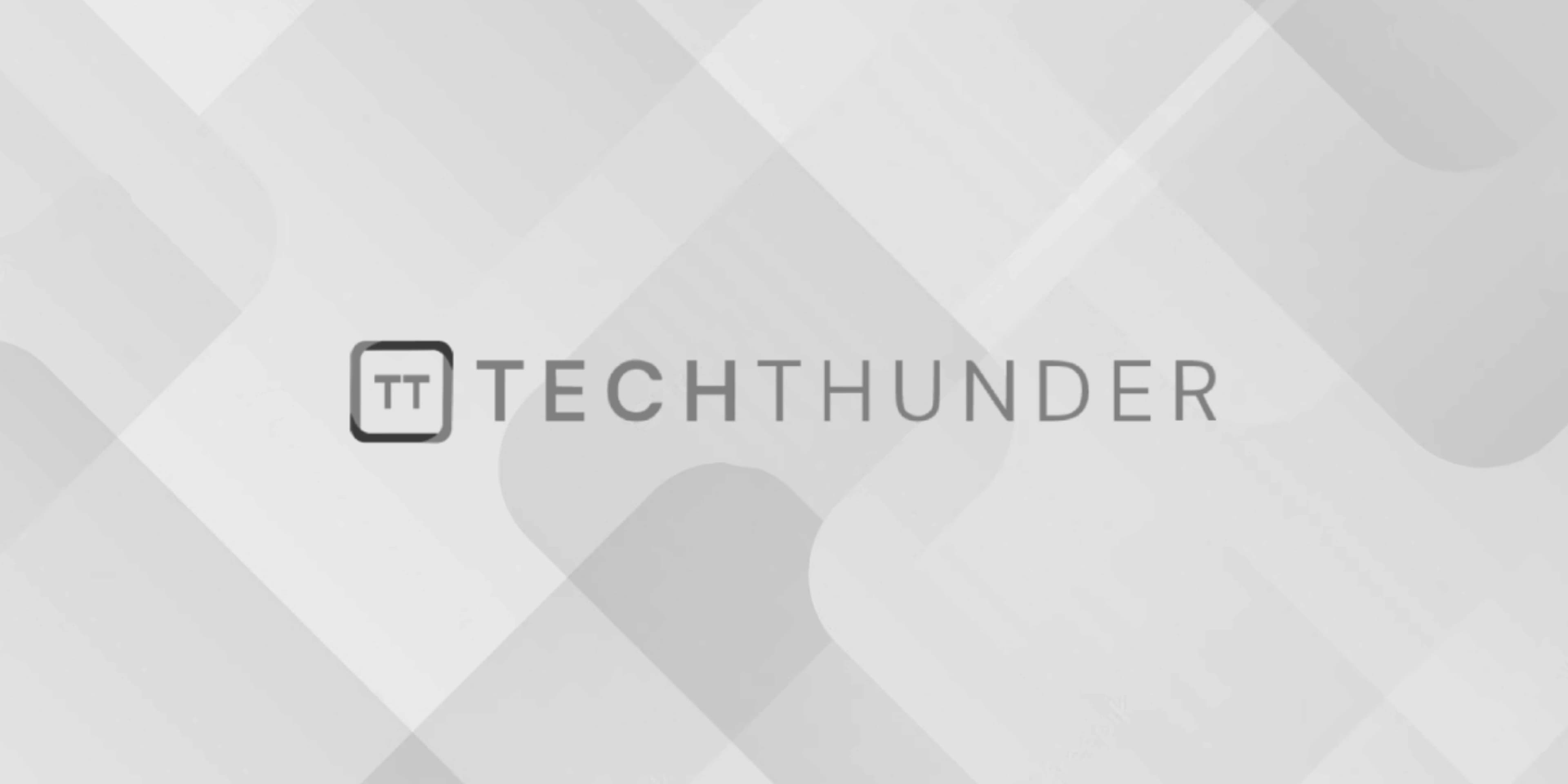
267 views
PHP Inheritance Task
Sure! Let’s create a simple PHP inheritance task to demonstrate the concept of inheritance in object-oriented programming.
Task: Create a base class called Animal
and two derived classes Dog
and Cat
. The Animal
class should have a property called name
and a method called makeSound()
. The makeSound()
method should be overridden in the Dog
and Cat
classes to produce different sounds for each animal.
Here’s how you can implement the task:
<?php
// Base class
class Animal {
protected $name;
// Constructor to set the name of the animal
public function __construct($name) {
$this->name = $name;
}
// Method to make a generic sound (to be overridden in derived classes)
public function makeSound() {
echo "Generic animal sound!\n";
}
}
// Derived class Dog
class Dog extends Animal {
public function __construct($name) {
parent::__construct($name);
}
// Override the makeSound() method to produce a dog sound
public function makeSound() {
echo "Woof! Woof!\n";
}
}
// Derived class Cat
class Cat extends Animal {
public function __construct($name) {
parent::__construct($name);
}
// Override the makeSound() method to produce a cat sound
public function makeSound() {
echo "Meow! Meow!\n";
}
}
// Create instances of Dog and Cat
$dog = new Dog("Buddy");
$cat = new Cat("Whiskers");
// Call the makeSound() method of each animal
echo $dog->name . " says: ";
$dog->makeSound();
echo $cat->name . " says: ";
$cat->makeSound();
?>
Output:
Buddy says: Woof! Woof!
Whiskers says: Meow! Meow!
Explanation:
- We define a base class
Animal
with a property$name
and a methodmakeSound()
. ThemakeSound()
method is a generic method that can be overridden in derived classes. - We define two derived classes,
Dog
andCat
, that extend theAnimal
class. Each derived class has its own implementation of themakeSound()
method. - In the
Dog
class, we override themakeSound()
method to produce a dog sound (“Woof! Woof!”). - In the
Cat
class, we override themakeSound()
method to produce a cat sound (“Meow! Meow!”). - We create instances of
Dog
andCat
, and then call themakeSound()
method on each animal to hear their respective sounds.
Inheritance allows the Dog
and Cat
classes to inherit properties and methods from the Animal
class, reducing code duplication and promoting code reusability.