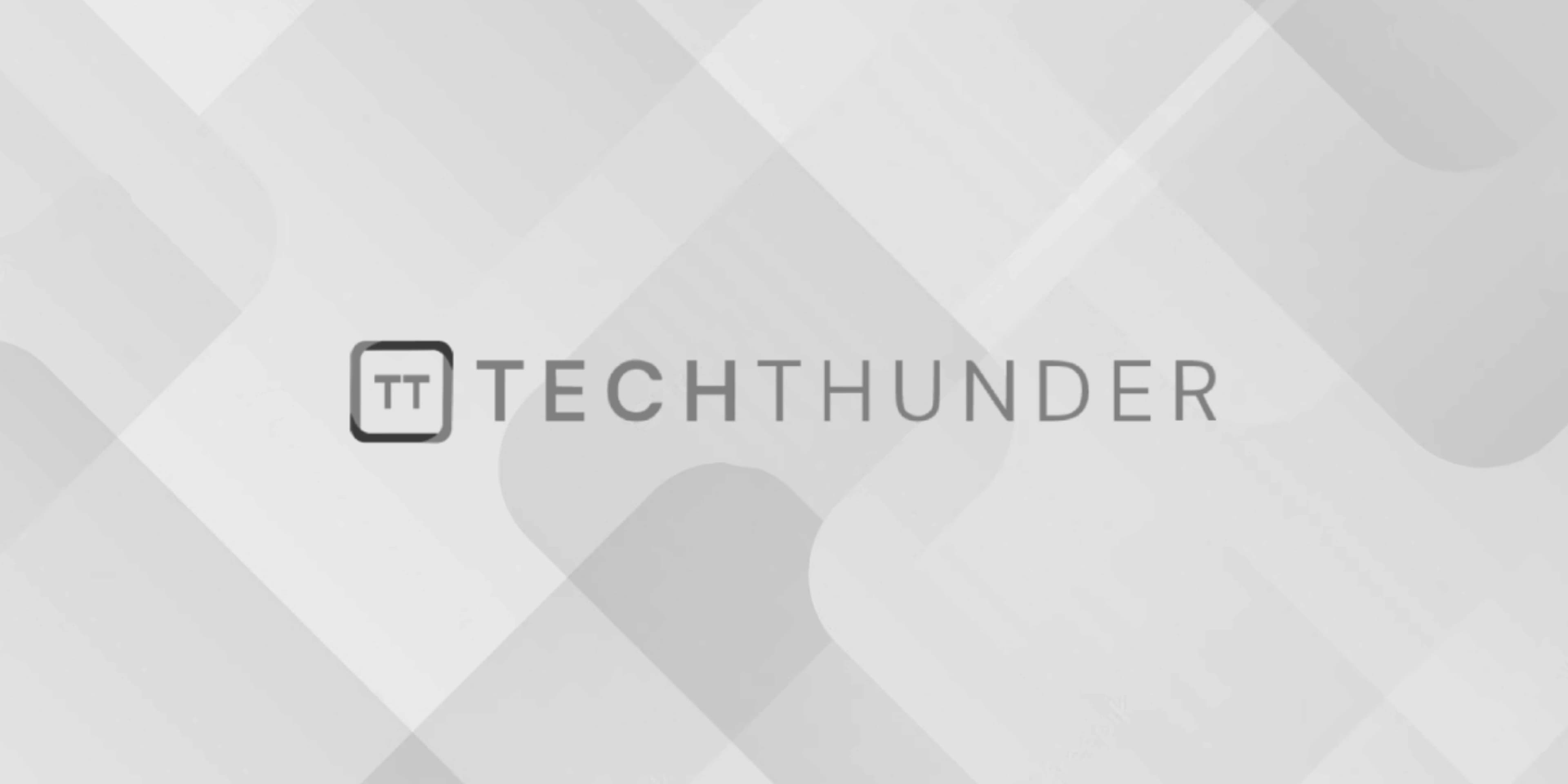
PHP try-catch
In PHP, try
and catch
are keywords used for exception handling. They allow you to manage and respond to runtime errors and exceptions in a more structured and controlled manner. The try
block contains the code that might throw an exception, and the catch
block is used to handle the caught exception.
Here’s the basic syntax of the try-catch
block:
try {
// Code that may throw an exception
} catch (ExceptionType $exception) {
// Code to handle the caught exception
}
try
: Thetry
block contains the code that you want to monitor for exceptions. If an exception occurs within this block, PHP will immediately stop executing the code inside thetry
block and jump to the correspondingcatch
block.catch
: Thecatch
block is where you define how to handle the caught exception. TheExceptionType
is the type of exception you want to catch. You can specify the specific exception class you want to catch or use the more generalException
class to catch all types of exceptions.
Here’s an example of using try-catch
in PHP:
function divide($numerator, $denominator) {
if ($denominator === 0) {
throw new Exception("Division by zero is not allowed.");
}
return $numerator / $denominator;
}
try {
$result = divide(10, 0);
echo "Result: " . $result; // This line will not be executed due to the exception
} catch (Exception $e) {
echo "Exception caught: " . $e->getMessage(); // Output: Exception caught: Division by zero is not allowed.
}
In this example, the divide()
function attempts to divide a number by zero, which is not allowed. Therefore, an exception is thrown within the try
block. As a result, the code inside the try
block is immediately halted, and PHP jumps to the catch
block, where the caught exception is handled. The caught exception’s message is then displayed using $e->getMessage()
.
You can catch different types of exceptions by using specific exception classes. For example, if you have a custom exception class named CustomException
, you can catch it using catch (CustomException $e) { ... }
.
Exception handling is essential for maintaining the stability and reliability of your PHP applications. It allows you to gracefully handle errors, log them for debugging purposes, and respond appropriately to different types of exceptions.