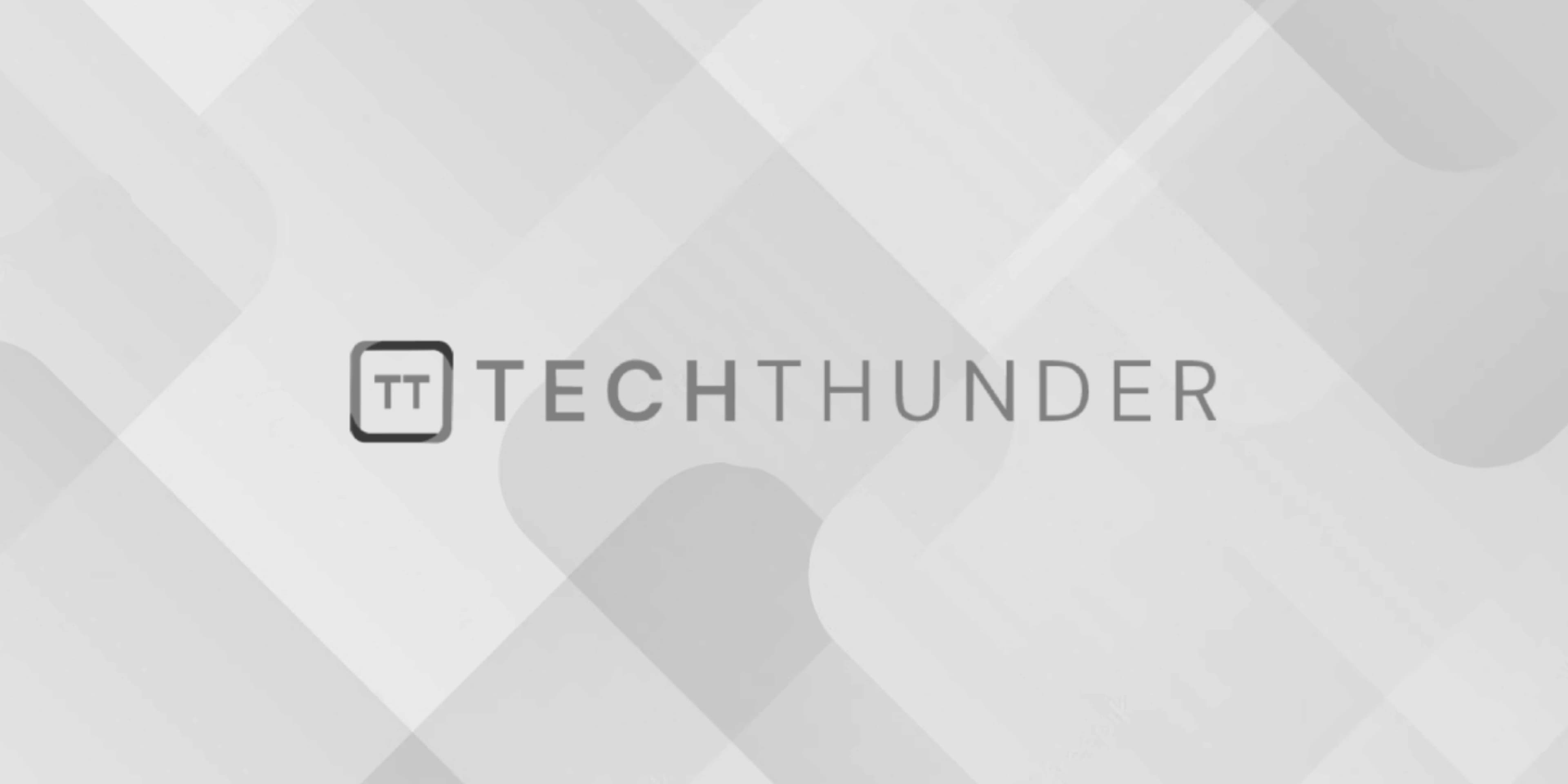
Types of Errors in PHP
In PHP, errors can occur during the execution of a script due to various reasons. These errors can be classified into different types based on their severity and impact on the script’s execution. The three main types of errors in PHP are:
- Syntax Errors:
Syntax errors occur when there are mistakes in the PHP code’s syntax, preventing the script from being executed. These errors are usually detected during the parsing phase before the script is executed. Common syntax errors include missing or mismatched parentheses, braces, or quotes, and typographical errors.
Example of a syntax error:
echo "Hello, World!;
Output:
Parse error: syntax error, unexpected ';', expecting ',' or ';' in script.php on line 2
- Runtime Errors:
Runtime errors occur during the execution of the PHP script. These errors are caused by issues such as attempting to divide by zero, accessing undefined variables or array indexes, using undefined functions or methods, or calling a function with the wrong number of arguments.
Example of a runtime error:
$number = 10;
$result = $number / 0;
Output:
Warning: Division by zero in script.php on line 2
- Logical Errors (or Semantic Errors):
Logical errors, also known as semantic errors, occur when the PHP script runs without any syntax or runtime errors, but it does not produce the expected or desired output. These errors can be more challenging to detect as the script technically runs without any error messages, but it may not function correctly due to incorrect logic or algorithm implementation.
Example of a logical error:
$price = 100;
$discount = 20;
$totalPrice = $price - $discount; // Incorrect calculation
echo "Total Price: $totalPrice"; // Output: Total Price: 80
In the above example, the logical error is that the discount is subtracted from the price instead of being calculated as a percentage of the price. As a result, the total price is incorrect.
Handling Errors:
To handle errors in PHP, you can use error handling functions such as error_reporting()
, ini_set()
, and set_error_handler()
to control error reporting levels, log errors, and handle errors with custom functions.
It is essential to handle errors properly in PHP to ensure the smooth functioning of your scripts and to provide meaningful error messages to users or log them for debugging purposes.