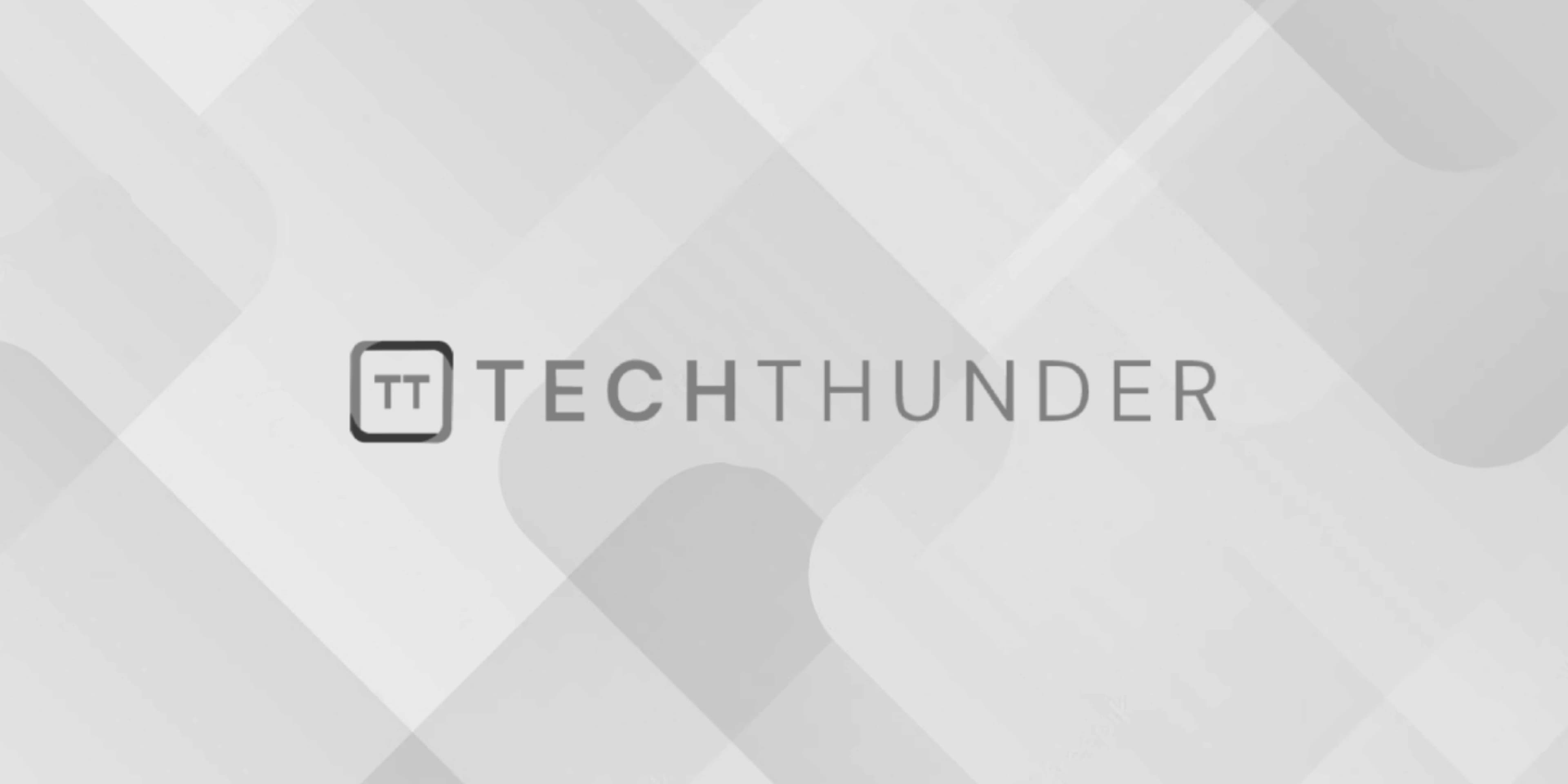
Imagick addImage() Function in PHP
In PHP, Imagick::addImage()
is a method of the Imagick class, which is part of the Imagick extension. The Imagick extension is a powerful PHP library that allows you to manipulate images using the ImageMagick software.
The addImage()
method is used to add another image to the current sequence of images stored in an Imagick object. It is typically used when working with animated images or creating image sequences.
Here’s the syntax of the addImage()
method:
public Imagick::addImage(Imagick $source)
Parameters:
$source
: An instance of the Imagick class representing the image that you want to add to the current image sequence.
Return value:
- This method does not have a return value.
Example:
Let’s see an example of how to use the addImage()
method to create an animated GIF from multiple image frames:
// Create a new Imagick object
$imagick = new Imagick();
// Load multiple image frames and add them to the Imagick object
$frame1 = new Imagick('frame1.png');
$frame2 = new Imagick('frame2.png');
$frame3 = new Imagick('frame3.png');
$imagick->addImage($frame1);
$imagick->addImage($frame2);
$imagick->addImage($frame3);
// Set the format of the output image to "gif"
$imagick->setFormat('gif');
// Combine the frames into an animated GIF and optimize it
$imagick = $imagick->deconstructImages();
$imagick->optimizeImageLayers();
// Save the final animated GIF to a file
$imagick->writeImages('animated.gif', true);
In this example, we create an Imagick object and load three image frames (frame1.png
, frame2.png
, and frame3.png
). We then add these frames to the Imagick object using the addImage()
method. After combining the frames, we set the format to GIF and optimize the layers to create an animated GIF. Finally, we save the resulting animated GIF to a file called animated.gif
.
Please note that to use the Imagick
class and its methods, you need to have the Imagick extension installed and enabled on your PHP server. Additionally, you should have the ImageMagick software installed on the server for the Imagick extension to work properly.