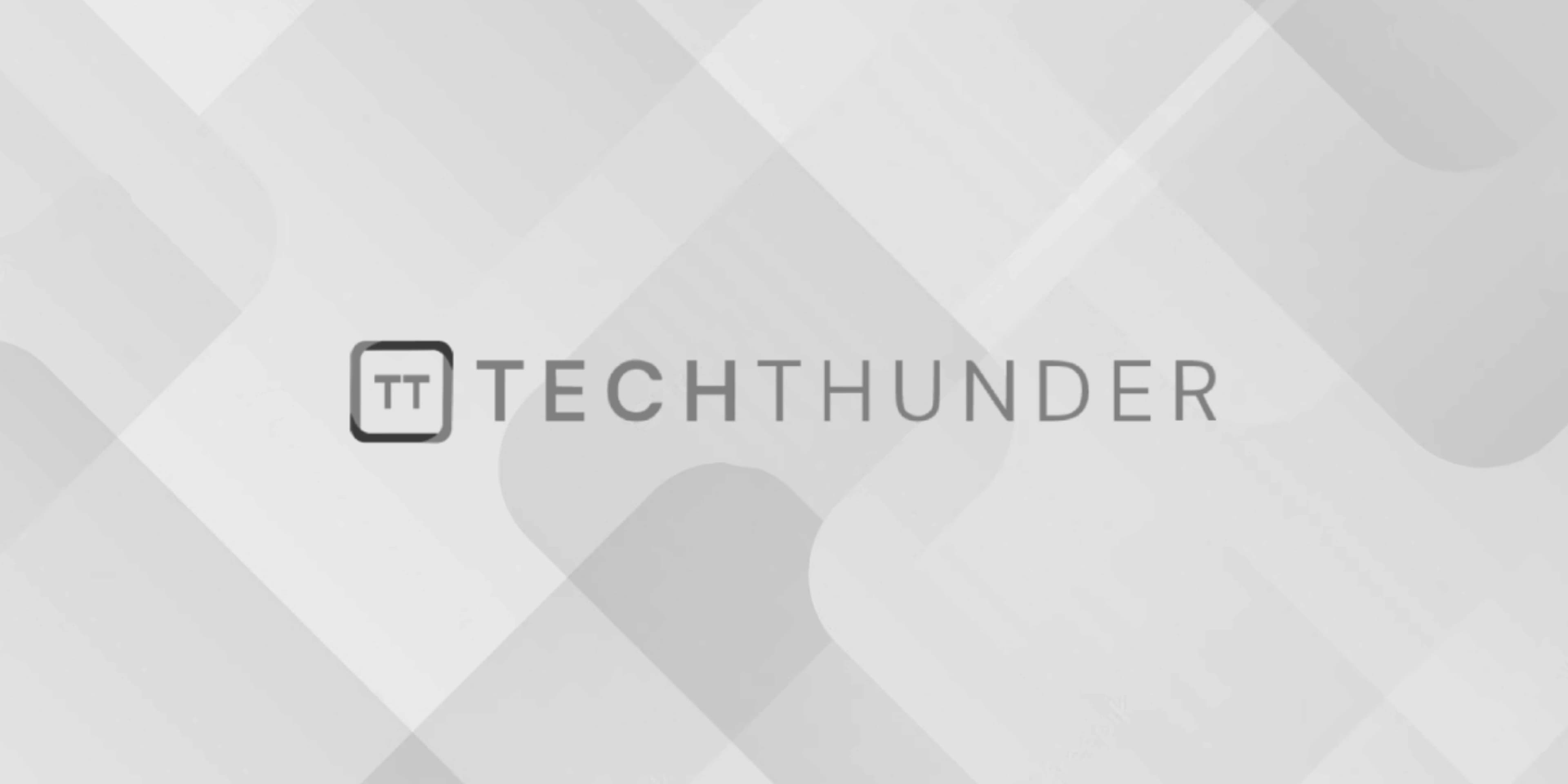
PHP MySQLi Order by
In PHP, you can use MySQLi (MySQL Improved) to perform a SELECT operation with the ORDER BY clause to sort the results of your query based on one or more columns in ascending or descending order. The ORDER BY clause is often used in combination with the SELECT query to arrange the retrieved data in a specific order.
Here’s how you can use ORDER BY with MySQLi in PHP:
- Connect to the MySQL Database:
First, establish a connection to the MySQL database using themysqli_connect()
function, as shown in the previous examples.
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database";
// Create a connection
$conn = mysqli_connect($servername, $username, $password, $dbname);
// Check the connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>
- Prepare and Execute the SELECT Query with ORDER BY:
Next, prepare and execute a SELECT query with the ORDER BY clause to retrieve data from the database table and sort it as needed. Use themysqli_query()
function to execute the query and obtain a result set.
<?php
// Prepare the SELECT query with ORDER BY
$sql = "SELECT id, name, email FROM users ORDER BY name ASC";
// Execute the query
$result = mysqli_query($conn, $sql);
// Check if the query was successful
if ($result) {
// Fetch the data from the result set
while ($row = mysqli_fetch_assoc($result)) {
echo "ID: " . $row['id'] . ", Name: " . $row['name'] . ", Email: " . $row['email'] . "<br>";
}
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
?>
In this example, we are retrieving data from the “users” table and sorting it based on the “name” column in ascending order (ASC). To sort in descending order, you can use the DESC keyword.
- Close the Database Connection:
After retrieving and displaying the data, close the database connection using themysqli_close()
function.
<?php
// Close the connection
mysqli_close($conn);
?>
Remember to use proper data sanitization and validation techniques to prevent SQL injection and other security issues, especially when building dynamic SQL queries with user input.
Also, keep in mind that ORDER BY can be used with multiple columns to create more complex sorting criteria. For example:
$sql = "SELECT id, name, email FROM users ORDER BY name ASC, email DESC";
This query would first sort the data by “name” in ascending order and then sort records with the same name by “email” in descending order.