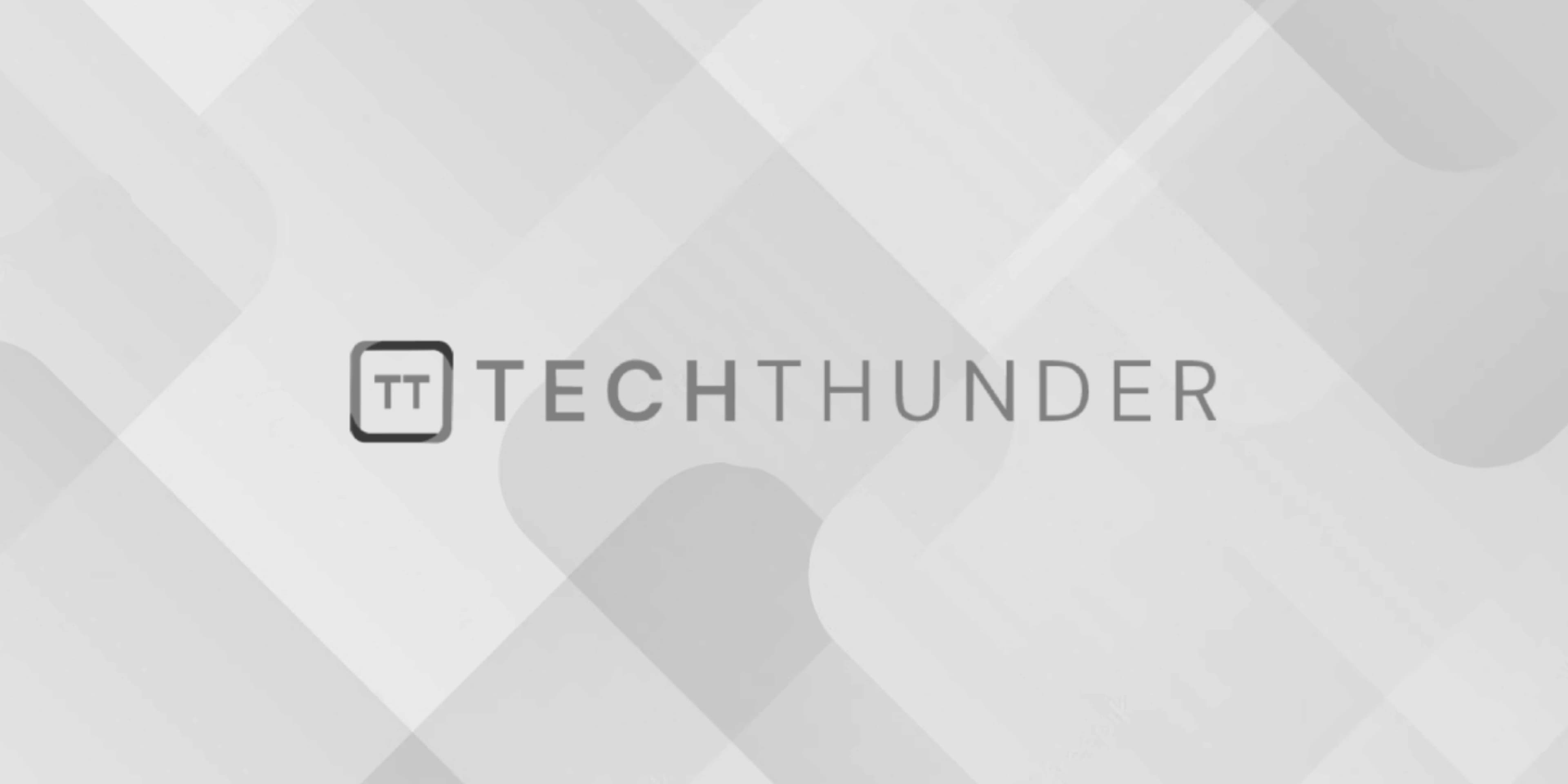
Imagick floodFillPaintImage() Function in PHP
In PHP, Imagick::floodFillPaintImage()
is a method of the Imagick class, which is part of the Imagick extension. The Imagick extension is a powerful PHP library that allows you to manipulate images using the ImageMagick software.
The floodFillPaintImage()
method is used to replace a particular color in an image with another color using a flood-fill algorithm. The flood-fill algorithm starts from a specified seed point (coordinates) and replaces all connected pixels of the same color with the new color.
Here’s the syntax of the floodFillPaintImage()
method:
public Imagick::floodFillPaintImage(mixed $fill, float $fuzz, mixed $target, int $x, int $y[, int $channel = Imagick::CHANNEL_DEFAULT])
Parameters:
$fill
: The color that will be used for replacing the target color. It can be specified as an ImagickPixel object or a color string (e.g., “red”, “#FF0000”).$fuzz
: A float value (0 to 1) that specifies the range of color variation allowed. The larger the$fuzz
, the broader the range of colors that will be replaced. For example, a$fuzz
value of 0.1 will match colors within 10% difference.$target
: The color to be replaced. It can be specified as an ImagickPixel object or a color string.$x
: The x-coordinate (horizontal position) of the seed point (starting point) for the flood-fill.$y
: The y-coordinate (vertical position) of the seed point (starting point) for the flood-fill.$channel
: (Optional) The channel to apply the flood-fill operation. It is represented by one of theImagick::CHANNEL_*
constants. By default,Imagick::CHANNEL_DEFAULT
is used, which applies the operation to all channels.
Return value:
- The method returns
true
on success.
Example:
Let’s see an example of how to use the floodFillPaintImage()
method to replace a color in an image:
// Create a new Imagick object and read an image file
$imagick = new Imagick('input.jpg');
// Specify the target color to be replaced (red in this example)
$targetColor = 'red';
// Specify the fill color (blue in this example)
$fillColor = 'blue';
// Specify the coordinates (seed point) to start the flood-fill
$seedX = 100;
$seedY = 100;
// Set the fuzz value (color variation allowed)
$fuzz = 0.1;
// Replace the target color with the fill color using flood-fill
$imagick->floodFillPaintImage($fillColor, $fuzz, $targetColor, $seedX, $seedY);
// Set the output image format to JPEG
$imagick->setImageFormat('jpeg');
// Output the modified image to the browser
header('Content-Type: image/jpeg');
echo $imagick;
In this example, we create an Imagick object and load an image file named input.jpg
. We then specify the target color to be replaced (red) and the fill color to use (blue). We set the seed point (starting point) for the flood-fill at coordinates (100, 100). Finally, we apply the flood-fill operation using the floodFillPaintImage()
method and output the modified image to the browser.
Please note that to use the Imagick
class and its methods, you need to have the Imagick extension installed and enabled on your PHP server. Additionally, you should have the ImageMagick software installed on the server for the Imagick extension to work properly.