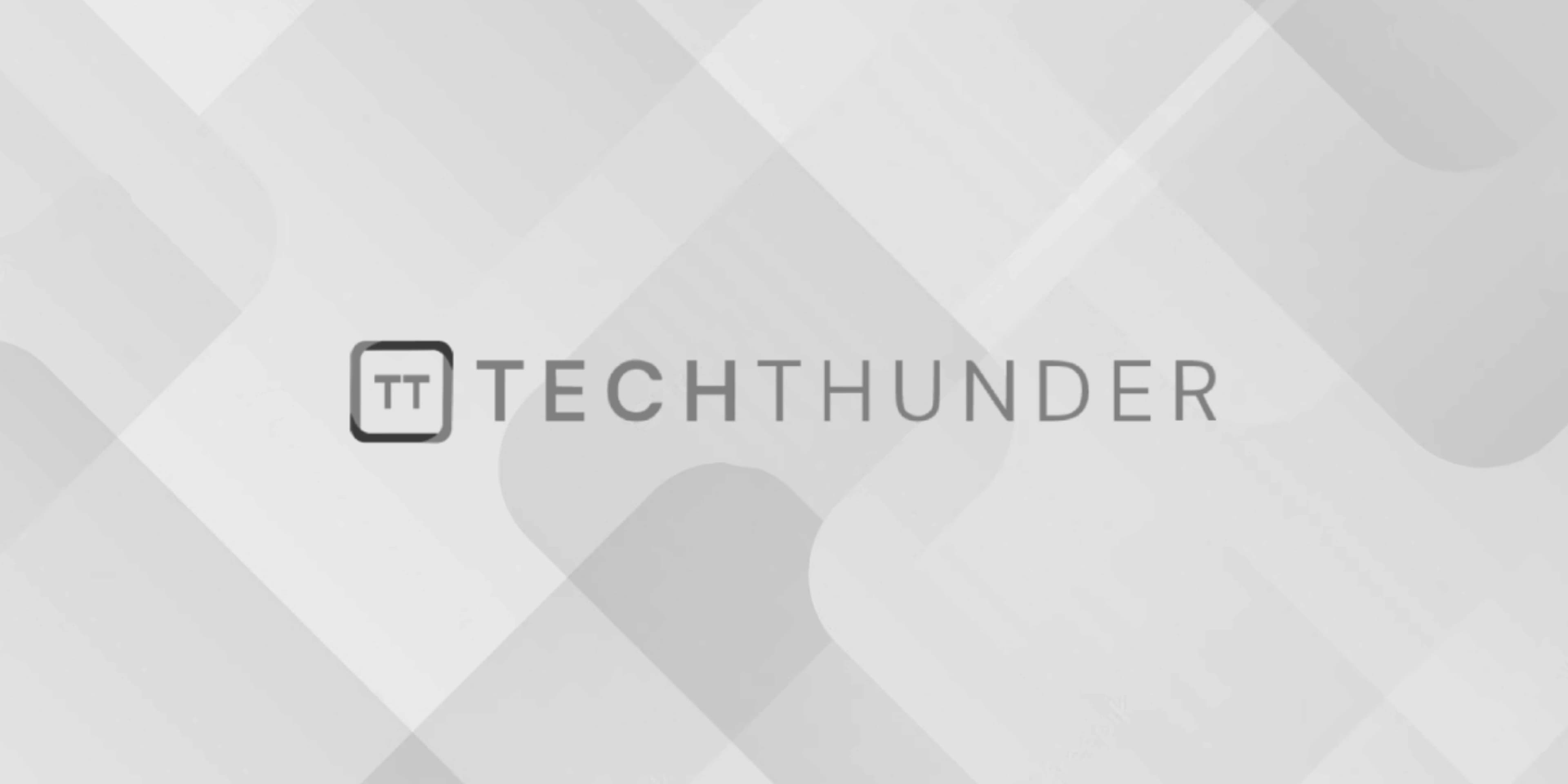
Get IP Address in PHP
In PHP, you can obtain the IP address of a client accessing your web server using the $_SERVER
superglobal array. The $_SERVER['REMOTE_ADDR']
element contains the client’s IP address.
Here’s how you can get the client’s IP address in PHP:
$clientIP = $_SERVER['REMOTE_ADDR'];
echo "Client IP Address: " . $clientIP;
The $_SERVER['REMOTE_ADDR']
variable contains the IP address of the client making the current request. Keep in mind that the REMOTE_ADDR
value may not always provide the actual client IP address directly, especially if your server is behind a proxy or load balancer. In such cases, the IP address of the proxy or load balancer may be provided instead.
To handle this situation and get the correct client IP address in cases of proxying, you can check other server variables, such as HTTP_X_FORWARDED_FOR
or HTTP_CLIENT_IP
. However, it’s essential to note that these values can be easily spoofed by clients, so you should use them with caution and only when you trust the proxy server’s configurations.
Here’s an example of getting the client’s IP address considering proxy configurations:
if (isset($_SERVER['HTTP_X_FORWARDED_FOR'])) {
$clientIP = $_SERVER['HTTP_X_FORWARDED_FOR'];
} elseif (isset($_SERVER['HTTP_CLIENT_IP'])) {
$clientIP = $_SERVER['HTTP_CLIENT_IP'];
} else {
$clientIP = $_SERVER['REMOTE_ADDR'];
}
echo "Client IP Address: " . $clientIP;
Using this code, the script checks if HTTP_X_FORWARDED_FOR
or HTTP_CLIENT_IP
server variables are set, and if they are, it considers them as the client’s IP address. Otherwise, it falls back to using REMOTE_ADDR
. This approach accounts for possible proxy or load balancer configurations.
Keep in mind that in some scenarios, the server configurations may prevent access to certain server variables, and the client’s IP address may not be retrievable through these methods. Always test the behavior on your specific server setup to ensure accurate results.