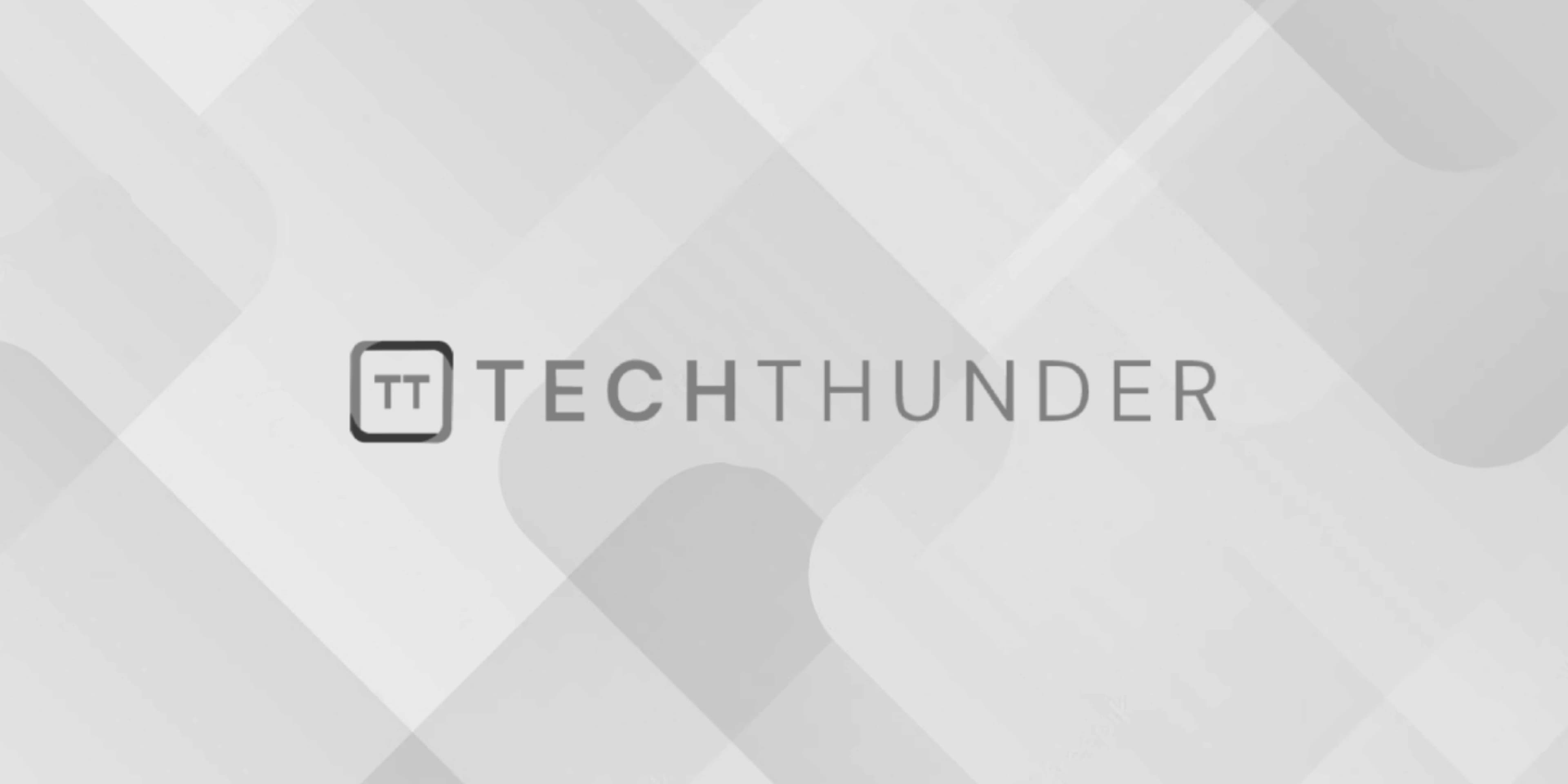
301 views
PHP Encryption
In PHP, you can perform encryption using various cryptographic algorithms to protect sensitive data, such as passwords or private information. There are two main types of encryption: symmetric encryption and asymmetric encryption.
- Symmetric Encryption:
In symmetric encryption, the same key is used for both encryption and decryption. It is typically faster than asymmetric encryption but requires secure key management since anyone with the key can decrypt the data.
Here’s a basic example of how to use symmetric encryption in PHP with the openssl_encrypt()
and openssl_decrypt()
functions:
<?php
// Data to encrypt
$data = "Sensitive information that needs to be encrypted.";
// Encryption key (should be kept secret and securely stored)
$encryptionKey = "YourEncryptionKey"; // Replace with your own strong encryption key
// Encryption algorithm
$algorithm = "AES-256-CBC"; // AES encryption with 256-bit key and CBC mode
// Initialization vector (IV) - random bytes
$iv = openssl_random_pseudo_bytes(openssl_cipher_iv_length($algorithm));
// Encrypt the data
$encryptedData = openssl_encrypt($data, $algorithm, $encryptionKey, OPENSSL_RAW_DATA, $iv);
// To store or transmit the encrypted data, you might want to encode it using base64
$encodedEncryptedData = base64_encode($iv . $encryptedData);
// Decrypt the data
$decodedEncryptedData = base64_decode($encodedEncryptedData);
$decryptedData = openssl_decrypt($decodedEncryptedData, $algorithm, $encryptionKey, OPENSSL_RAW_DATA, $iv);
echo "Original Data: " . $data . "\n";
echo "Decrypted Data: " . $decryptedData . "\n";
?>
- Asymmetric Encryption:
In asymmetric encryption, a pair of keys is used: a public key for encryption and a private key for decryption. The public key is known to everyone, while the private key is kept secret by the owner.
Here’s a basic example of how to use asymmetric encryption in PHP with the openssl_public_encrypt()
and openssl_private_decrypt()
functions:
<?php
// Data to encrypt
$data = "Sensitive information that needs to be encrypted.";
// Generate a new key pair (usually, the public key is stored and shared, while the private key is kept secure)
$config = array(
"private_key_bits" => 2048,
"private_key_type" => OPENSSL_KEYTYPE_RSA,
);
$keyPair = openssl_pkey_new($config);
openssl_pkey_export($keyPair, $privateKey);
// Get the public key from the key pair
$publicKey = openssl_pkey_get_details($keyPair)['key'];
// Encrypt the data using the public key
openssl_public_encrypt($data, $encryptedData, $publicKey);
// Decrypt the data using the private key
openssl_private_decrypt($encryptedData, $decryptedData, $privateKey);
echo "Original Data: " . $data . "\n";
echo "Decrypted Data: " . $decryptedData . "\n";
?>
Remember to handle encryption keys securely and store them in a safe place, as the security of your encrypted data relies on the strength and secrecy of the encryption keys.