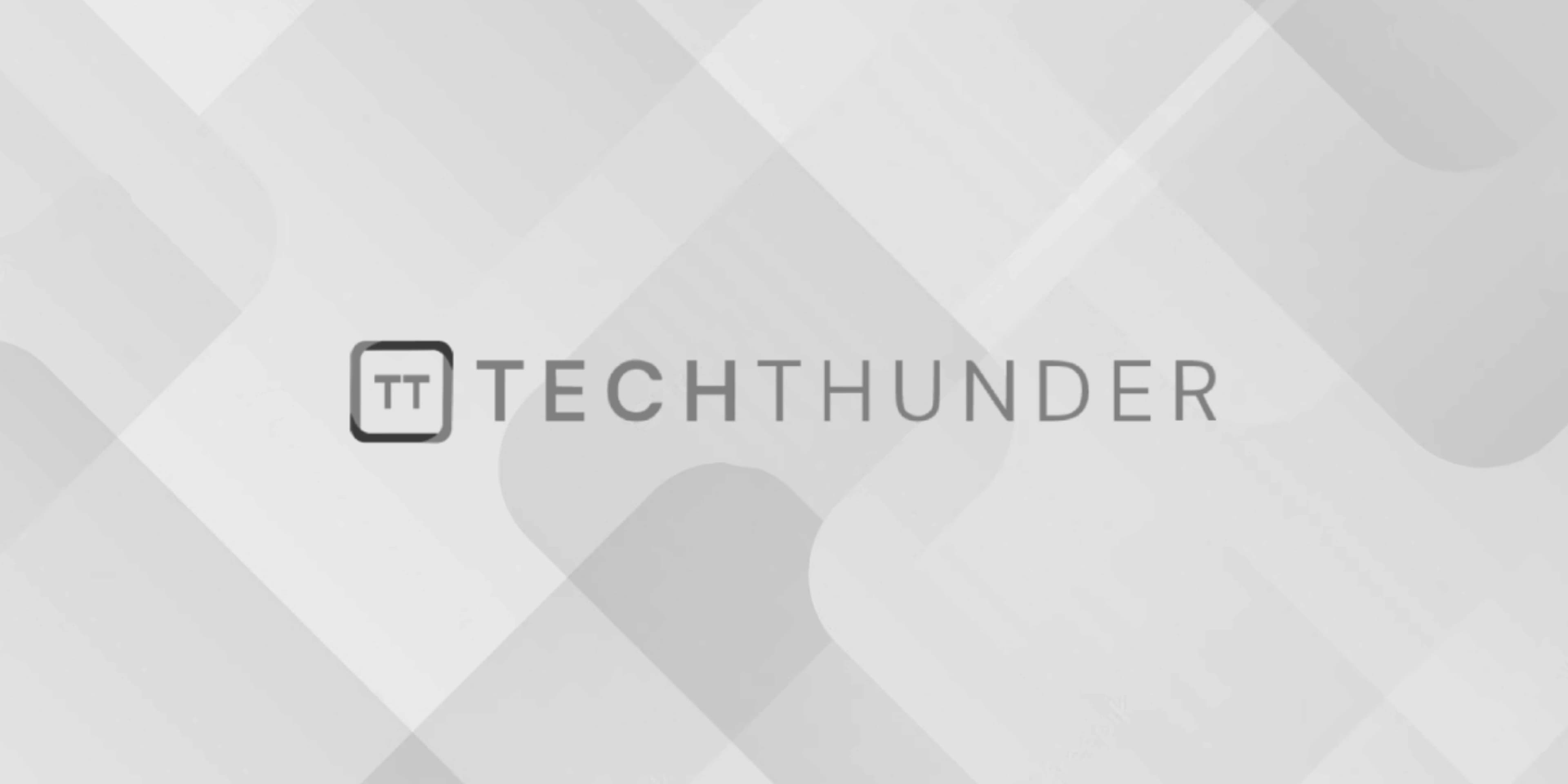
Get Current Page URL in PHP
In PHP, you can obtain the current page URL using the $_SERVER
superglobal array. The $_SERVER['REQUEST_URI']
element contains the relative URL of the current page, while the $_SERVER['HTTP_HOST']
element holds the domain name.
Here’s how you can get the current page URL in PHP:
$currentURL = 'http';
if (isset($_SERVER['HTTPS']) && $_SERVER['HTTPS'] === 'on') {
$currentURL .= 's';
}
$currentURL .= "://";
$currentURL .= $_SERVER['HTTP_HOST'];
$currentURL .= $_SERVER['REQUEST_URI'];
echo $currentURL;
Let’s break down the code:
- We check if the
$_SERVER['HTTPS']
element is set and has a value of'on'
. This indicates that the current page is accessed using HTTPS. If this condition is true, we append's'
to the URL to indicate the HTTPS protocol. - We start building the URL with the
"http"
or"https"
part, depending on whether HTTPS is used. - We append the
"://"
, the domain name from$_SERVER['HTTP_HOST']
, and the relative URL of the current page from$_SERVER['REQUEST_URI']
. - Finally, we have the complete URL stored in the variable
$currentURL
, and we echo it to display the current page’s URL.
The above code will give you the full URL of the current page, including the protocol (http or https), domain name, and the relative path. For example, the output might be something like:
https://www.example.com/myfolder/mypage.php
This method works for most scenarios, but keep in mind that in some specific server configurations or behind certain proxy setups, the $_SERVER['HTTP_HOST']
and $_SERVER['REQUEST_URI']
variables may not always contain the exact values you expect. Therefore, it’s a good idea to test the behavior on your specific server setup to ensure accurate results.