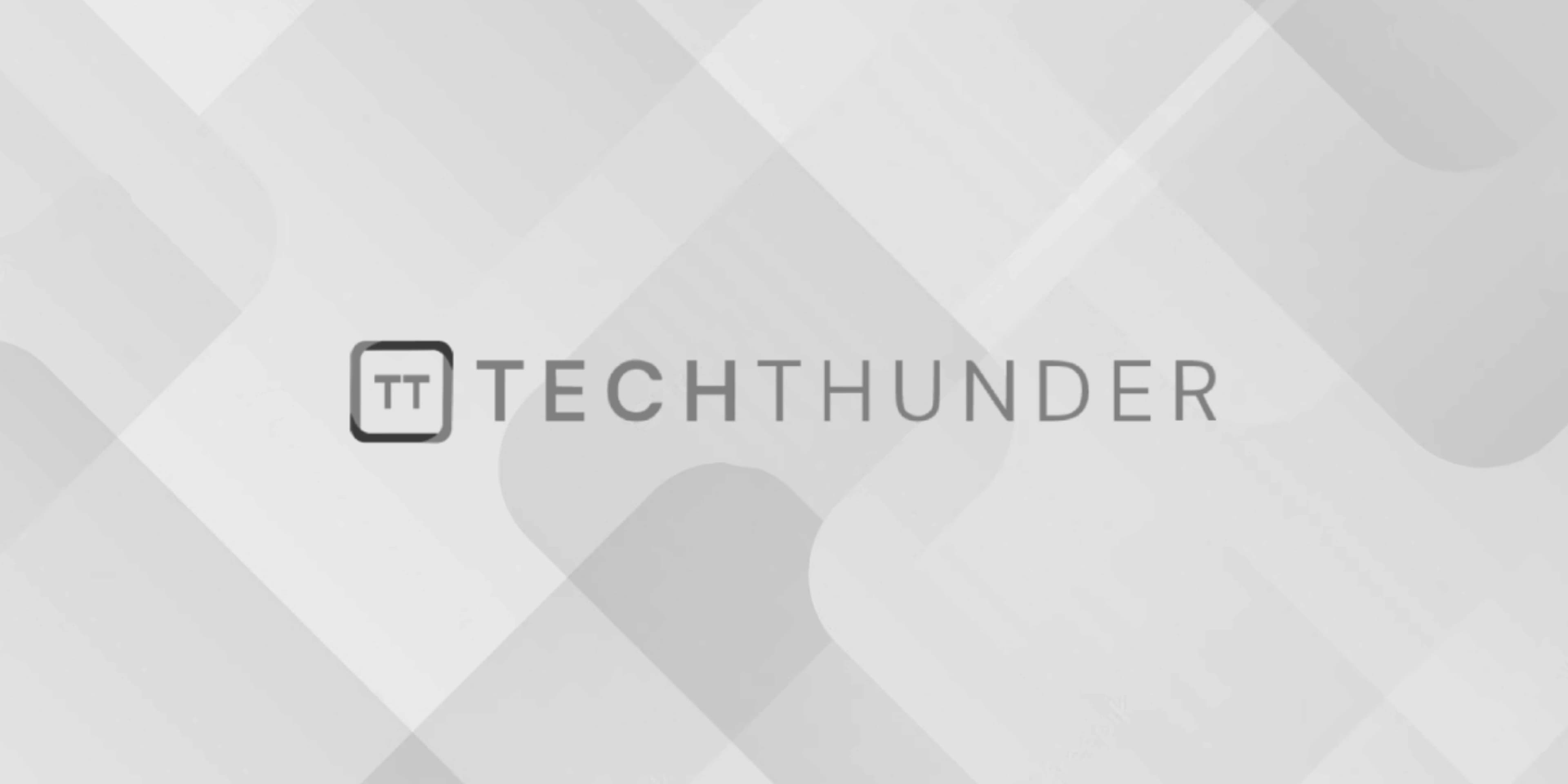
PHP Image createtruecolor( ) Function
In PHP, imagecreatetruecolor()
is a function used to create a new true-color image resource with a specified width and height. This function is part of the GD (GIF Draw) library, which is commonly used in PHP for creating and manipulating images.
A true-color image is an image that stores colors directly as RGB values, allowing for a higher color depth and more accurate representation of colors compared to indexed color images.
Here’s the syntax of the imagecreatetruecolor()
function:
resource imagecreatetruecolor(int $width, int $height)
Parameters:
$width
: The width of the new image in pixels.$height
: The height of the new image in pixels.
Return value:
- The
imagecreatetruecolor()
function returns a GD true-color image resource, which is a special type of resource that represents the newly created image. This image resource is used to perform various image manipulation operations using GD functions.
Example:
// Create a new true-color image with width 400 pixels and height 200 pixels
$imageWidth = 400;
$imageHeight = 200;
$image = imagecreatetruecolor($imageWidth, $imageHeight);
// Allocate colors for the background (white) and a rectangle (blue)
$backgroundColor = imagecolorallocate($image, 255, 255, 255); // White
$rectangleColor = imagecolorallocate($image, 0, 0, 255); // Blue
// Set the background color
imagefill($image, 0, 0, $backgroundColor);
// Draw a blue rectangle on the image
imagefilledrectangle($image, 50, 50, 350, 150, $rectangleColor);
// Set the content type header to output the image as PNG
header('Content-Type: image/png');
// Output the image as PNG to the browser
imagepng($image);
// Free up memory by destroying the image resource
imagedestroy($image);
In this example, we use imagecreatetruecolor()
to create a new true-color image with a width of 400 pixels and a height of 200 pixels. We then allocate two colors for the image: $backgroundColor
for the background (white) and $rectangleColor
for the blue rectangle. We set the background color for the entire image using imagefill()
and draw a blue rectangle on the image using imagefilledrectangle()
. Finally, we output the image as PNG to the browser using imagepng()
and free up memory by destroying the image resource with imagedestroy()
.
True-color images are generally recommended for higher-quality graphics and photographs because they provide a wider range of colors and more accurate color representation compared to indexed color images. However, true-color images consume more memory and may have larger file sizes compared to indexed color images.