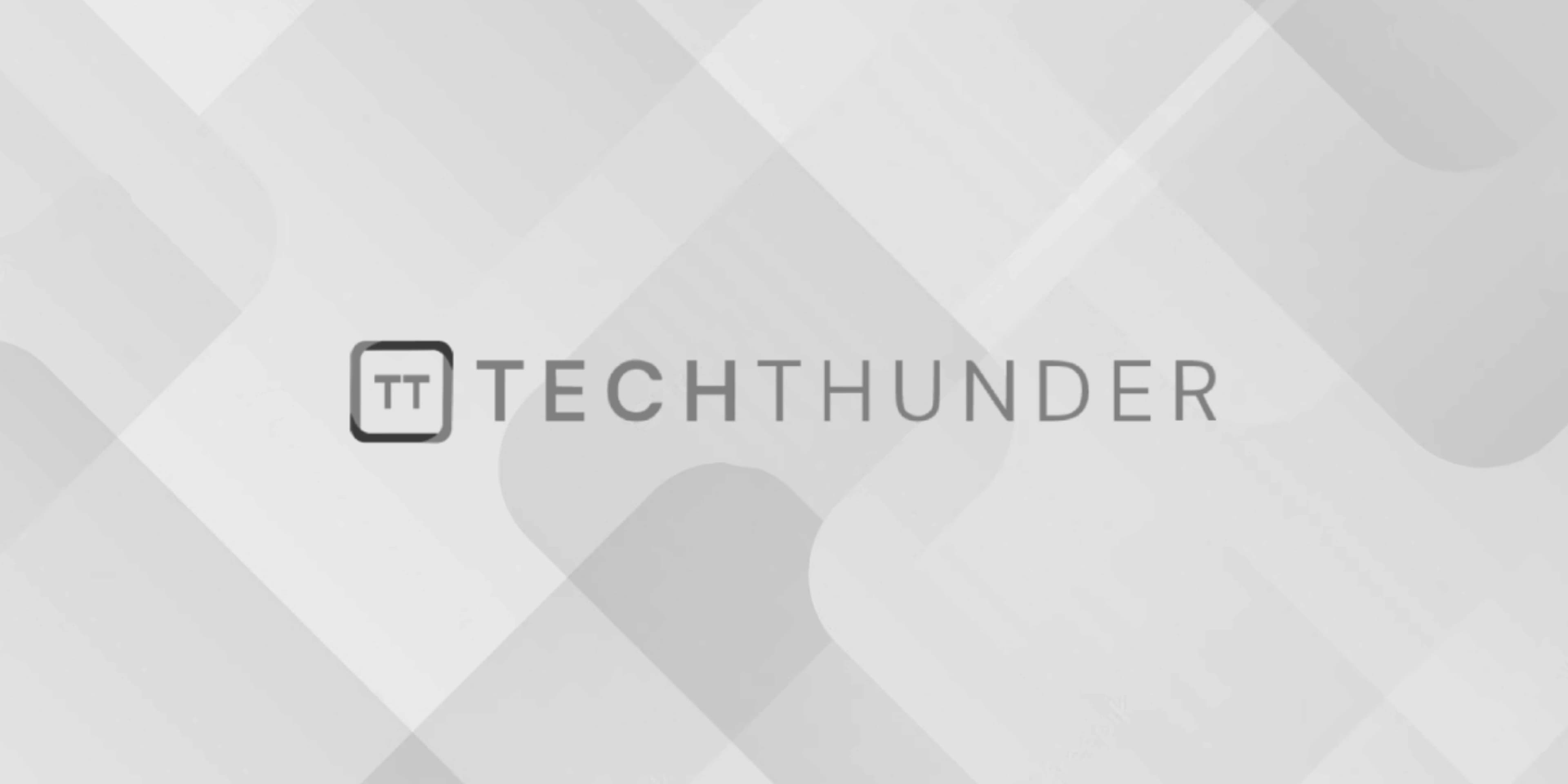
PHP Dropzone File Upload on Button Click
Dropzone is a popular JavaScript library for handling file uploads with drag-and-drop functionality. To trigger file uploads on a button click, you can integrate Dropzone with a simple HTML button and JavaScript event handling. Below is an example of how to achieve this using PHP, Dropzone.js, and JavaScript:
- Include the Required Libraries:
Download the Dropzone.js library or include it via CDN. Also, include the Dropzone CSS and any other required JavaScript libraries, such as jQuery.
<!DOCTYPE html>
<html>
<head>
<title>Dropzone File Upload</title>
<!-- Include Dropzone CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.9.2/min/dropzone.min.css">
</head>
<body>
<h2>File Upload</h2>
<button id="uploadButton">Upload File</button>
<!-- Include Dropzone.js and other JavaScript libraries -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.9.2/min/dropzone.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</body>
</html>
- Initialize Dropzone on Button Click:
Create a JavaScript function that will initialize the Dropzone on the button click event. We’ll use theclick()
function from jQuery to trigger the Dropzone when the button is clicked.
<script>
$(document).ready(function() {
$("#uploadButton").click(function() {
// Create a Dropzone instance on the button click
var myDropzone = new Dropzone("#uploadButton", {
url: "upload.php", // PHP script for handling file upload
acceptedFiles: ".jpg, .jpeg, .png", // Specify the allowed file types
maxFiles: 1, // Limit the number of files to be uploaded
maxFilesize: 5, // Limit the file size (in MB)
dictDefaultMessage: "Drop your files here or click to upload",
success: function(file, response) {
// Handle the success response (if needed)
console.log("File uploaded successfully!");
},
error: function(file, response) {
// Handle the error response (if needed)
console.error("Error uploading file: " + response);
}
});
});
});
</script>
- Create the PHP Upload Script (upload.php):
Create a PHP script (upload.php) to handle the file upload and save the file on the server. This script will be called by Dropzone when a file is uploaded.
<?php
if (isset($_FILES['file'])) {
// Set your desired upload directory
$uploadDir = "uploads/";
$file = $_FILES['file'];
$fileName = $file['name'];
$filePath = $uploadDir . $fileName;
if (move_uploaded_file($file['tmp_name'], $filePath)) {
echo "File uploaded successfully.";
} else {
echo "Error uploading file.";
}
}
?>
In this example, when you click the “Upload File” button, it will trigger Dropzone, and you can drag and drop a file onto the button or click the button to open the file selection dialog. The selected file will be uploaded to the server using the PHP script (upload.php), and you will see the success or error messages accordingly.
Make sure you have appropriate permissions set on the server to allow file uploads and ensure that you sanitize and validate user input to prevent security issues. Additionally, consider implementing server-side checks for file size, file type, and other validations based on your requirements.