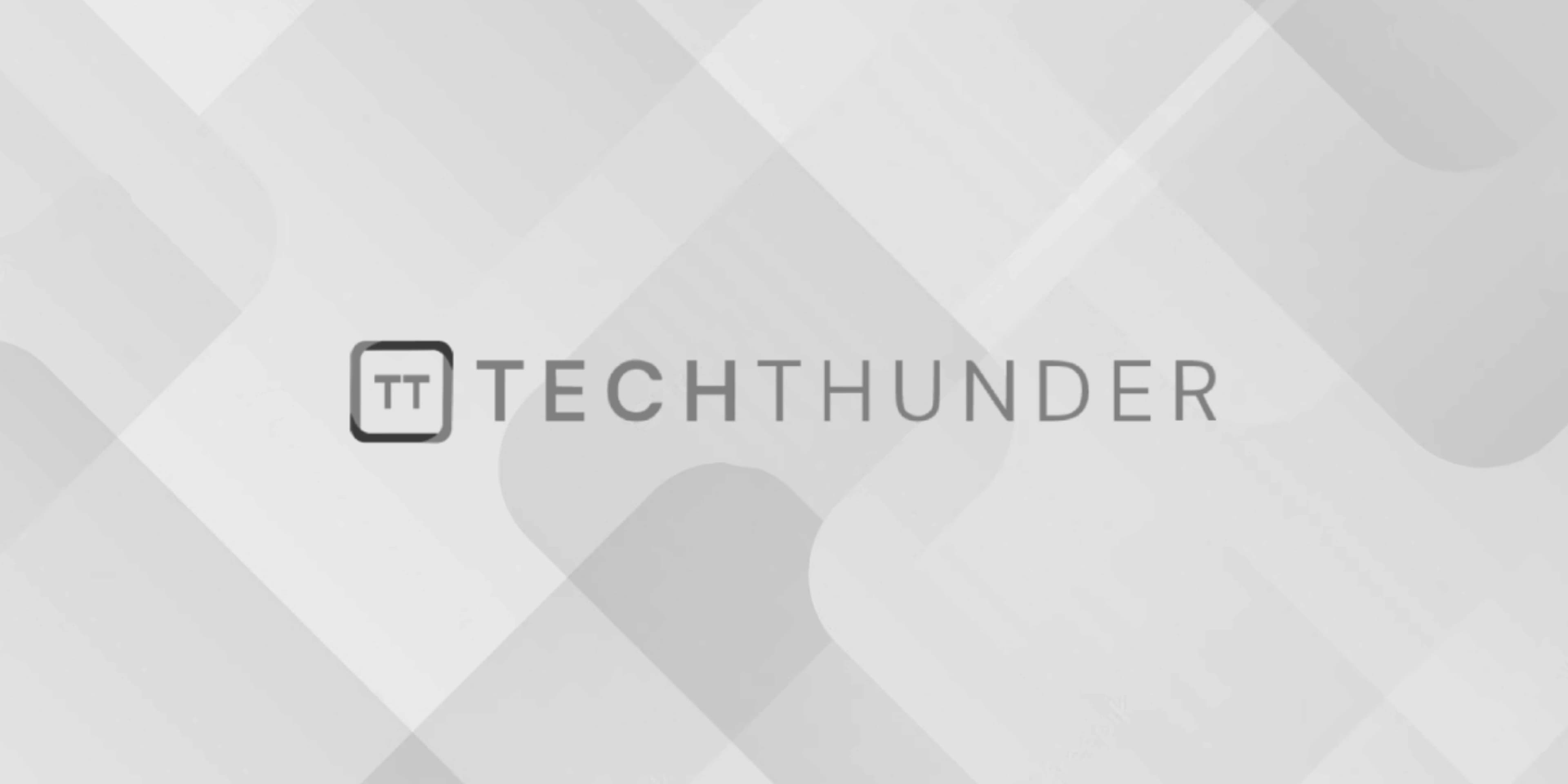
PHP preg_replace() function
In PHP, preg_replace()
is a built-in function used for performing regular expression-based string replacements. It allows you to search for a specific pattern within a string and replace all occurrences of that pattern with a given replacement.
Syntax:
The basic syntax of preg_replace()
is as follows:
preg_replace($pattern, $replacement, $subject);
Parameters:
$pattern
: The regular expression pattern you want to search for.$replacement
: The replacement string that will be used to replace the matched patterns.$subject
: The string in which you want to perform the search and replacement.
Return Value:
The preg_replace()
function returns a new string with all occurrences of the pattern replaced by the replacement string. If no matches are found, it returns the original subject string unchanged.
Example:
$subject = "The quick brown fox jumps over the lazy dog.";
$pattern = "/quick.*fox/";
$replacement = "swift white cat";
$result = preg_replace($pattern, $replacement, $subject);
echo $result;
Output: The swift white cat jumps over the lazy dog.
In this example, the regular expression pattern "/quick.*fox/"
searches for the substring “quick” followed by any characters (.*
) and then followed by the substring “fox”. The preg_replace()
function finds all occurrences of this pattern in the $subject
string and replaces them with the $replacement
string “swift white cat”.
It’s important to note that preg_replace()
performs a global replacement by default, which means it replaces all occurrences of the pattern in the subject string. If you only want to replace the first occurrence, you can use the limit
parameter as the fourth argument:
$subject = "The quick brown fox jumps over the lazy dog.";
$pattern = "/quick.*fox/";
$replacement = "swift white cat";
$result = preg_replace($pattern, $replacement, $subject, 1);
echo $result;
Output: The swift white cat jumps over the lazy dog.
In this case, only the first occurrence of the pattern is replaced.
preg_replace()
is particularly useful when you need to perform advanced and flexible string replacements based on patterns. Regular expressions allow you to define complex patterns to search for, making the function versatile for various text manipulation tasks. However, be cautious when using regular expressions, as they can be computationally expensive and may require proper escaping to prevent unintended side effects.