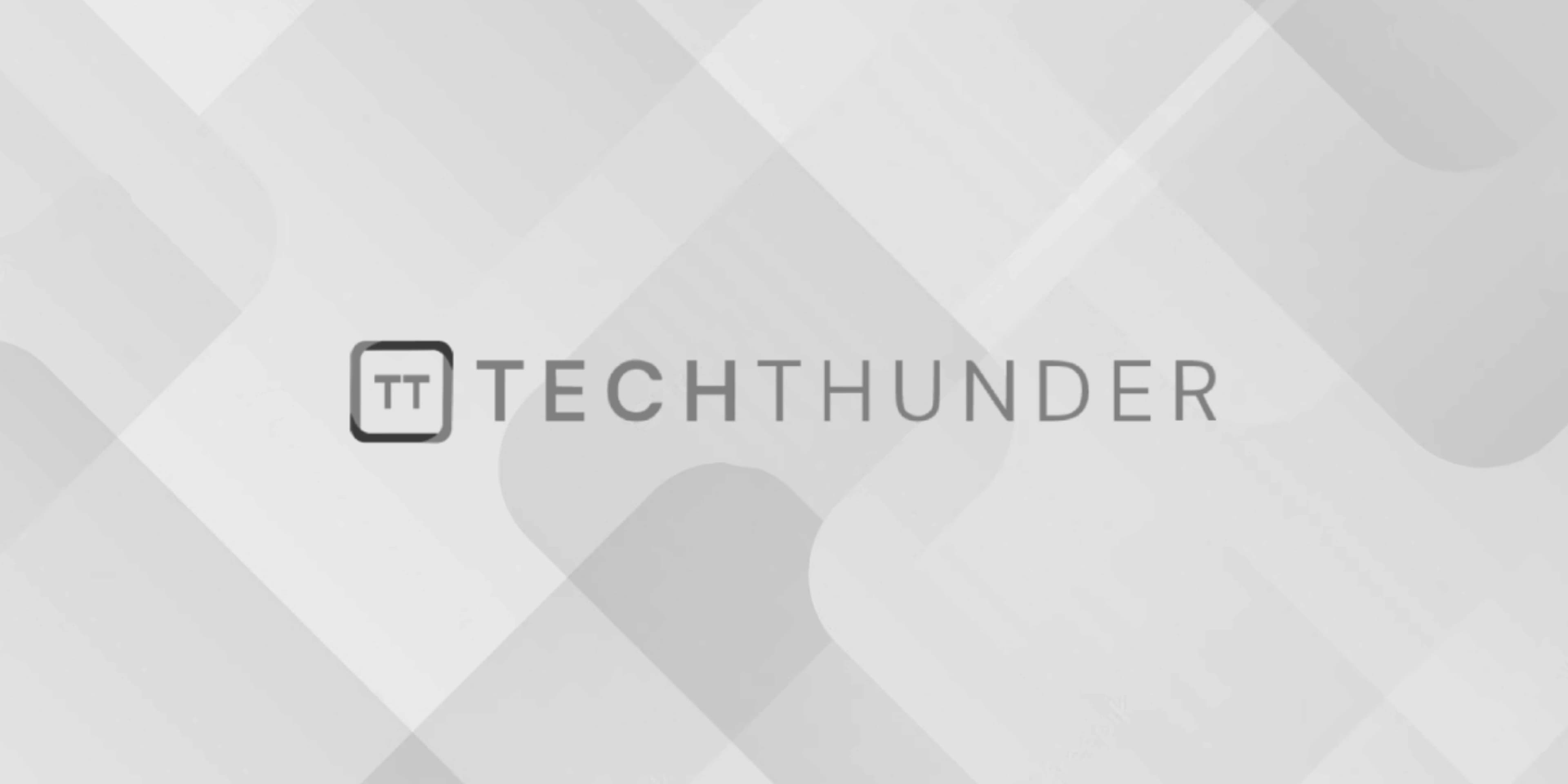
195 views
PHP STR_CONTAINS()
As of my last update in September 2021, there is no built-in str_contains()
function in PHP. However, PHP provides other functions that can be used to check if a string contains a specific substring. Some of these functions are:
strpos()
: Thestrpos()
function is used to find the position of the first occurrence of a substring in a string. It returns the position as an integer, orfalse
if the substring is not found.
$string = "Hello, world!";
$substring = "world";
if (strpos($string, $substring) !== false) {
echo "Substring found in the string.";
} else {
echo "Substring not found in the string.";
}
stripos()
: Thestripos()
function is similar tostrpos()
, but it performs a case-insensitive search.
$string = "Hello, World!";
$substring = "world";
if (stripos($string, $substring) !== false) {
echo "Substring found in the string (case-insensitive).";
} else {
echo "Substring not found in the string.";
}
strstr()
: Thestrstr()
function is used to find the first occurrence of a substring in a string and return the rest of the string from that position. It returnsfalse
if the substring is not found.
$string = "Hello, world!";
$substring = "world";
$result = strstr($string, $substring);
if ($result !== false) {
echo "Substring found in the string: " . $result;
} else {
echo "Substring not found in the string.";
}
stristr()
: Thestristr()
function is similar tostrstr()
, but it performs a case-insensitive search.
$string = "Hello, World!";
$substring = "world";
$result = stristr($string, $substring);
if ($result !== false) {
echo "Substring found in the string (case-insensitive): " . $result;
} else {
echo "Substring not found in the string.";
}
Keep in mind that the availability of functions may change in newer PHP versions, so always refer to the PHP manual for the specific version you are using. As of my last update, the above functions are available in PHP and can be used to check if a string contains a specific substring.