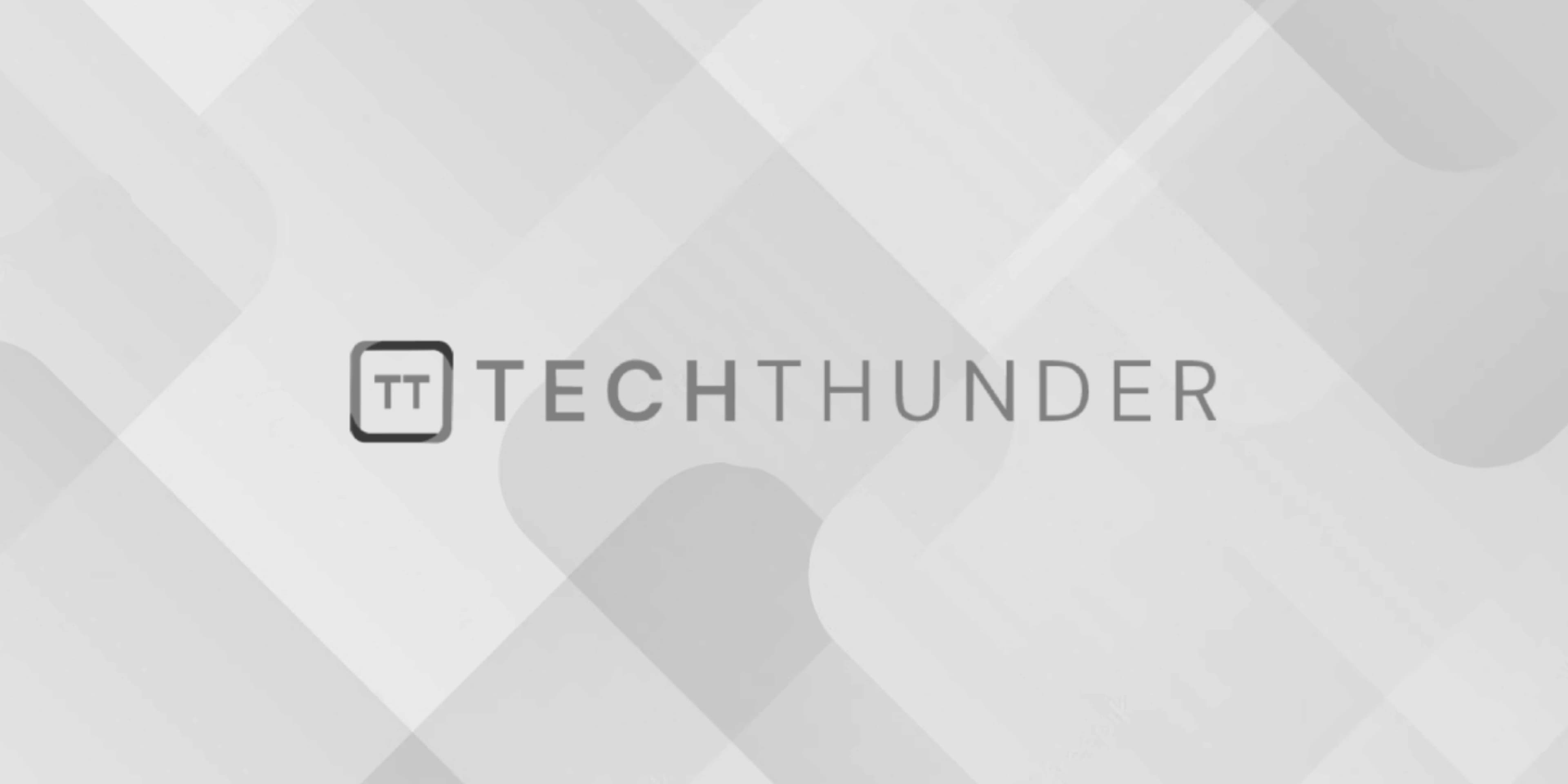
OOPs Overloading
In object-oriented programming (OOP), method overloading refers to the ability of a class to define multiple methods with the same name but with different parameter lists. Each method performs a different operation based on the number or types of arguments it receives. The method overloading feature allows a class to have multiple implementations of a method, making the code more flexible and expressive.
Method overloading is useful when you want to provide different ways of calling a method, depending on the type or number of arguments provided, without the need to give each method a unique name.
Let’s consider an example in TypeScript:
class MathOperations {
add(x: number, y: number): number {
return x + y;
}
add(x: number, y: number, z: number): number {
return x + y + z;
}
}
In this example, the MathOperations
class has two add
methods: one that takes two arguments and returns their sum, and another that takes three arguments and returns their sum. The methods have the same name (add
), but they have different parameter lists, allowing the class to handle addition with two or three arguments differently.
When you call the add
method, the TypeScript compiler will determine which version of the method to invoke based on the number and types of arguments you provide:
const math = new MathOperations();
console.log(math.add(2, 3)); // Calls the first add method and prints 5
console.log(math.add(2, 3, 4)); // Calls the second add method and prints 9
Method overloading is not limited to TypeScript; it is a feature supported by many object-oriented programming languages like Java and C#. In these languages, method overloading works similarly to the TypeScript example provided above.
Keep in mind that method overloading is based on the number and types of arguments, not on the return type of the methods. If you define multiple methods with the same name and parameter lists but with different return types, it will result in a compilation error.