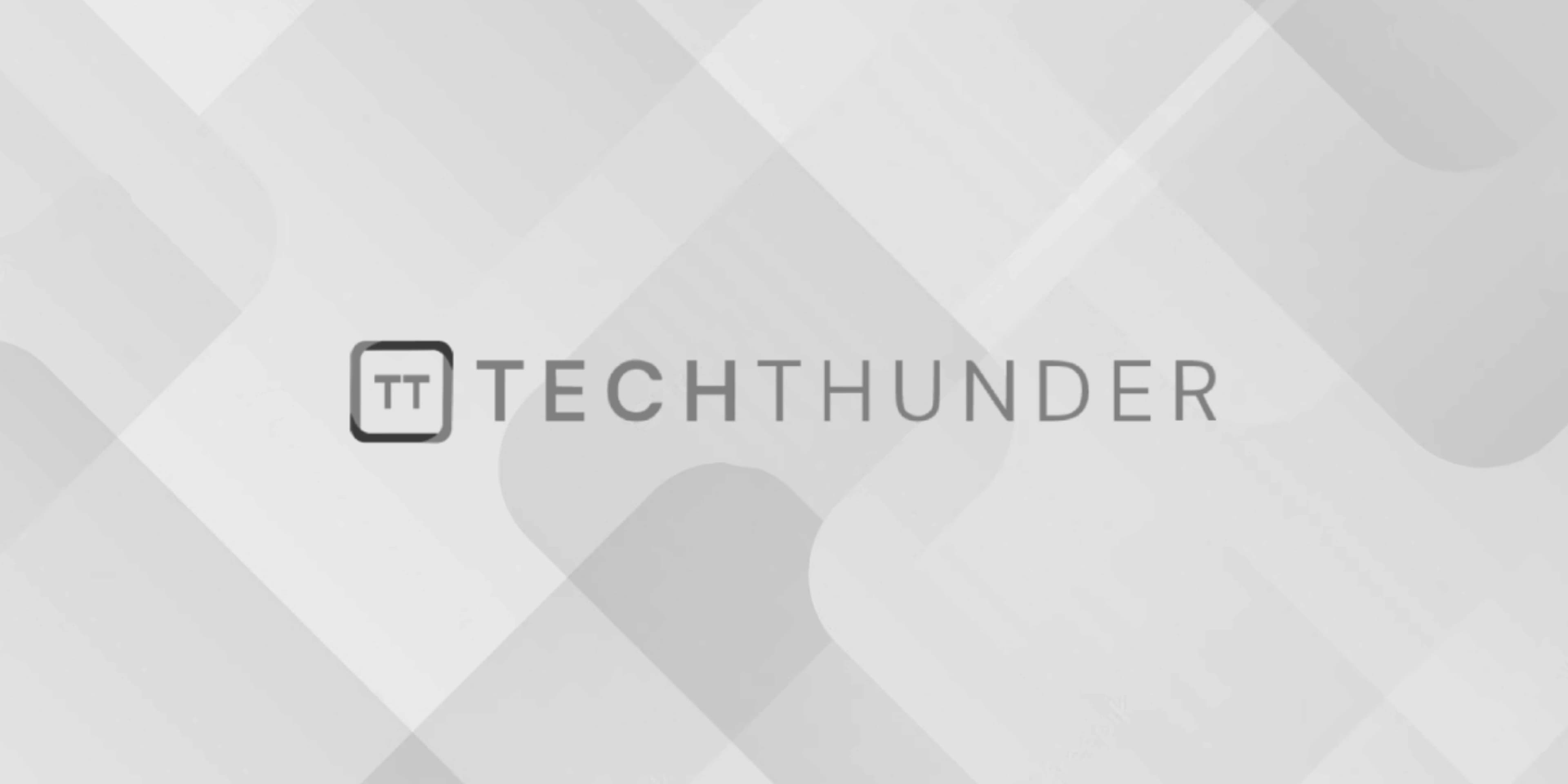
PHP include_once
In PHP, include_once
is a language construct used to include and execute a PHP file in the current script. It is similar to the include
and require
constructs, but with one key difference: include_once
ensures that the file is included only once, even if the include_once
statement is called multiple times in the script.
The general syntax of include_once
is:
include_once 'path/to/file.php';
or
include_once('path/to/file.php');
In both cases, the specified file (file.php
in this example) will be included and executed in the current PHP script if it exists and is readable. The include_once
statement will also check if the file has been included before in the current script execution. If the file has already been included, PHP will skip including it again, preventing duplication of code and potential errors that could arise from redeclaring functions or classes.
Here’s a simple example to illustrate the usage of include_once
:
file1.php
<?php
$variable = "Hello from file1.php";
?>
file2.php
<?php
// Using include_once to include file1.php
include_once 'file1.php';
echo $variable; // Output: Hello from file1.php
?>
In this example, when file2.php
is executed, it includes file1.php
using include_once
. The variable $variable
defined in file1.php
is accessible in file2.php
, and it will output “Hello from file1.php.”
By using include_once
, you can ensure that you don’t accidentally include the same file multiple times, which can help you avoid issues related to redefining functions or classes and improve code organization and maintainability.