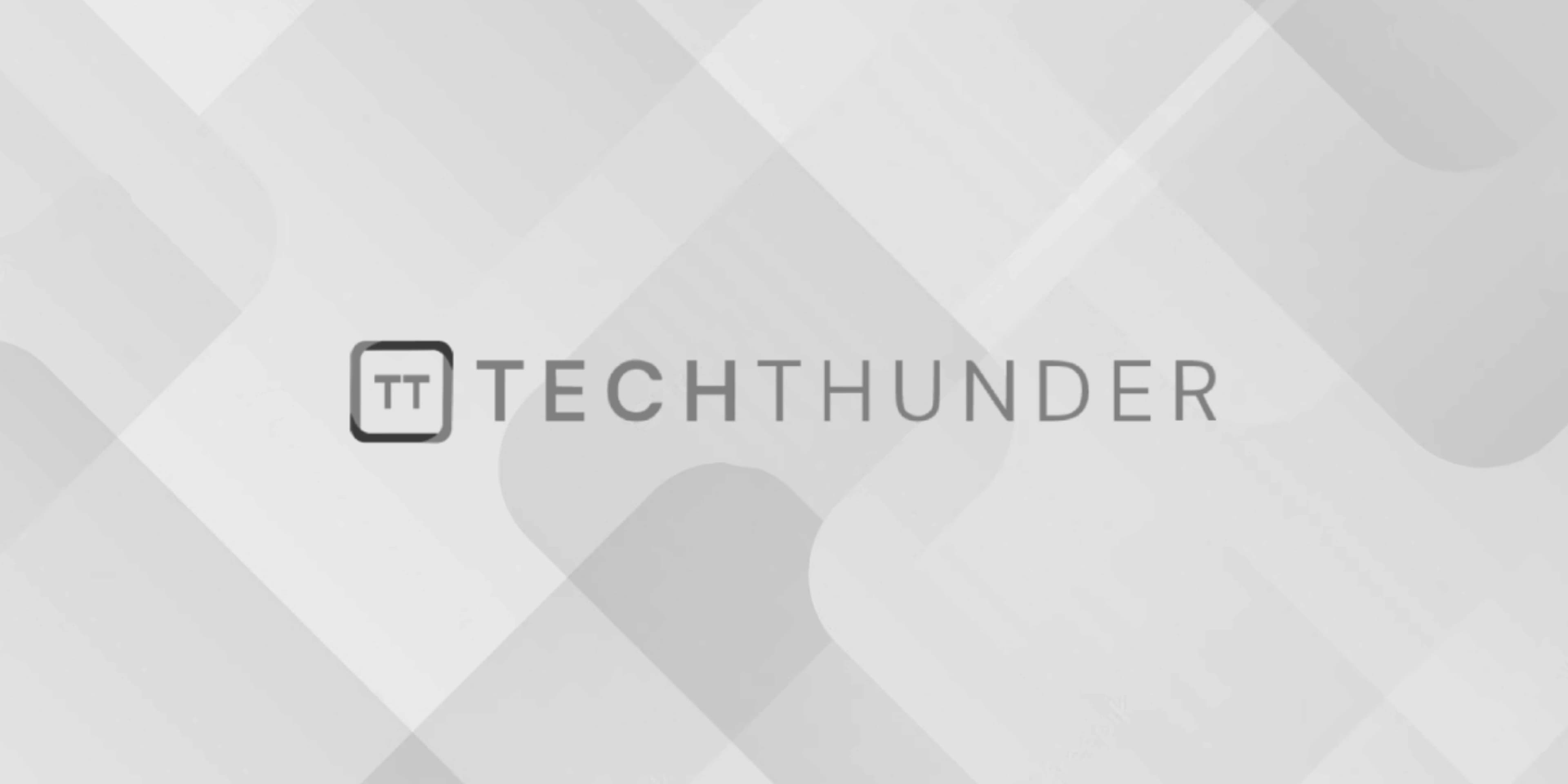
Array to string conversion in PHP
In PHP, the “Array to string conversion” error occurs when you try to use an array as a string in a context where a string is expected. This can happen, for example, when you try to concatenate an array with a string using the dot (.) concatenation operator or when you use an array in a function or operation that expects a string.
To avoid the “Array to string conversion” error, you should make sure you are using arrays and strings correctly in your code. Here are some common scenarios that can trigger this error and how to handle them:
- Concatenating Array with a String:
If you want to concatenate the elements of an array into a string, you can use functions likeimplode()
orjoin()
(they are aliases) to join the array elements into a single string.
Example:
$array = [1, 2, 3];
$string = implode(', ', $array);
echo $string; // Output: "1, 2, 3"
- Using an Array in a Function that Expects a String:
If you pass an array to a function that expects a string, it will trigger the “Array to string conversion” error. Make sure you are passing the correct data type to the function.
Example:
// Incorrect usage - passing an array to the 'strlen()' function
$array = [1, 2, 3];
$length = strlen($array); // Error: Array to string conversion
// Correct usage - passing a string to the 'strlen()' function
$string = "Hello, World!";
$length = strlen($string); // Returns the length of the string
- Debugging:
If you encounter the “Array to string conversion” error and you are not sure which part of your code is causing it, you can use thevar_dump()
orprint_r()
functions to inspect the content and data types of your variables.
Example:
$array = [1, 2, 3];
var_dump($array); // Outputs the array structure and its elements
By checking the output of var_dump()
or print_r()
, you can identify if there’s an array where a string is expected.
Remember that arrays and strings are different data types in PHP, and you need to handle them accordingly in your code. Using appropriate functions and ensuring the data types match the context where they are used will help you avoid “Array to string conversion” errors.