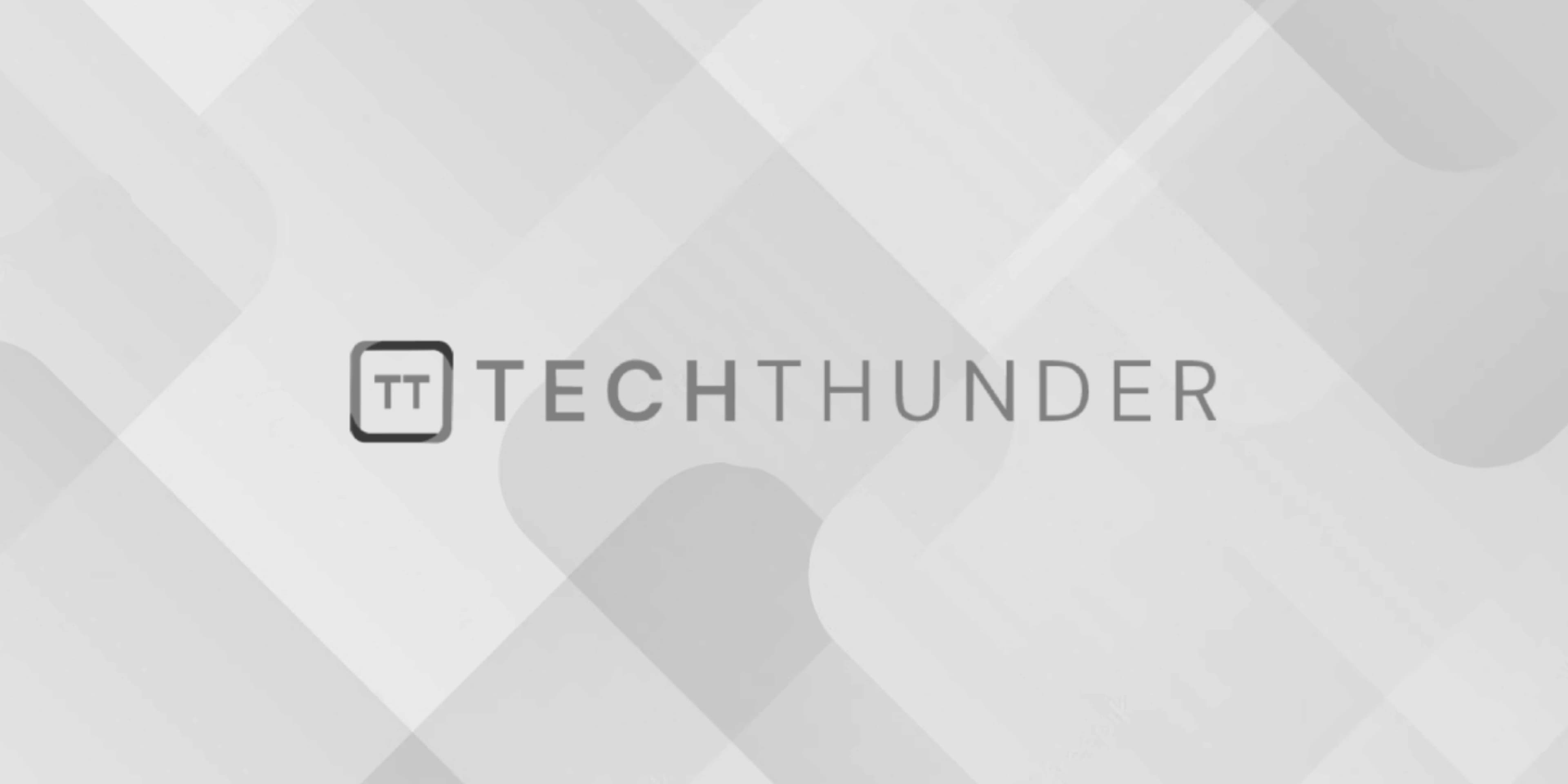
PHP Type Casting and Conversion of an Object to an Object of other class
In PHP, type casting and conversion refer to the process of changing the data type of a variable or converting an object from one class to an object of another class. PHP provides several methods to perform type casting and conversion, including explicit type casting and object conversion through constructors.
- Explicit Type Casting:
PHP allows you to explicitly cast variables from one data type to another using casting operators. The basic casting operators in PHP are:
(int)
or(integer)
: Converts a value to an integer.(float)
or(double)
: Converts a value to a floating-point number (float/double).(string)
: Converts a value to a string.(bool)
or(boolean)
: Converts a value to a boolean (true/false).(array)
: Converts a value to an array.(object)
: Converts a value to an object.
Example of explicit type casting:
$intValue = 42;
$floatValue = (float) $intValue;
$stringValue = (string) $intValue;
$boolValue = (bool) $intValue;
- Object Conversion:
To convert an object from one class to an object of another class, you typically use constructors or create custom conversion methods. Constructors are special methods that are automatically called when an object is created from a class. You can define custom logic in constructors to convert an object from one type to another.
Example of object conversion using constructors:
class Person {
private $name;
private $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
}
class Employee {
private $name;
private $position;
public function __construct($name, $position) {
$this->name = $name;
$this->position = $position;
}
}
// Creating a Person object
$person = new Person('John Doe', 30);
// Converting Person object to Employee object using constructor
$employee = new Employee($person->name, 'Manager');
In this example, we have two classes: Person
and Employee
. We create a Person
object and then use the data from that object to create an Employee
object by passing the relevant information to the Employee
constructor.
Keep in mind that converting objects between unrelated classes might not always be straightforward, and it might require additional logic or data mapping to ensure the conversion is meaningful and correct.
It’s essential to use type casting and object conversion carefully to avoid unexpected results and maintain the integrity of your data and application logic.