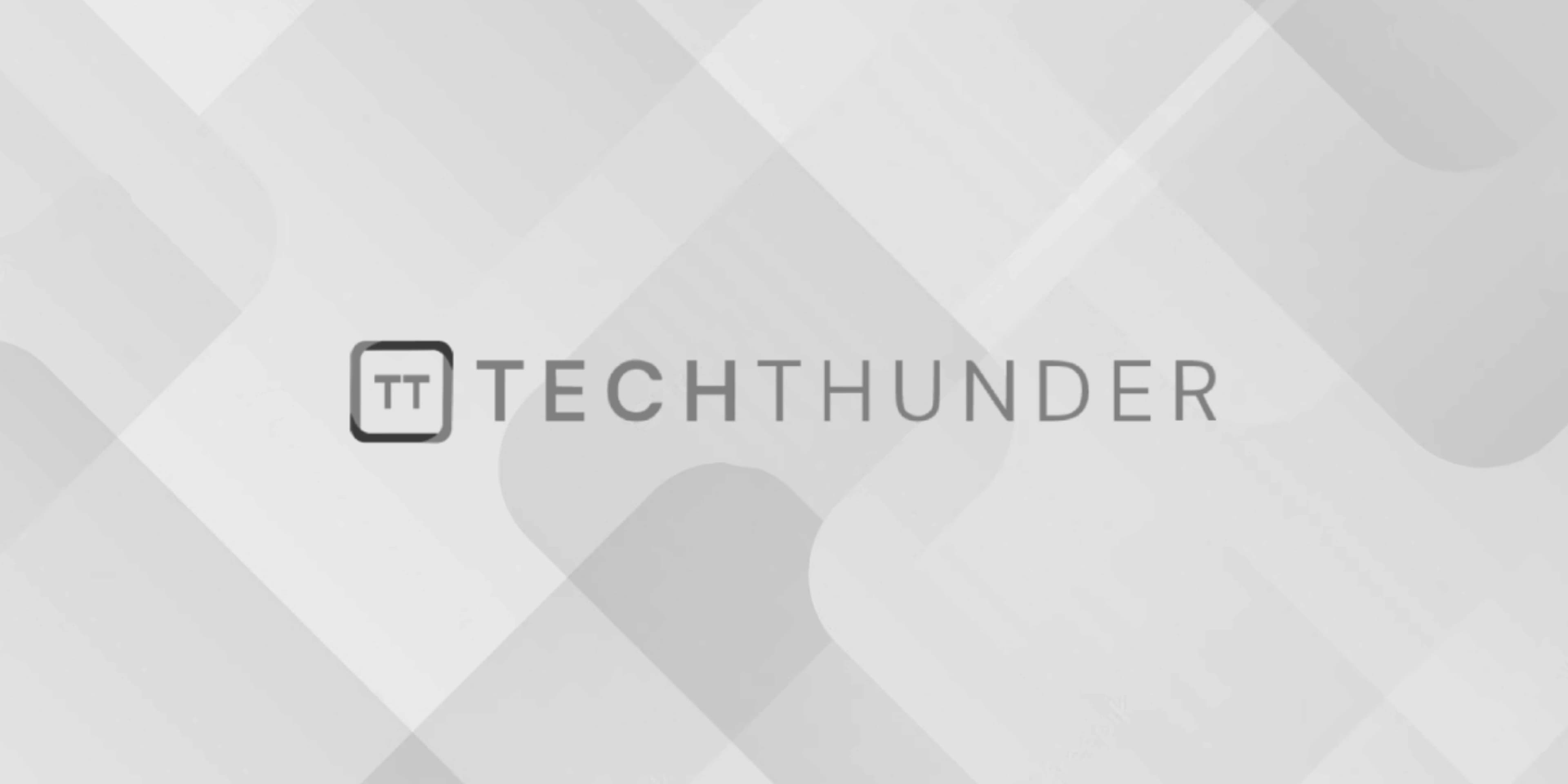
OOPs Final Keyword
In object-oriented programming (OOP), the final
keyword is used to indicate that a class, method, or property cannot be extended, overridden, or redefined by any subclass. It prevents inheritance and ensures that the final element remains unchanged in its derived classes.
- Final Class:
When a class is marked asfinal
, it cannot be subclassed, meaning no other class can inherit from it.
final class MyFinalClass {
// Class members and methods...
}
- Final Method:
When a method is marked asfinal
, it cannot be overridden by any subclass, even if the subclass has a method with the same name and signature.
class ParentClass {
final public function finalMethod() {
// Method implementation...
}
}
class ChildClass extends ParentClass {
// Attempting to override the final method will result in an error.
// The finalMethod() in the ParentClass cannot be redefined here.
}
- Final Property (as of PHP 8.0):
In PHP 8.0 and later, properties can also be marked asfinal
. When a property is declared asfinal
, it cannot be redeclared in any subclass.
class ParentClass {
final public $finalProperty = "This property cannot be changed.";
}
class ChildClass extends ParentClass {
// Attempting to redeclare the final property will result in an error.
// The $finalProperty in the ParentClass cannot be redeclared here.
}
Using the final
keyword is helpful when you want to prevent further modifications or extensions of specific classes, methods, or properties to maintain the intended behavior of your code and avoid unintended consequences. It can be particularly useful for critical components of a class or framework, where changes could have significant impacts on the system.
However, it’s important to use the final
keyword judiciously. Overusing it may limit the flexibility and extensibility of your code, making it harder to maintain and adapt in the future. It’s best to apply it only to elements that you genuinely want to be immutable and not subject to changes or extension in derived classes.