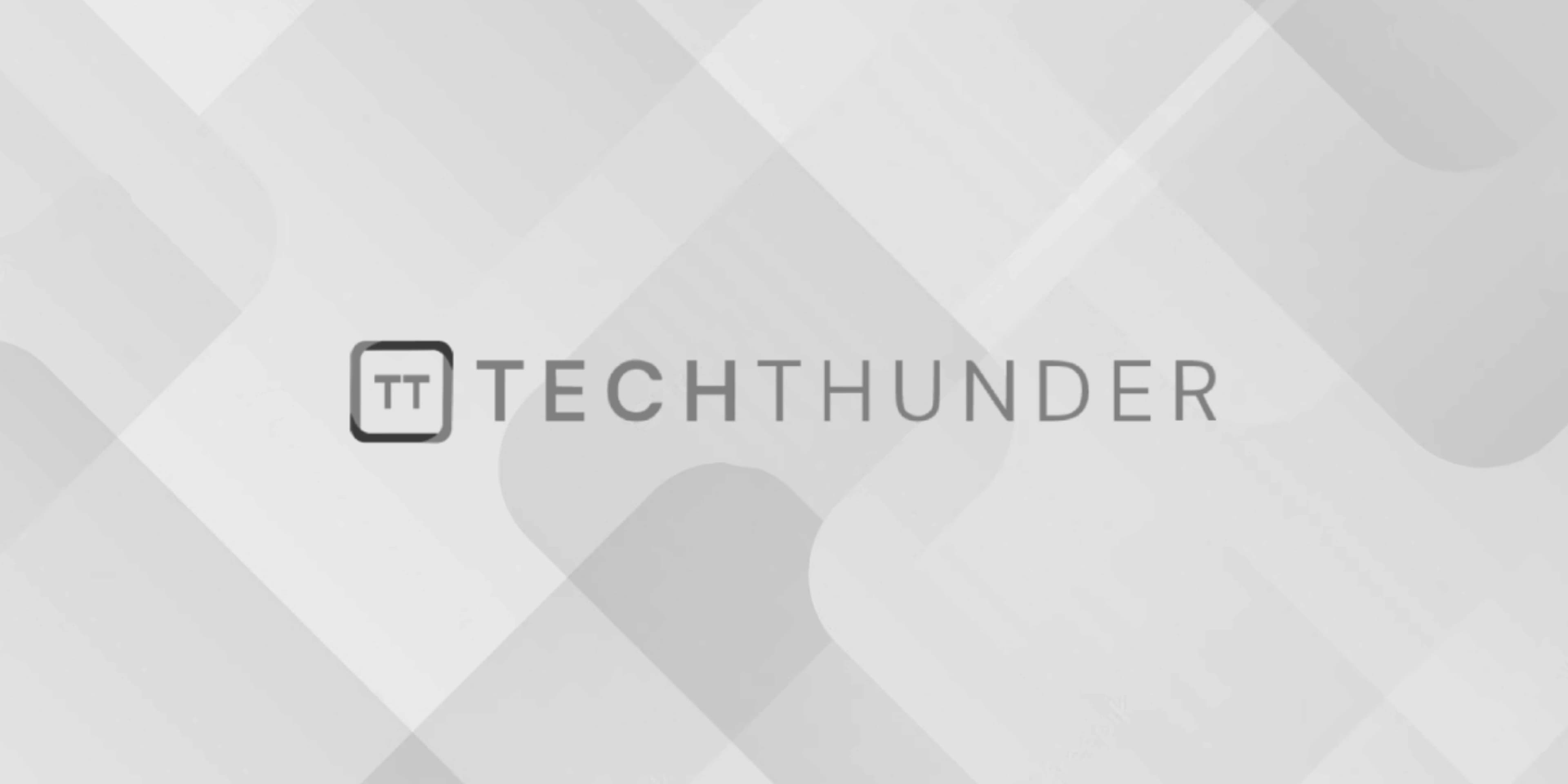
PHP Echo
In PHP, echo
is a language construct used to output data to the browser or the standard output. It allows you to display text, HTML, or the values of variables.
The basic syntax of echo
is:
echo expression;
Here, the expression
can be a string (enclosed in quotes) or a variable containing the value you want to display.
Examples:
- Displaying a String:
<?php
echo "Hello, World!";
?>
Output: Hello, World!
- Displaying Variables:
<?php
$name = "John";
$age = 30;
echo "My name is " . $name . " and I am " . $age . " years old.";
?>
Output: My name is John and I am 30 years old.
- Displaying HTML:
<?php
echo "<h1>Welcome to My Website</h1>";
echo "<p>This is a paragraph of text.</p>";
?>
Output:
Welcome to My Website
This is a paragraph of text.
- Displaying the Result of an Expression:
<?php
$number1 = 10;
$number2 = 5;
$result = $number1 + $number2;
echo "The sum of $number1 and $number2 is: " . $result;
?>
Output: The sum of 10 and 5 is: 15
Note: You can use double quotes or single quotes to define strings in PHP. Using double quotes allows you to include variables within the string (variable interpolation), while single quotes treat everything as a literal string. For example:
$name = "John";
echo "My name is $name."; // Output: My name is John.
echo 'My name is $name.'; // Output: My name is $name.
Remember to use semicolons at the end of each echo
statement to terminate the PHP statement properly.