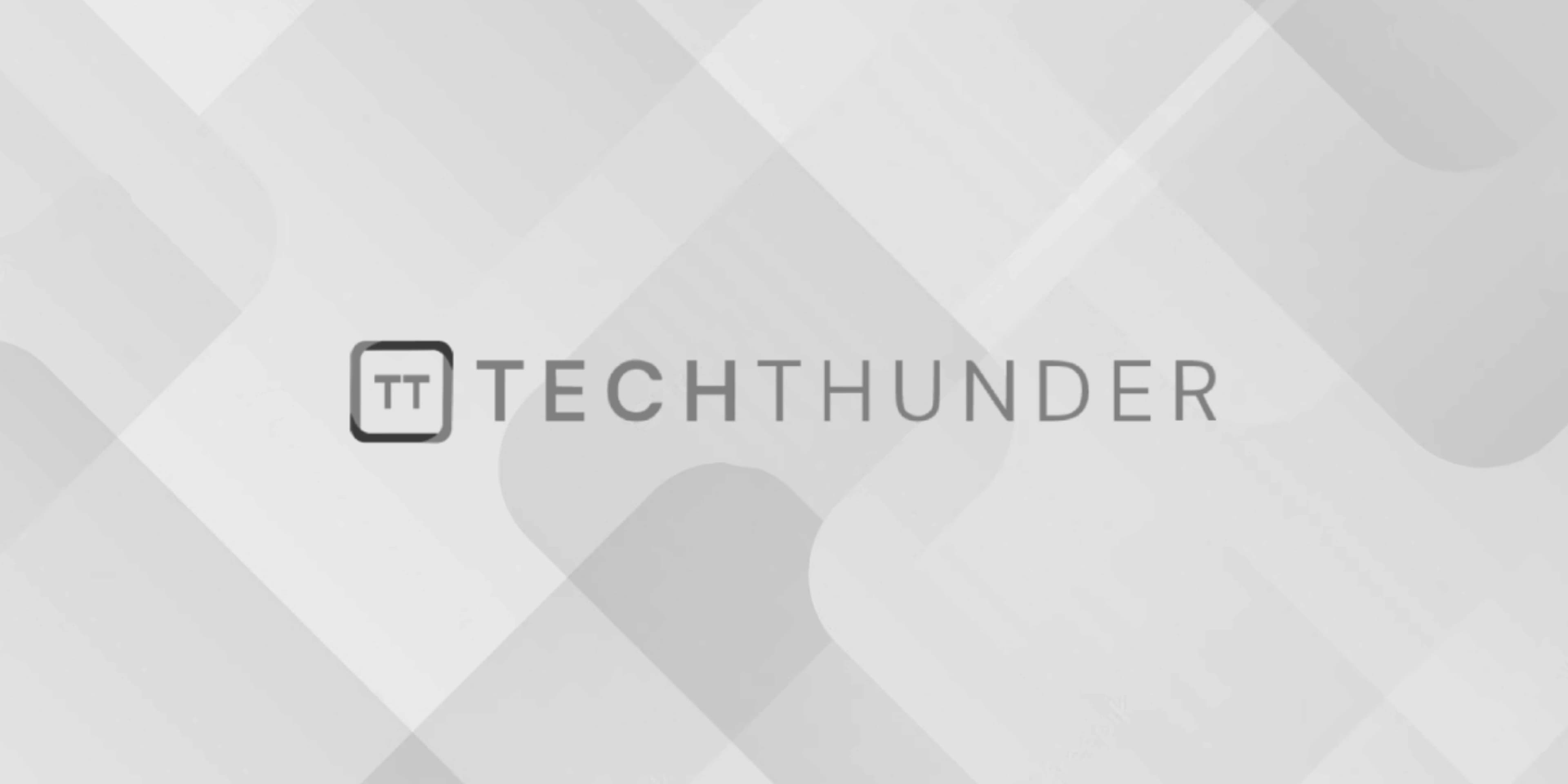
PHP MySQLi CREATE DB
In PHP, you can use MySQLi (MySQL Improved) to create a new MySQL database using the mysqli_query()
function to execute SQL queries. Before creating a database, you need to establish a connection to the MySQL server using mysqli_connect()
. Here’s a step-by-step guide on how to create a new MySQL database using MySQLi in PHP:
<?php
$servername = "localhost"; // Replace with your server name or IP address
$username = "your_username"; // Replace with your MySQL username
$password = "your_password"; // Replace with your MySQL password
// Create a connection
$conn = mysqli_connect($servername, $username, $password);
// Check the connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// Create a new database
$databaseName = "your_new_database"; // Replace with the name you want for your new database
$sql = "CREATE DATABASE $databaseName";
if (mysqli_query($conn, $sql)) {
echo "Database created successfully.";
} else {
echo "Error creating database: " . mysqli_error($conn);
}
// Close the connection
mysqli_close($conn);
?>
In this example, we first create a connection to the MySQL server using mysqli_connect()
and then check if the connection is successful. Next, we define the name of the new database in the $databaseName
variable.
The SQL query to create the new database is constructed in the $sql
variable. The CREATE DATABASE
statement is used to create a new database in MySQL.
We use the mysqli_query()
function to execute the SQL query. If the database creation is successful, it will print “Database created successfully.” Otherwise, it will display an error message.
Finally, we close the database connection using mysqli_close()
.
Remember to replace "your_username"
, "your_password"
, and "your_new_database"
with your actual MySQL username, password, and the desired name for the new database. Also, make sure that the MySQL user you are using has the necessary privileges to create a new database on the server.
Please exercise caution while running this script, as it has the potential to create a new database on your MySQL server. Always double-check your credentials and ensure that you are authorized to perform this operation.