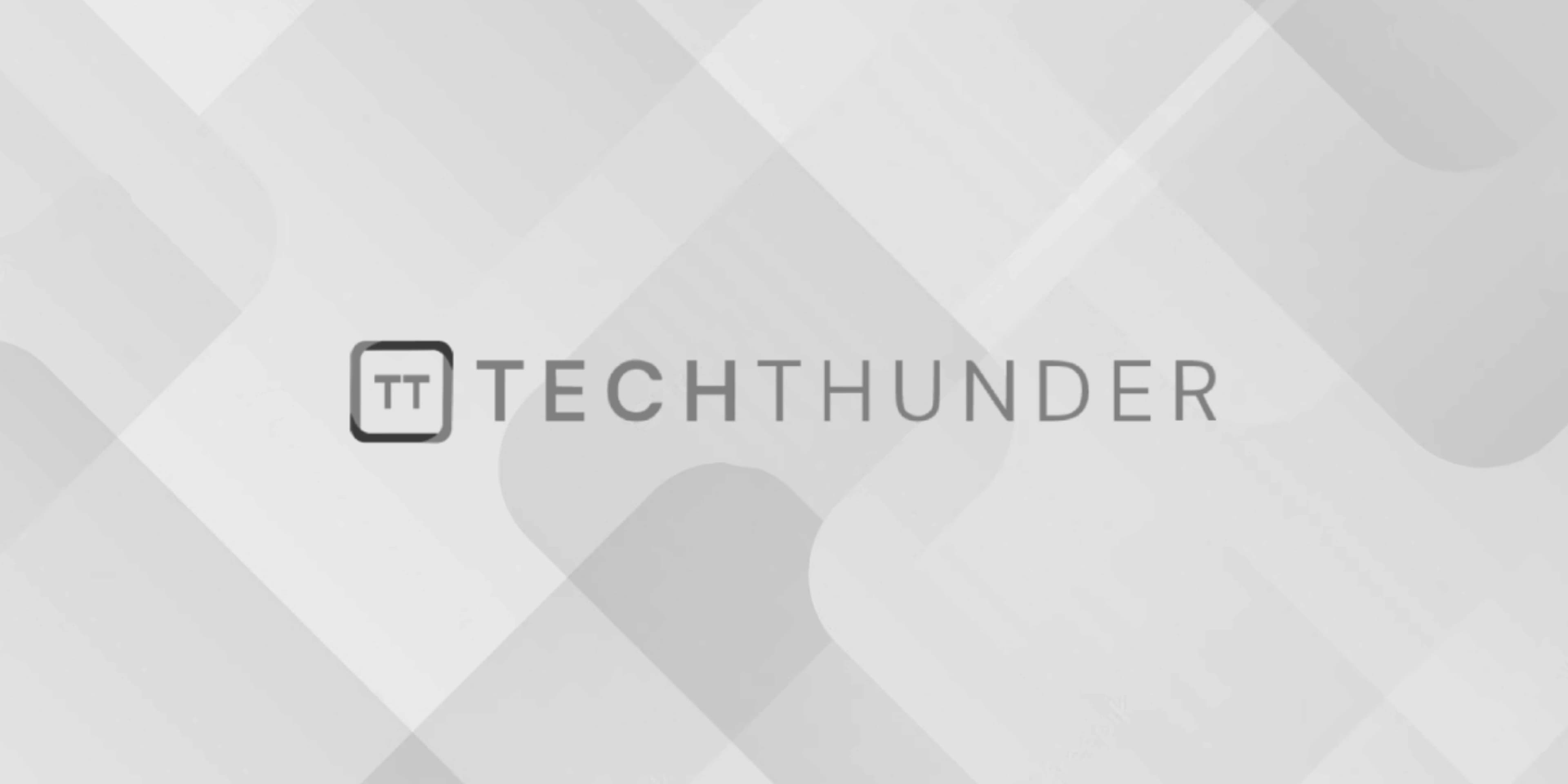
190 views
OOPs Access Specifiers
In object-oriented programming (OOP), access specifiers are keywords used to define the visibility or accessibility of class properties and methods. Access specifiers control how different parts of a class can be accessed or modified from outside the class or its subclasses. In most object-oriented languages, including PHP, there are three main access specifiers:
- Public: The
public
access specifier allows class properties and methods to be accessed from anywhere, both within the class and from outside the class.
Example:
class MyClass {
public $publicProperty;
public function publicMethod() {
echo "This is a public method.\n";
}
}
$obj = new MyClass();
$obj->publicProperty = "Hello, World!";
$obj->publicMethod(); // Output: This is a public method.
echo $obj->publicProperty; // Output: Hello, World!
- Protected: The
protected
access specifier restricts class properties and methods to be accessed only within the class and its subclasses. It prevents direct access from outside the class.
Example:
class MyClass {
protected $protectedProperty;
protected function protectedMethod() {
echo "This is a protected method.\n";
}
}
class SubClass extends MyClass {
public function accessProtected() {
$this->protectedProperty = "Hello, OOP!";
$this->protectedMethod();
}
}
$subObj = new SubClass();
$subObj->accessProtected(); // Output: This is a protected method.
- Private: The
private
access specifier restricts class properties and methods to be accessed only within the class. They cannot be accessed from outside the class or its subclasses.
Example:
class MyClass {
private $privateProperty;
private function privateMethod() {
echo "This is a private method.\n";
}
public function accessPrivate() {
$this->privateProperty = "Hello, Private!";
$this->privateMethod();
}
}
$obj = new MyClass();
$obj->accessPrivate(); // Output: This is a private method.
It’s important to use access specifiers wisely to encapsulate the internal details of a class and to control the access to its properties and methods. By using proper access specifiers, you can ensure that the class is properly encapsulated, providing better data protection and reducing the potential for unintended side effects in your code.