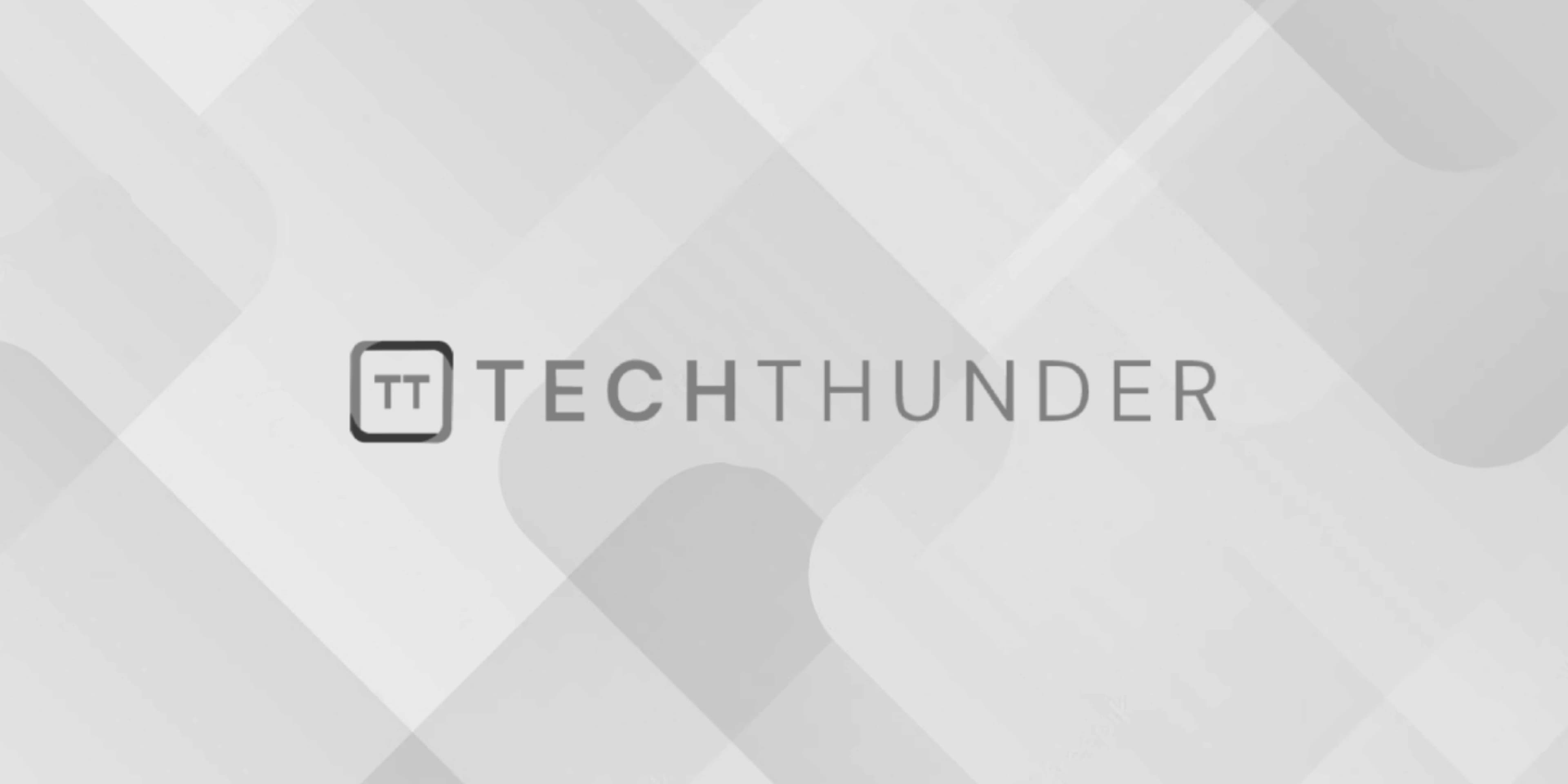
222 views
How to Convert array into string in PHP
In PHP, you can convert an array into a string using various functions and methods based on your specific requirements. Here are some common ways to convert an array into a string:
- Using implode() function:
Theimplode()
function (also known asjoin()
) takes an array and concatenates its elements into a single string using a specified delimiter.
$array = array('apple', 'banana', 'orange');
$string = implode(', ', $array);
echo $string; // Output: "apple, banana, orange"
- Using join() function:
Thejoin()
function is an alias ofimplode()
, and you can use it in the same way.
$array = array('apple', 'banana', 'orange');
$string = join(', ', $array);
echo $string; // Output: "apple, banana, orange"
- Using the concatenation operator (.)
You can manually loop through the array and concatenate its elements into a string using the dot (.) operator.
$array = array('apple', 'banana', 'orange');
$string = '';
foreach ($array as $value) {
$string .= $value . ', ';
}
// Remove the trailing comma and space
$string = rtrim($string, ', ');
echo $string; // Output: "apple, banana, orange"
- Using array_reduce() function:
Thearray_reduce()
function allows you to apply a callback function to an array and return a single value. You can use it to concatenate the array elements into a string.
$array = array('apple', 'banana', 'orange');
$string = array_reduce($array, function ($carry, $item) {
return $carry . $item . ', ';
}, '');
// Remove the trailing comma and space
$string = rtrim($string, ', ');
echo $string; // Output: "apple, banana, orange"
- Using json_encode() function:
If you want to convert the entire array into a JSON string, you can use thejson_encode()
function.
$array = array('apple', 'banana', 'orange');
$string = json_encode($array);
echo $string; // Output: '["apple","banana","orange"]'
The method you choose depends on how you want the array elements to be represented in the string and what format you need for further processing. For most cases, implode()
or join()
is the preferred method for converting an array into a string with custom delimiters.