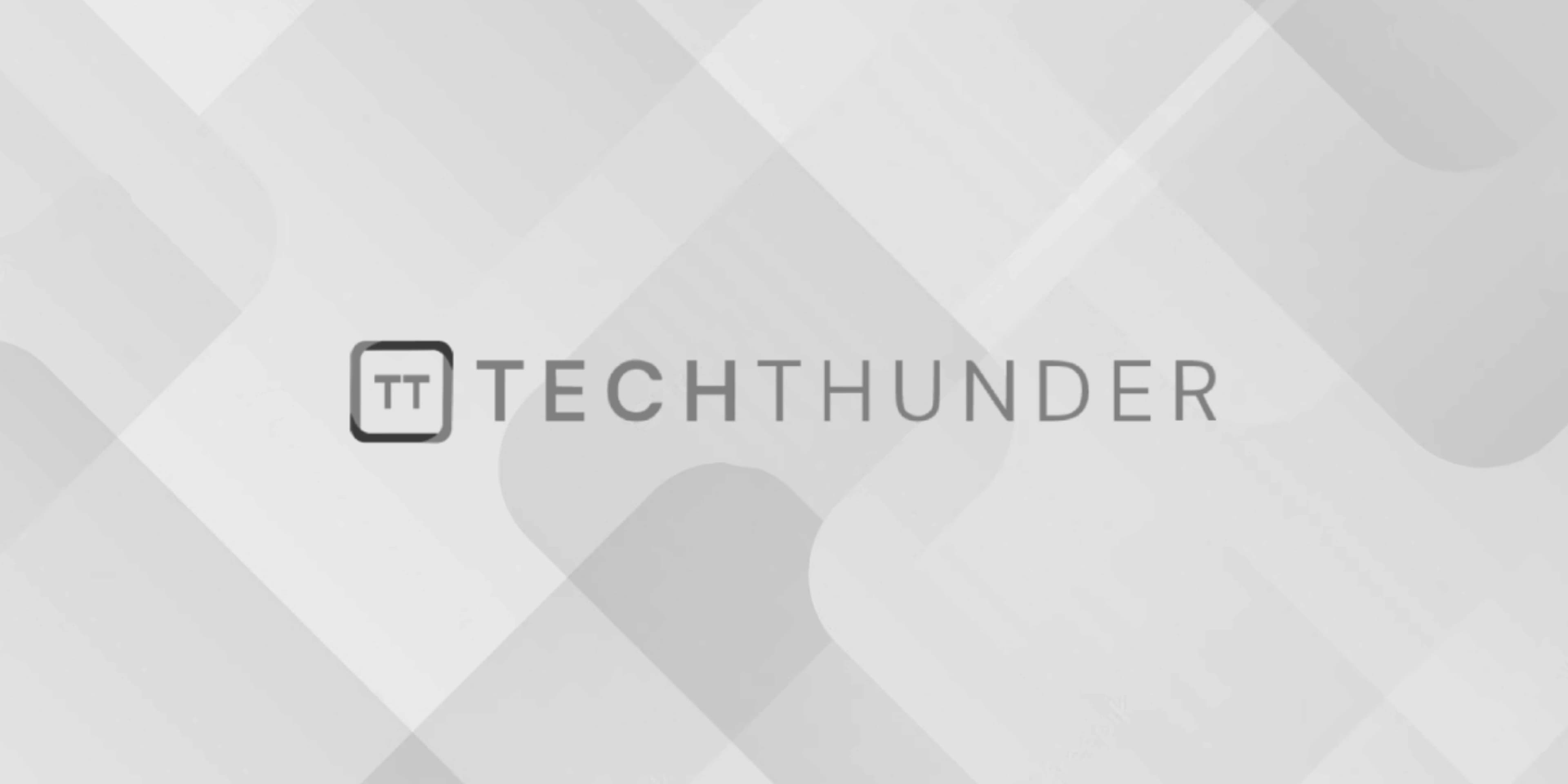
OOPs Constructor
In object-oriented programming (OOP), a constructor is a special method defined within a class that is automatically called when an object of the class is created. Constructors are used to initialize the object’s properties or perform any necessary setup tasks when the object is instantiated.
In PHP, the constructor method is named __construct()
(double underscore before “construct”). When an object of a class is created using the new
keyword, PHP automatically looks for and executes the __construct()
method if it is defined within the class.
Here’s the syntax for defining a constructor in PHP:
class ClassName {
public function __construct() {
// Constructor code here
}
}
Example:
class Person {
public $name;
public $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
echo "Object created. Name: {$this->name}, Age: {$this->age}\n";
}
}
// Creating an object of the class automatically calls the constructor
$person1 = new Person("John Doe", 30); // Output: Object created. Name: John Doe, Age: 30
In the example above, we defined a Person
class with a constructor that takes two parameters: $name
and $age
. When an object of the class is created with the new
keyword, the constructor is automatically called with the provided arguments, and it initializes the object’s properties.
Constructors are beneficial when you want to ensure that certain initialization steps are always performed when an object is created. For example, you can set default property values, establish database connections, or perform any other setup tasks required for the object to function correctly.
If a class does not have a constructor defined, PHP will use a default constructor that does nothing. However, it’s a good practice to explicitly define constructors in your classes to ensure proper object initialization and avoid unexpected behavior. Additionally, constructors can accept any number of parameters, allowing you to pass initial values to the object during instantiation.