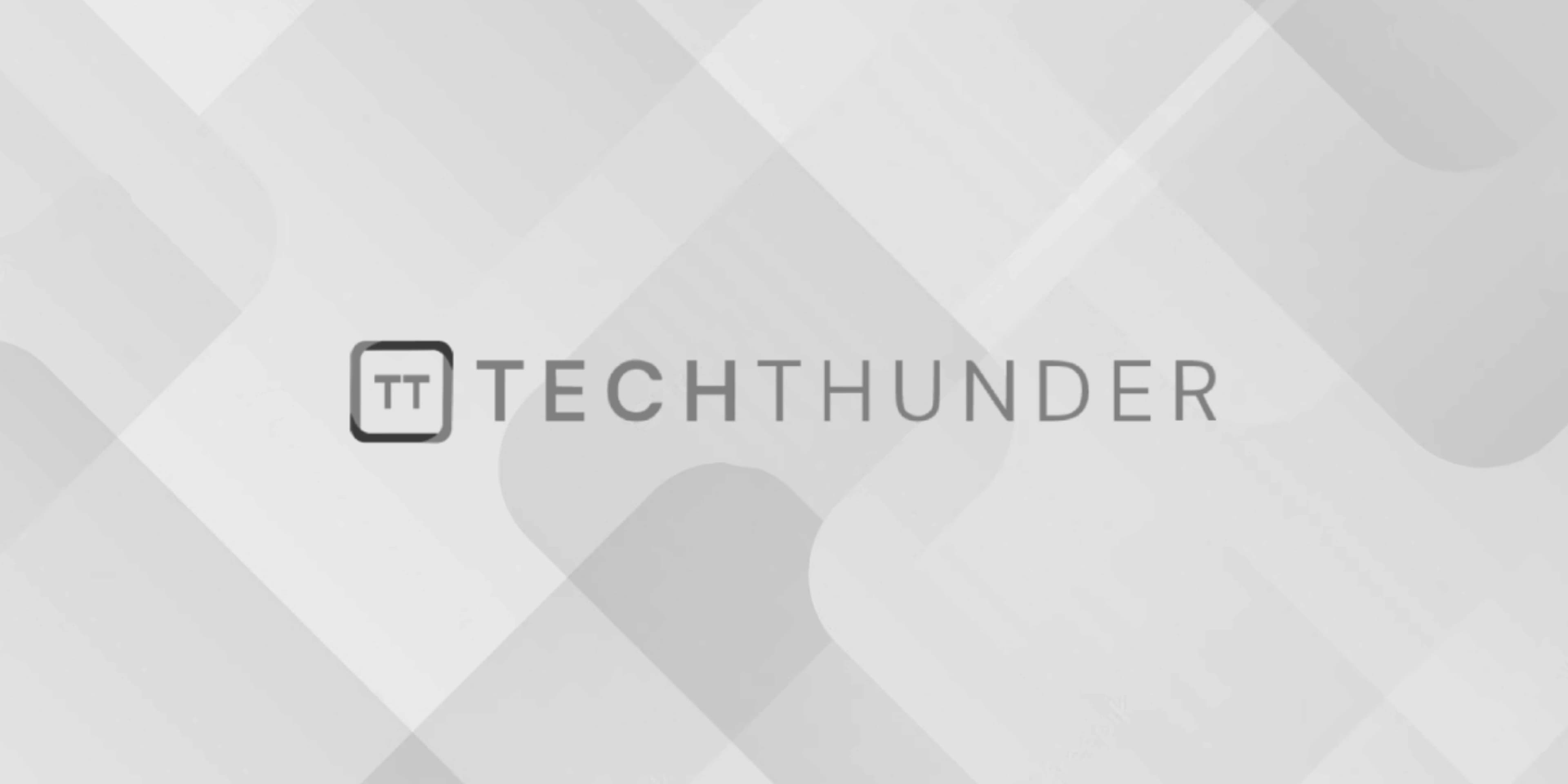
199 views
PHP Multidimensional Array
In PHP, a multidimensional array is an array that contains other arrays as its elements. In other words, each element of a multidimensional array is itself an array, creating a nested or hierarchical structure. This allows you to organize data in a more complex way, making it easier to represent and work with data that has multiple dimensions or levels.
Here’s how you can create and work with multidimensional arrays in PHP:
- Creating a Multidimensional Array:
You can create a multidimensional array by nesting arrays inside one another.
Example:
// Creating a two-dimensional array
$students = array(
array("name" => "John Doe", "age" => 25, "gender" => "Male"),
array("name" => "Jane Smith", "age" => 22, "gender" => "Female"),
array("name" => "Bob Johnson", "age" => 28, "gender" => "Male")
);
- Accessing Elements of a Multidimensional Array:
To access elements of a multidimensional array, you need to specify both the outer array index and the inner array index (the key) to retrieve the value.
Example:
echo $students[0]["name"]; // Output: John Doe
echo $students[1]["age"]; // Output: 22
- Modifying Elements of a Multidimensional Array:
You can modify elements of a multidimensional array in the same way as you would with a regular array.
Example:
$students[2]["age"] = 29;
echo $students[2]["age"]; // Output: 29
- Adding Elements to a Multidimensional Array:
You can add new key-value pairs to a multidimensional array using the square bracket notation.
Example:
$students[0]["location"] = "New York";
- Looping through a Multidimensional Array:
To iterate through the elements of a multidimensional array, you need to use nested loops, such asforeach
loops.
Example:
foreach ($students as $student) {
foreach ($student as $key => $value) {
echo $key . ": " . $value . "\n";
}
echo "\n";
}
Output:
name: John Doe
age: 25
gender: Male
name: Jane Smith
age: 22
gender: Female
name: Bob Johnson
age: 29
gender: Male
Multidimensional arrays are helpful for representing data that has multiple levels of hierarchy or dimensions, such as tables or matrices. They are commonly used when working with datasets or handling data structures that require nested organization.