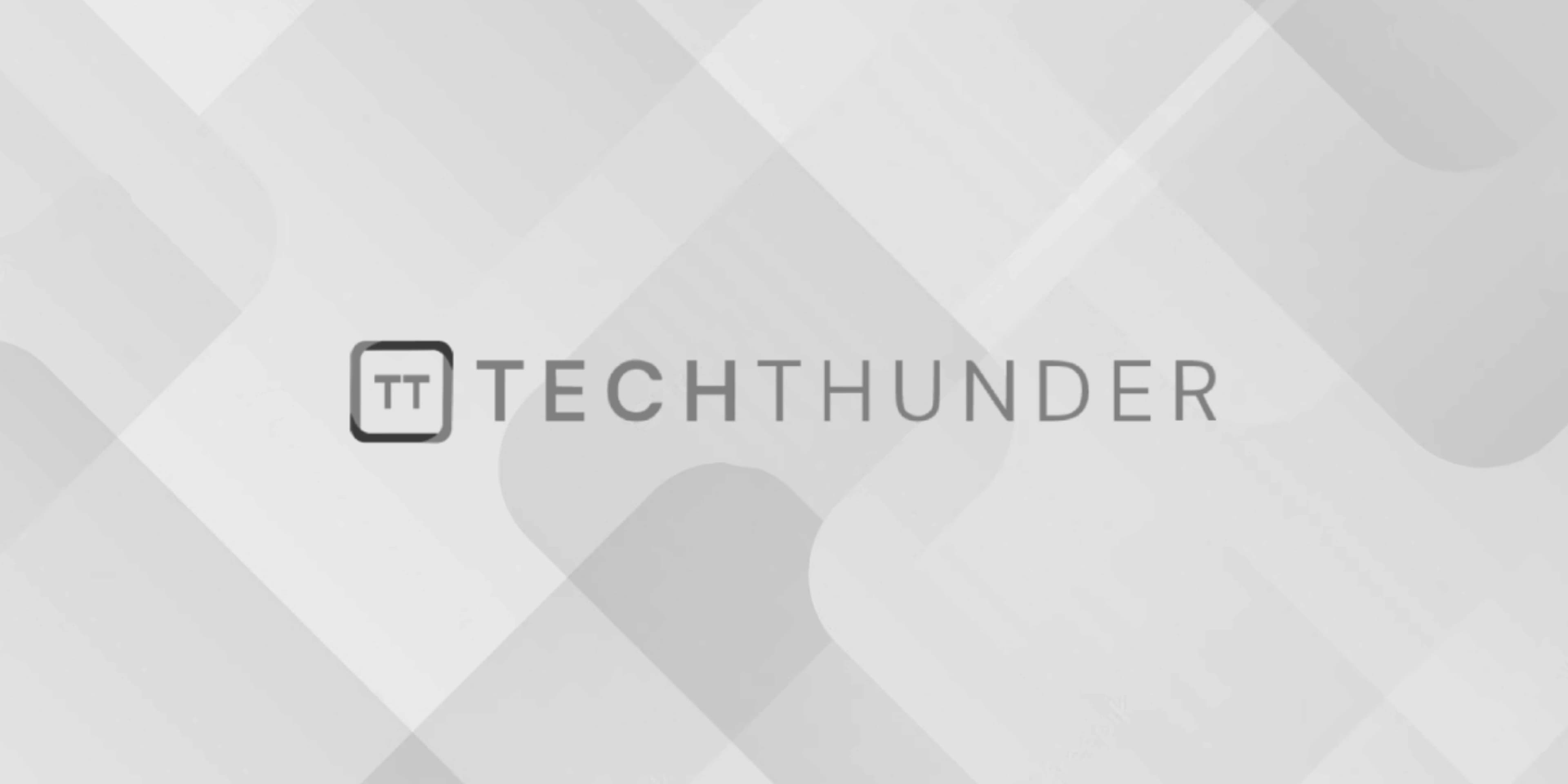
Multiple File Upload using Dropzone JS in PHP
To implement multiple file upload using Dropzone JS in PHP, you need to follow these steps:
- Set Up Dropzone JS and HTML Form:
Include the Dropzone JS library and create an HTML form with the appropriate attributes for the file upload.
<!DOCTYPE html>
<html>
<head>
<title>Multiple File Upload using Dropzone JS</title>
<!-- Include Dropzone CSS -->
<link rel="stylesheet" href="path/to/dropzone.min.css">
</head>
<body>
<h2>Multiple File Upload</h2>
<!-- Dropzone area -->
<form action="upload.php" class="dropzone" id="myDropzone">
<!-- You can add other form fields here if needed -->
</form>
<!-- Include Dropzone JS -->
<script src="path/to/dropzone.min.js"></script>
</body>
</html>
- Create Upload PHP Script (upload.php):
Create a PHP script (upload.php) to handle the file upload and move the uploaded files to the desired directory.
<?php
// Set your upload directory
$uploadDir = "uploads/";
// Check if the upload directory exists, and create it if not
if (!file_exists($uploadDir)) {
mkdir($uploadDir, 0777, true);
}
// Handle the file upload
if (!empty($_FILES)) {
$totalFiles = count($_FILES['file']['name']);
for ($i = 0; $i < $totalFiles; $i++) {
$tmpFilePath = $_FILES['file']['tmp_name'][$i];
$newFilePath = $uploadDir . $_FILES['file']['name'][$i];
// Move the uploaded file to the destination directory
if (move_uploaded_file($tmpFilePath, $newFilePath)) {
// File uploaded successfully
} else {
// Error uploading the file
}
}
}
?>
- Initialize Dropzone JS:
Use Dropzone JS to initialize the file upload process and configure its settings.
<!-- Add the following script below the previous <script> tag -->
<script>
Dropzone.autoDiscover = false; // Disable Dropzone auto-discovery to prevent conflicts
// Initialize Dropzone
$(document).ready(function() {
$("#myDropzone").dropzone({
url: "upload.php", // PHP script for handling file upload
paramName: "file", // Name of the file input field
maxFilesize: 5, // Maximum file size in MB
maxFiles: 5, // Maximum number of files to upload
acceptedFiles: ".jpg, .jpeg, .png", // Allowed file types
dictDefaultMessage: "Drop files here or click to upload",
success: function(file, response) {
// Handle the success response (if needed)
console.log("File uploaded successfully!");
},
error: function(file, response) {
// Handle the error response (if needed)
console.error("Error uploading file: " + response);
}
});
});
</script>
Now, when you access the HTML page with the form, you will see the Dropzone area. You can drag and drop multiple files into the Dropzone or click the area to open the file selection dialog. The uploaded files will be handled by the PHP script (upload.php) and moved to the specified upload directory.
Adjust the settings and PHP script to suit your specific requirements, such as the allowed file types, maximum file size, or the destination upload directory.
Make sure the upload directory has the appropriate permissions to allow file uploads. For security reasons, you should also consider adding further validation and file type checks on the server-side before saving the uploaded files.