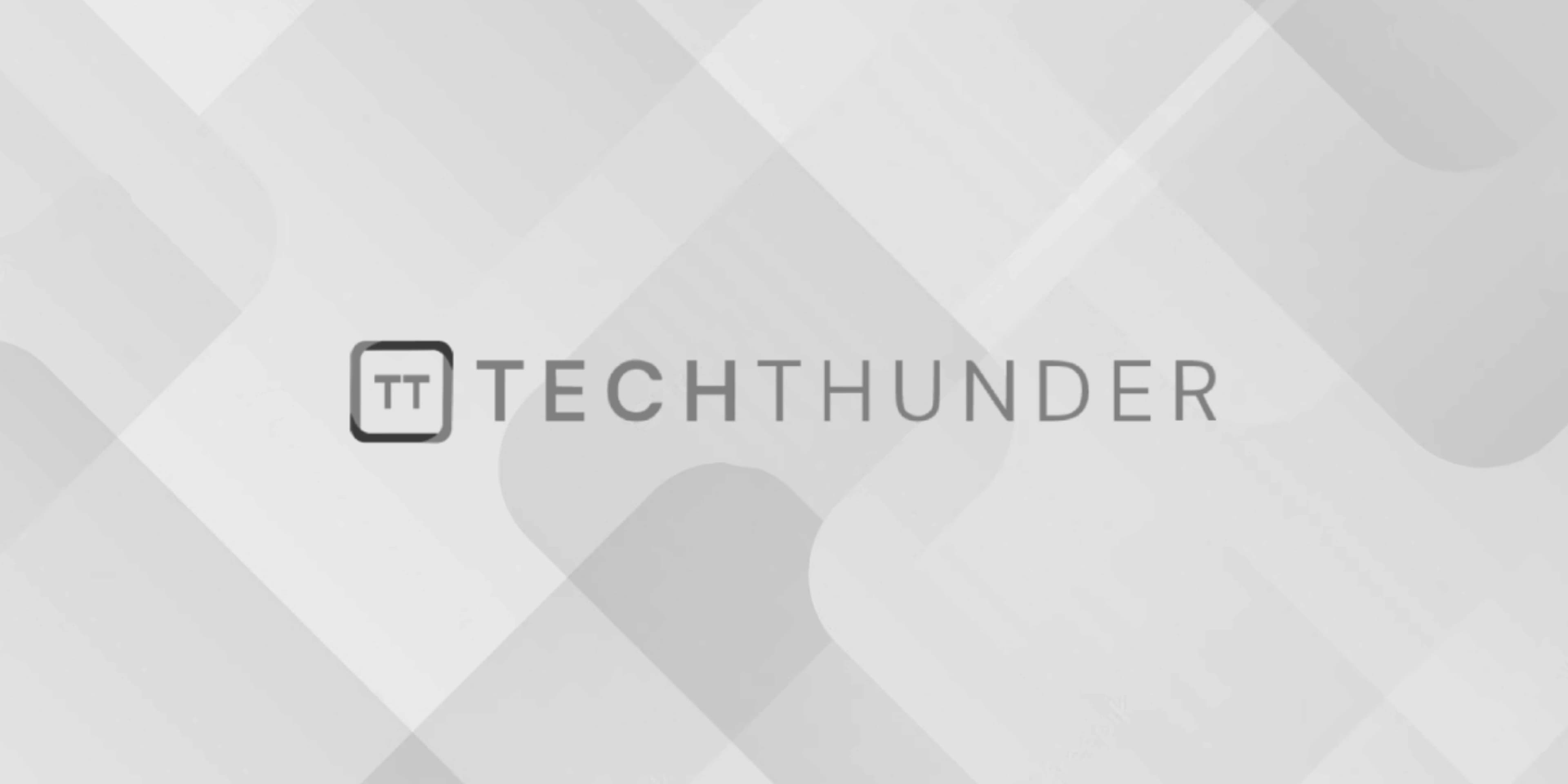
PHP Variable
In PHP, a variable is a container used to store data, such as numbers, strings, arrays, or objects. Variables are an essential part of any programming language and allow developers to work with data dynamically and manipulate it during program execution.
In PHP, you can create a variable by using the dollar sign ($
) followed by the variable name. The variable name must start with a letter or underscore and can be followed by letters, numbers, or underscores. PHP variables are case-sensitive, so $variable
, $Variable
, and $VARIABLE
are considered different variables.
Here’s the basic syntax for creating and assigning values to variables:
$variableName = value;
Example of creating and using variables:
$name = "John";
$age = 30;
$height = 6.1;
$isStudent = true;
echo "Name: " . $name . "<br>";
echo "Age: " . $age . "<br>";
echo "Height: " . $height . "<br>";
echo "Is Student: " . ($isStudent ? "Yes" : "No") . "<br>";
Output:
Name: John
Age: 30
Height: 6.1
Is Student: Yes
In this example, we create four variables: $name
, $age
, $height
, and $isStudent
, and assign different types of data to them. We then use echo
to display the values of these variables.
You can change the value of a variable at any time during the execution of the script:
$counter = 1;
$counter = $counter + 1;
echo $counter; // Output: 2
In PHP, variables are dynamically typed, which means you do not need to declare the data type of the variable explicitly. The data type is determined automatically based on the value assigned to the variable. This makes PHP a loosely typed language.
Variables play a fundamental role in PHP programming, allowing you to store, manipulate, and access data throughout the execution of your PHP scripts.