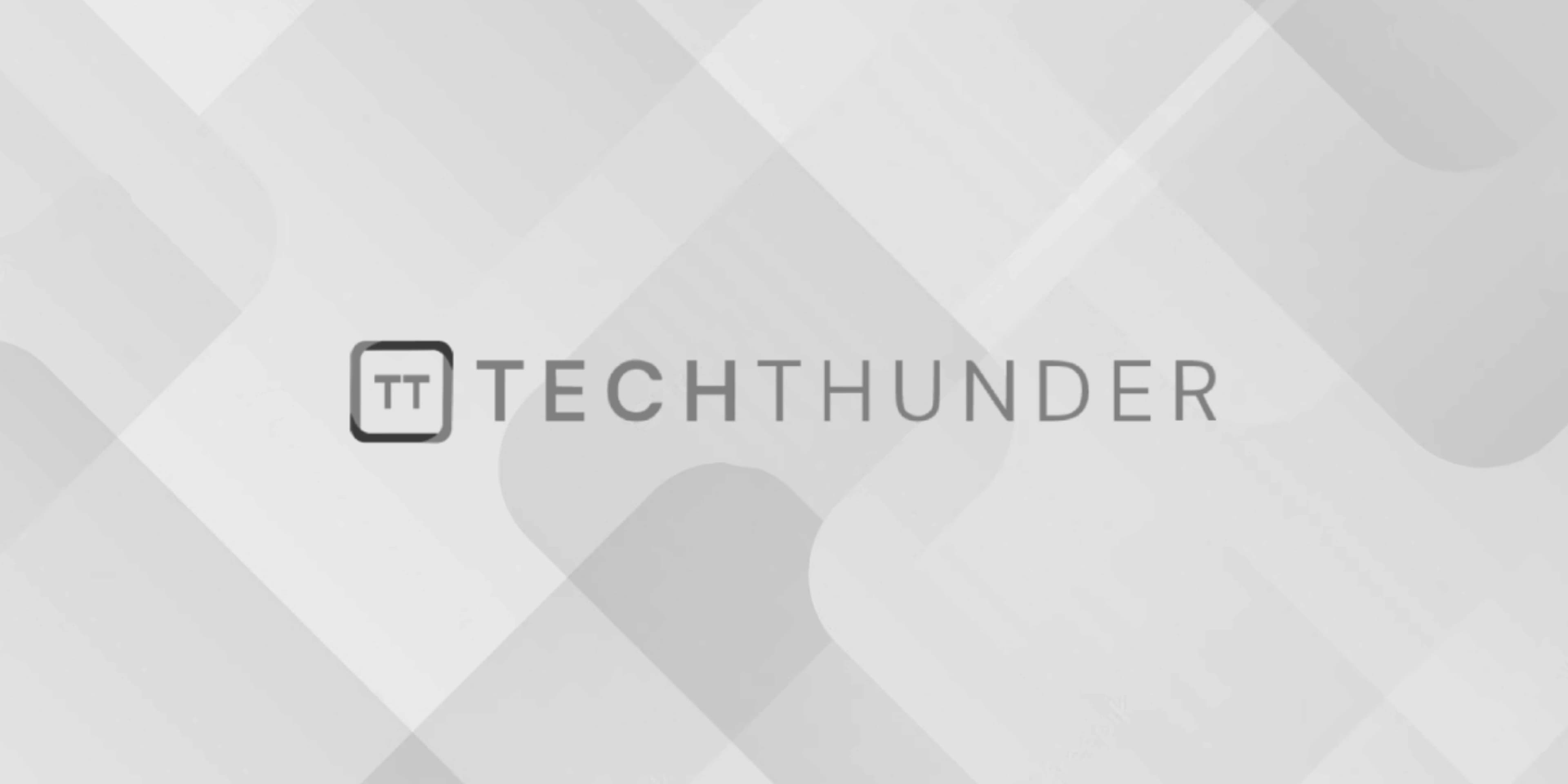
PHP header() function
In PHP, the header()
function is used to send raw HTTP header information to the client (the web browser) before any other output is sent. It allows you to set HTTP headers, such as content type, status codes, caching instructions, and redirection, which affect how the client interprets and handles the response from the server.
Syntax:
The basic syntax of the header()
function is as follows:
header(string $header, bool $replace = true, int $http_response_code = 0);
Parameters:
$header
: The HTTP header to send to the client in the format “HeaderName: HeaderValue”.$replace
: (Optional) A boolean flag indicating whether to replace an existing header with the same name. By default, it is set totrue
, which means the header will replace an existing header with the same name. If set tofalse
, it will not replace an existing header with the same name.$http_response_code
: (Optional) The HTTP response code to send with the header. This is often used when sending specific status codes, such as 404 for “Not Found” or 301 for “Moved Permanently.”
Example:
- Setting Content Type:
header("Content-Type: text/html");
- Redirecting to Another Page:
header("Location: https://www.example.com");
exit; // It is important to exit the script after sending a redirection header.
- Setting Custom HTTP Response Code:
header("HTTP/1.1 404 Not Found");
It is crucial to call the header()
function before any output is sent to the client. If you try to call header()
after there has been any output (e.g., HTML, text, or even white spaces), it will result in a warning, and the headers will not be set. In such cases, you can use the ob_start()
function to buffer the output and delay it until after the headers are set.
Example of using ob_start()
to set headers after output:
<?php
ob_start();
header("Content-Type: text/html");
// ... your PHP code ...
ob_end_flush(); // Send the buffered output after setting headers.
It is essential to use the header()
function carefully, especially when sending redirection headers. For proper redirection, it is recommended to include an exit;
statement after the header()
call to halt script execution immediately after the redirection header is sent. Otherwise, the script will continue executing, and the redirection may not work as expected.