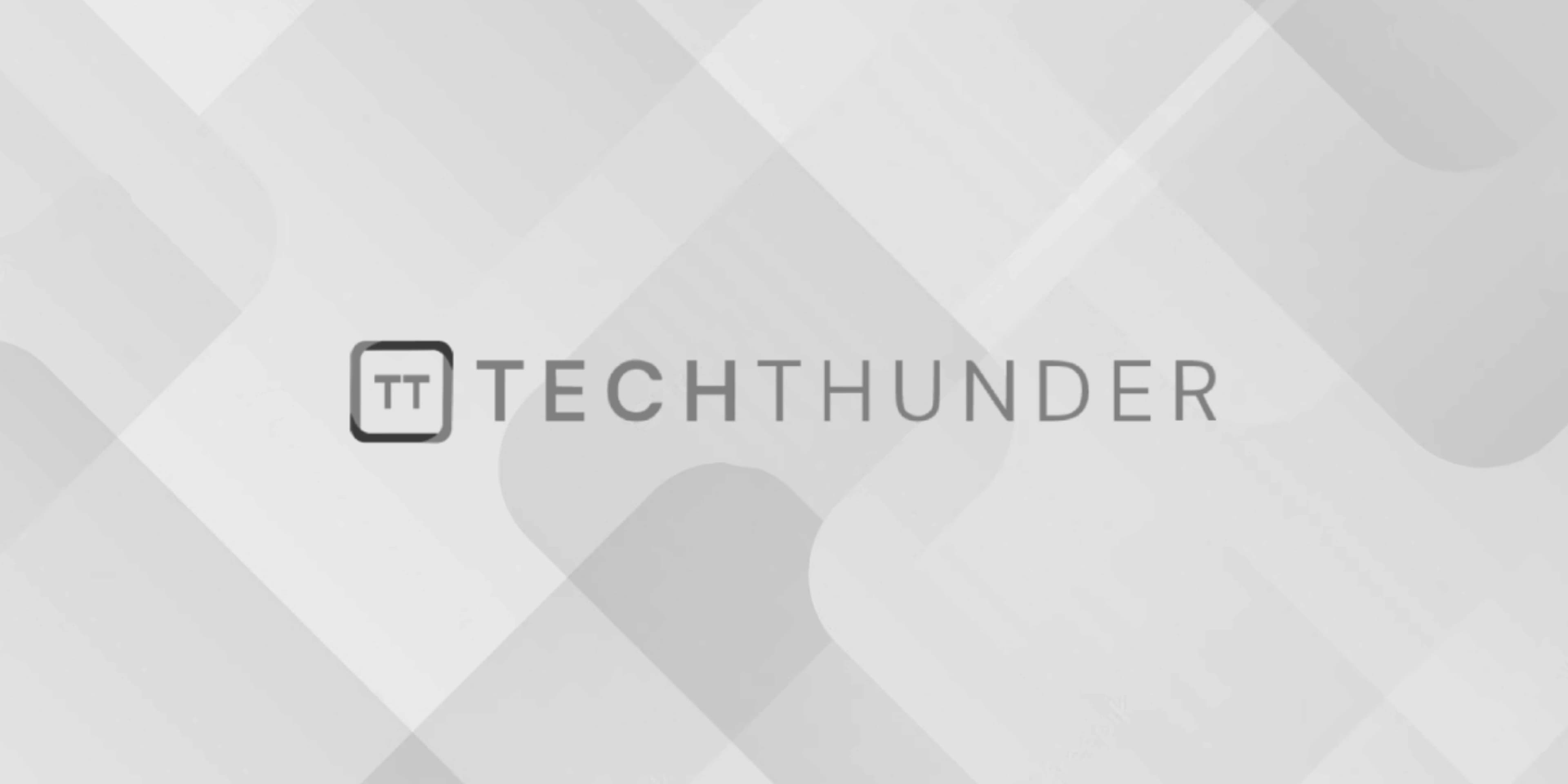
PHP Classes
In PHP, classes are the fundamental building blocks of object-oriented programming (OOP). A class is a blueprint or template that defines the structure and behavior of objects. Objects, in turn, are instances of a class, and they represent real-world entities with specific properties and functionalities.
To create a class in PHP, you use the class
keyword followed by the class name and a pair of curly braces to define the class body. The class body contains properties (also known as class variables) and methods (functions within the class).
Here’s the basic syntax of a PHP class:
class ClassName {
// Class properties (variables)
public $property1;
private $property2;
protected $property3;
// Class methods (functions)
public function method1() {
// Method code...
}
private function method2() {
// Method code...
}
protected function method3() {
// Method code...
}
}
Let’s explore some essential concepts related to PHP classes:
- Properties: Class properties are variables that hold data specific to each object created from the class. They can have different visibility levels, such as
public
,private
, orprotected
, which control their accessibility outside the class. - Methods: Class methods are functions that define the behavior of the class. They can interact with the class properties and perform specific actions.
- Constructors: The constructor is a special method with the name
__construct()
. It is automatically called when an object is created from the class and is used to initialize the object’s properties. - Visibility: As mentioned earlier, class properties and methods can have different visibility levels.
public
properties and methods can be accessed from anywhere,private
properties and methods are accessible only within the class itself, andprotected
properties and methods are accessible within the class and its subclasses. - Inheritance: PHP supports class inheritance, allowing you to create a new class (called a subclass or child class) based on an existing class (called a superclass or parent class). The subclass inherits properties and methods from the parent class and can also define its own unique properties and methods.
- Object Instantiation: To create an object from a class, you use the
new
keyword followed by the class name and parentheses. The constructor is called automatically when the object is created.
Example of creating and using a PHP class:
class Car {
public $brand;
public $model;
public function __construct($brand, $model) {
$this->brand = $brand;
$this->model = $model;
}
public function startEngine() {
return "Starting the engine of $this->brand $this->model.";
}
}
// Create an object of the Car class
$myCar = new Car('Toyota', 'Camry');
// Call the method of the object
echo $myCar->startEngine(); // Output: Starting the engine of Toyota Camry.
In this example, we define a Car
class with properties brand
and model
, and a constructor to initialize these properties. We also have a method startEngine()
that returns a message about starting the engine. We then create an object $myCar
from the class and call its startEngine()
method.
Classes play a central role in object-oriented programming, allowing developers to organize and structure code in a modular and reusable manner, promoting code encapsulation and separation of concerns.